Beginner Level
Intermediate Level
Advanced Level
Introduction
Generators are Python functions that return an iterable generator object, which can be looped over like a list or a tuple. One of the key ingredients in creating generator functions is the Yield statement. Yield is a Python keyword that allows you to pause the execution of your function and return a value to the caller. In this tutorial, we'll cover everything you need to know about the Yield statement, its syntax, and its usage in building and working with generators. So, let's dive in!
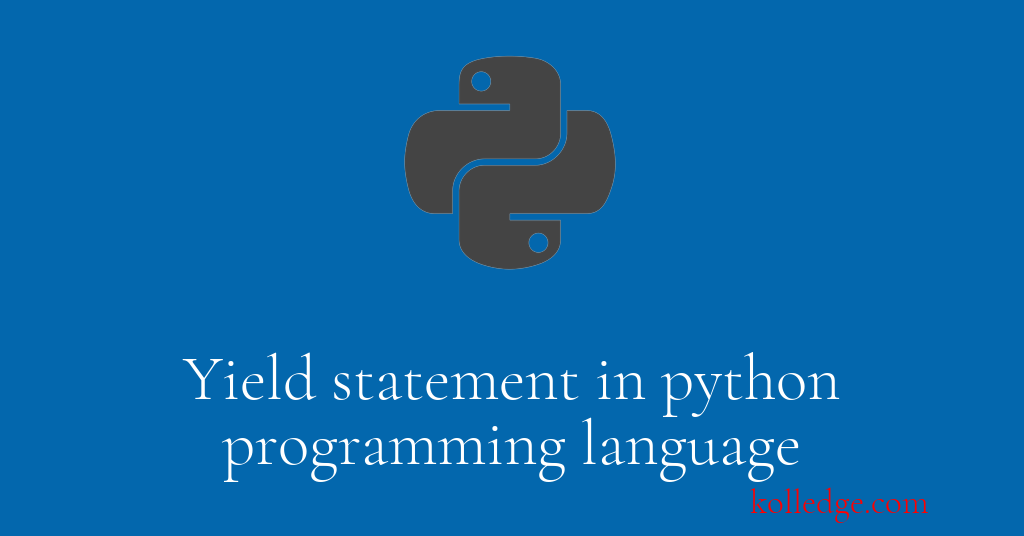
Table of Contents :
- What is Yield statement in Python
- Using Yield to Call Functions
- Using Yield Statement
- Yield vs. Return in Python
- Pros and Cons of Yield
What is Yield statement in Python:
- Yield is a keyword used in Python to define a generator function.
- A generator function is a function that returns an iterator.
- Yield allows us to create iterators in a simple, efficient, and clean way.
- When a yield statement is encountered in a function, the function becomes a generator function.
- The yield statement is used to pause the execution of the generator function and return a value.
Code sample of Using Yield in Python:
def countdown(num):
while num > 0:
yield num
num -= 1
for i in countdown(5):
print(i)
Using Yield to Call Functions :
- To call a function that uses the yield statement, we use the
next()
built-in function. - Each time the
next()
function is called, the generator function resumes execution until it reaches the next yield statement. - The result of the yield statement is returned by the
next()
function. - Code Sample :
def countdown(num):
while num > 0:
yield num
num -= 1
c = countdown(5)
print(next(c))
print(next(c))
print(next(c))
Using Yield Statement:
- Yield is useful for generating a sequence of values that can be iterated over, without having to generate all the values at once.
- It is useful for memory-efficient traversal of large data sets.
- Yield can be used to implement lazy evaluation of objects.
Yield vs. Return in Python:
- Return sends a specified value back to the caller and terminates the function.
- Yield sends a value back to the caller, but retains the state of the function, so it can resume execution later.
- A function that uses return can only be called once, while a function that uses yield can be called multiple times.
Pros and Cons of Yield:
Pros :
- Yield is easy to implement and can be used to create generators with very little code.
- Yield allows efficient and memory-friendly iteration over large data sets.
- Yield allows you to implement lazy evaluation of objects.
Cons :
- Yield can make code more complex and difficult to understand.
- Yield is not always the most efficient way to generate a sequence of values.
- Yield requires additional overhead to maintain the state of the function between calls.
Prev. Tutorial : Generator expressions
Next Tutorial : functions as objects