Beginner Level
Intermediate Level
Advanced Level
Introduction
An exception is an error that occurs during the execution of a program, and Python provides several built-in exceptions that can be used to handle such errors. However, sometimes developers encounter situations where they need to raise their own custom exceptions to suit their specific needs. In this tutorial, we will explore how to create and raise custom exceptions in Python programming language, and how to handle them gracefully, in order to write better and more robust code.
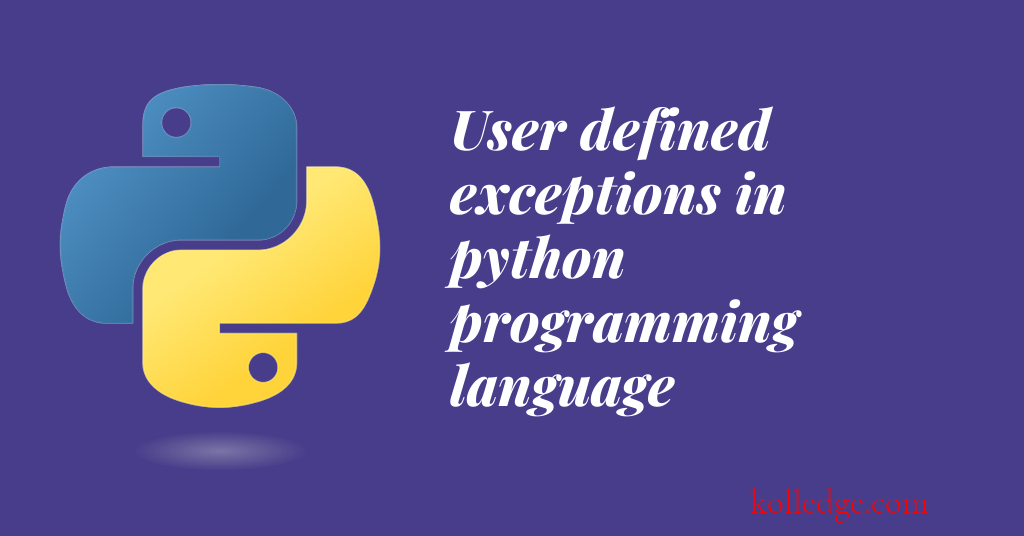
Table of Contents :
- Exception classes in Python
- User defined Exceptions
- Creating a user defined exception
- Raising a user-defined exception
- Catching a user-defined exception
- Code Sample for user defined exception
- Catching multiple user-defined exception
- Getting type of user-defined exception
Exception classes in Python :
- In the previous tutorials we have learned how python handles exceptions.
- Python uses exception classes to handle specific types of exceptions.
- Exception classes can be of two types :
- Built-in
- User defined
- We have already read about built-in exceptions in python.
- One way of exception handling in python is by using user-defined exceptions.
User defined Exceptions
Creating a user defined exception
- User-defined exception classes are created by inheriting from the
Exception
class. - Basic syntax of creating user-defined exceptions is :
class MyError(Exception):
pass
# This creates a new exception class called MyError.
Raising a user-defined exception :
- To raise an user-defined exception, we can use the raise keyword as normal .
- Basic syntax for raising user-defined exceptions :
raise MyError
# This will raise an exception of type MyError.
raise MyError("Something went wrong")
# including a custom message with the exception
Catching a user-defined exception :
- If we want to catch an exception, we can do so using a try..except block.
- Basic syntax for catching user-defined exceptions :
try:
raise MyError
except MyError:
print("Caught MyError")
# This will catch any exceptions of type MyError.
Code Sample for user defined exceptions
- Below we have a full code sample for user defined exceptions in python.
- Code Sample :
class MyException(Exception):
def __init__(self, msg):
self.msg = msg
def show_details(name, id):
try:
print("Inside try block")
if id < 0:
raise MyException("Id should be a Positive number.")
else:
print(f"The name is : {name}")
print(f"The id is : {id}")
except MyException as e:
print("Inside MyException except block : ", e)
show_details("John", -5)
print()
show_details("Alice", 1)
# Output
# Inside try block
# Inside MyException except block : Id should be a Positive number.
# Inside try block
# The name is : Alice
# The id is : 1
Catching multiple user-defined exception
- We can also catch multiple user defined exception types by providing a tuple of exception types to the
except
keyword. - Basic syntax for catching multiple user-defined exceptions :
try:
raise MyError
except (MyError, AnotherError):
print("Caught an error")
- Code Sample :
class MyException(Exception):
def __init__(self, msg):
self.msg = msg
class MySecondException(Exception):
def __init__(self, msg):
self.msg = msg
def show_details(name, id):
try:
print("Inside try block")
if id < 0:
raise MyException("MyException : Id should be a Positive number.")
elif id > 1000:
raise MySecondException("MySecondException : Id cannot be greater than 1000.")
else:
print(f"The name is : {name}")
print(f"The id is : {id}")
except (MyException, MySecondException) as e:
print(e)
show_details("John", -5)
print()
show_details("Alice", 2000)
# Output
# Inside try block
# MyException : Id should be a Positive number.
# Inside try block
# MySecondException : Id cannot be greater than 1000.
Getting type of user-defined exception :
- We can also get the type of an exception using the
type()
function. - For example:
try:
raise MyError
except MyError as e:
print("Caught MyError:", type(e))
# This will print the type of the exception.
- Code Sample :
class MyException(Exception):
def __init__(self, msg):
self.msg = msg
class MySecondException(Exception):
def __init__(self, msg):
self.msg = msg
def show_details(name, id):
try:
print("Inside try block")
if id < 0:
raise MyException("Id should be a Positive number.")
elif id > 1000:
raise MySecondException("Id cannot be greater than 1000.")
else:
print(f"The name is : {name}")
print(f"The id is : {id}")
except (MyException, MySecondException) as e:
type_excep = type(e)
print(e)
print (f"Type of Exception : {type_excep}")
show_details("John", -5)
print()
show_details("Alice", 2000)
# Output
# Inside try block
# Id should be a Positive number.
# Type of Exception : <class '__main__.MyException'>
# Inside try block
# Id cannot be greater than 1000.
# Type of Exception : <class '__main__.MySecondException'>
Prev. Tutorial : Built-in exceptions
Next Tutorial : Modules