Beginner Level
Intermediate Level
Advanced Level
Introduction
Iterables are an essential concept in Python programming. They are objects that can be iterated over, meaning we can retrieve their elements one by one or in groups. These objects can be lists, tuples, dictionaries, sets, and even strings. Understanding iterables is crucial for becoming skilled at programming in Python because they enable us to perform tasks such as looping through data, filtering elements, or transforming data. In this tutorial, we will delve into what iterables are, how they work, and how to use them effectively to make your Python programs more efficient and concise.
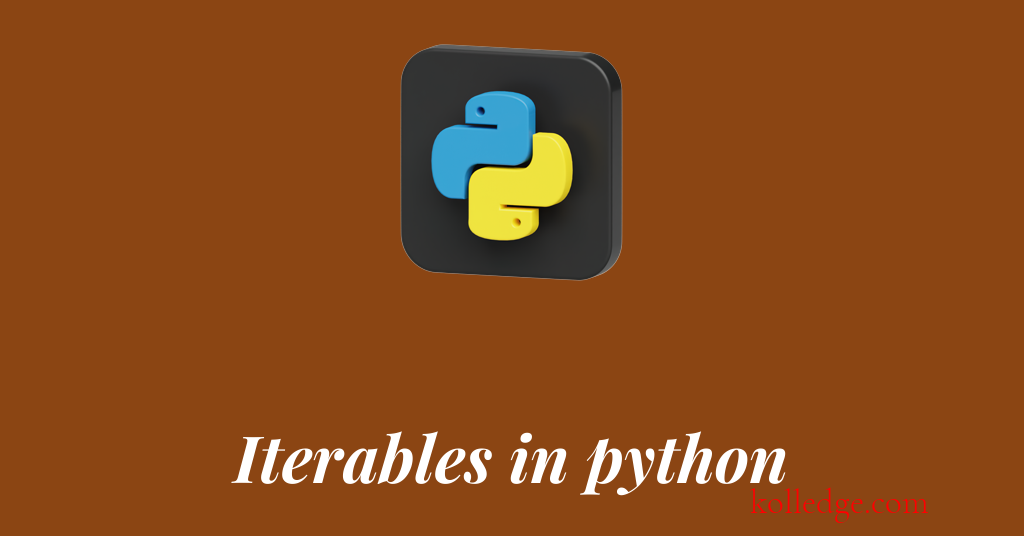
Table of Contents :
- Iterables in Python
- Iterator in Python
Iterables in Python :
- An iterable is an object in Python that can be iterated upon.
- We can use any python loop to iterate an iterable object in Python.
- In simple words - If we can loop over something, its an iterable in python.
- An iterable can have zero, one or more than one elements.
- An iterable object can return its element one at a time.
- Few example of iterable objects in Python are :
- Lists and tuples are iterables as we can iterate over its elements.
- A string is an iterable because we can iterate over its characters one at a time.
- The result of a range() function is an iterable.
Iterator in Python :
- We have just read that an iterable is an object that can be iterated over.
- An iterator is the object that is used to perform these iterations.
- We can use the python's built-in function
iter()
to get an iterator for an iterable object. - The iterable object is passed as an argument to the
iter()
function and it returns the iterator object for that iterable. - The
next()
method can be used on the iterator object to get the next element of the iterable. - The next element of the iterator is returned every time we call the
next()
method. - If there are no more elements left in the iterator, calling the
next()
method raises an exception. - Once we have iterated an element, it is removed from the iterator.
- i.e. once we have completed a full loop cycle over an iterator, the iterator becomes empty.
- The iterator object is also an iterable, since we can iterate over an iterator object.
- If we call an
iter()
function for an iterator, it returns the same iterator object back. - Our tutorial on iterators vs iterables explains this topic in depth with the help of code samples.
Prev. Tutorial : Tuples
Next Tutorial : Map() function