Beginner Level
Intermediate Level
Advanced Level
Introduction
In Python, manipulating strings is made easy with a variety of built-in features, including the ability to slice a string. String slicing is the process of extracting a portion of a string based on a specified starting and ending point, or a particular step size. Slicing strings is a critical skill in Python programming since strings are often an essential part of any program, ranging from simple scripts to more complex applications. In this tutorial, we will explore the various techniques involved in slicing a string in Python, with practical examples that demonstrate the concepts in clear and concise language.
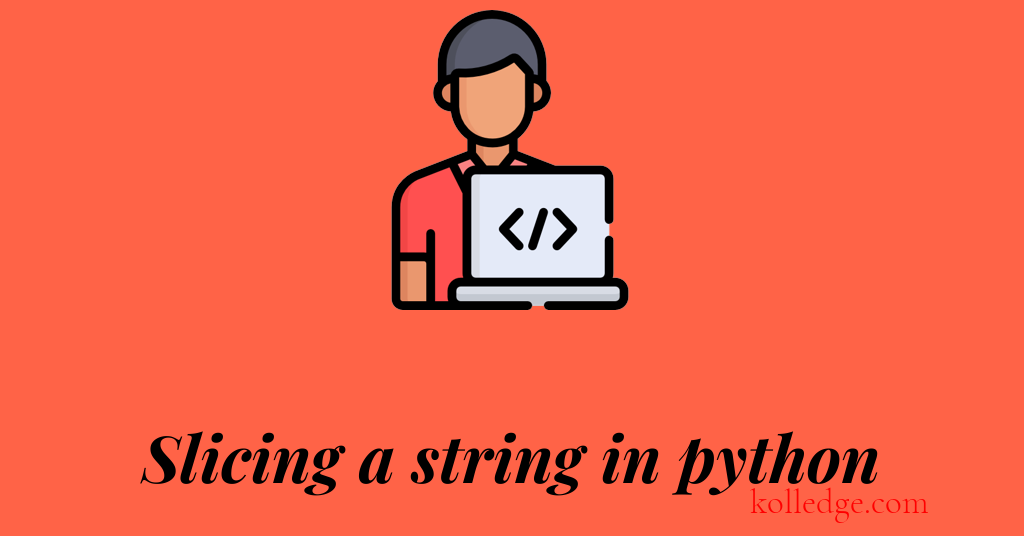
Table of Contents :
- Different methods of Slicing a string in python :
- Basic string slicing
- Negative index slicing
- Step slicing
- Slicing and copying
- Slicing with string methods
- Slicing using split() method
Different methods of Slicing a string in python :
- In python we can slice a string in different ways :
- Basic string slicing
- Negative index slicing
- Step slicing
- Slicing and copying
- Slicing with string methods
- Slicing using split() method
Basic string slicing:
- We can use square brackets
[ ]
and index numbers to access individual characters of a string. - Separate the start and end indices with a colon
:
to slice a part of the string. - Code Sample :
my_string = "Hello, Kolledge Scholars"
print(my_string[0])
print(my_string[7:15])
# Output
# H
# Kolledge
Negative index slicing:
- We can use negative index numbers to access characters from the end of the string.
- Code Sample :
my_string = "Hello, Kolledge Scholars"
print(my_string[-1])
print(my_string[-8:-1])
# Output
# s
# Scholar
Step slicing:
- We can use a third parameter in the slicing syntax to specify the step or interval between characters.
- Code Sample :
my_string = "12345678"
print(my_string[::2])
print(my_string[::-1])
print(my_string[-1:-5:-1])
# Output
# 1357
# 87654321
# 8765
Slicing and copying a string:
- We can use the slicing syntax to copy a string to a new variable.
- Code Sample :
my_string = "Hello, Kolledge Scholars"
new_string = my_string[7:15]
print(new_string)
# Output
# Kolledge
Slicing with string methods:
- We can use string methods such as
find()
,index()
, andcount()
to find the start and end indices for slicing a string. - Code Sample :
my_string = "Hello, Kolledge Scholars"
start_index = my_string.find("Kolledge")
end_index = start_index + len("Kolledge")
sliced_string = my_string[start_index:end_index]
print(sliced_string)
# Output
# Kolledge
Using split() method to slice a string into a list of sub-strings:
- We can use the
split()
method to split a string into a list of sub-strings based on a delimiter. - We can then use the indexing syntax to access individual sub-strings.
- Code Sample :
my_string = "Hello, Kolledge, Scholars"
split_string = my_string.split(", ")
print(split_string)
print(split_string[1])
# Output
# ['Hello', 'Kolledge', 'Scholars']
# Kolledge
Prev. Tutorial : Reversing a string
Next Tutorial : Splitting a string