Beginner Level
Intermediate Level
Advanced Level
Introduction
In this tutorial, we will focus on how to insert data into a MySQL table using Python. We will cover different ways of inserting values into a MySQL Database Table, including pure Python code and Python libraries such as mysql.connector. By the end of this tutorial, you will have a clear understanding of how to insert values into a MySQL table using Python and be able to apply this knowledge to your Python Database Management tasks.
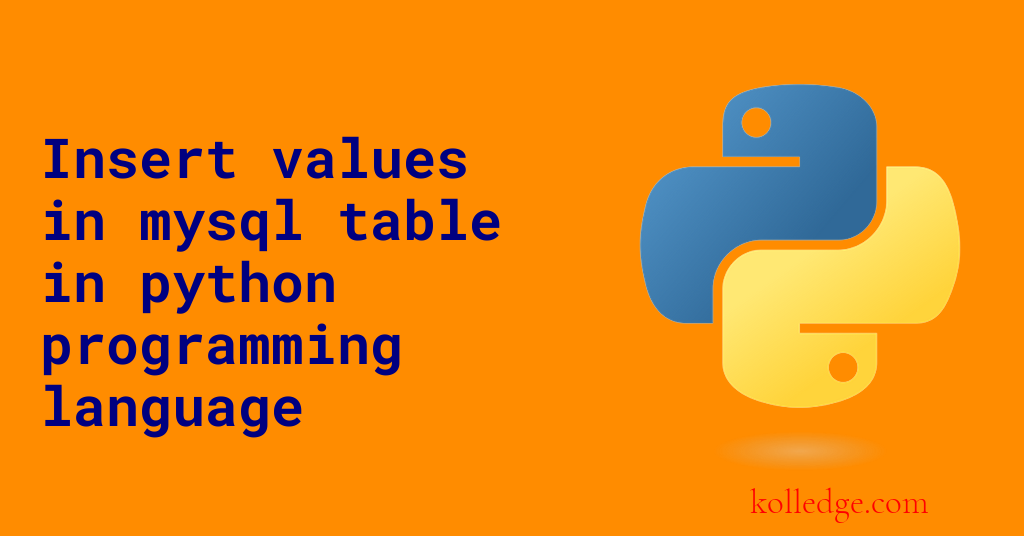
Table of Contents :
- How to Insert values in table in MySQL with Python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: Insert Values in MySQL Table
- Step 5: Commit the changes
How to Insert values in table in MySQL with Python :
- Follow the steps given below to Insert values in table in MySQL with python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: Insert Values in MySQL Table
- Step 5: Commit the changes
Step 1: Install the Required Packages
- The first step is to ensure that you have installed the required packages on your machine.
- The most popular packages for connecting to MySQL in Python are
- mysql-connector and
- PyMySQL.
- You can install these packages using pip through the command line.
- Code Sample :
!pip install mysql-connector-python
!pip install PyMySQL
Step 2: Connect to the MySQL Database
- To create a new database in MySQL using Python, we must have a connection to the MySQL server.
- To connect to a MySQL server in Python we can use either
- mysql.connector or
- PyMySQL package
- We need to provide the necessary connection parameters to establish the connection.
- We need to pass the following parameters to the Connection object:
- host : the hostname of the MySQL server user
- user : username for the MySQL account
- password : password for the MySQL account database
- database : The name of the database to connect to
- To study package specific code for connecting to MySQL read our tutorial on connecting to MySQL server.
Step 3: Create a Cursor Object
- After establishing a connection to the MySQL server, your next step is to create a cursor object.
- This is done using the cursor() method provided by mysql.connector or PyMySQL.
- Example:
cursor = mydb.cursor()
Step 4: Insert Values in MySQL Table
- To insert values into an existing MySQL table using Python, use the `INSERT INTO` statement.
- Use the `execute()` method on the cursor object to execute the query.
- Example:
# Insert Values
query = "INSERT INTO employees (id, name) VALUES (1, 'John')"
cursor.execute(query)
#Print message to confirm Inserting Values
print("Values Inserted successfully!")
Step 5: Commit the changes
- Finally, make sure to commit the changes to the MySQL table
- This can be done using the commit() method provided by mydb.
- Example:
mydb.commit()
#Print message to confirm changes
print("Changes successfully saved to the database!")
Prev. Tutorial : Drop table in mysql using Python
Next Tutorial : Using sql select query