Beginner Level
Intermediate Level
Advanced Level
Introduction
Python provides a built-in JSON library that allows developers to decode and encode JSON data effortlessly. This makes it easy for programs to exchange data in a standardized format. In this tutorial, we will discuss how to read a JSON file in Python using the standard library and other third-party libraries, and how to manipulate the data in a meaningful way. By the end of this tutorial, you will be able to read a JSON file with Python and use the data to power your applications.
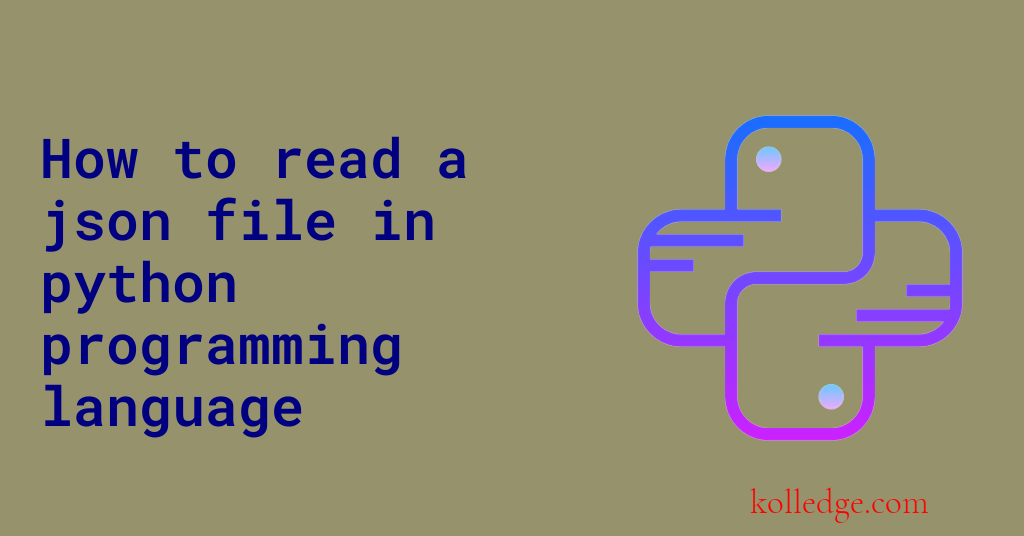
Table of Contents :
- Opening and Reading JSON Files in Python
- Accessing Values from JSON Data
- Working with Nested JSON Data
- Handling Errors when Reading JSON Files
Opening and Reading JSON Files in Python :
- In order to read a JSON file in Python, you can use the built-in
json
module. - The
json
module provides aload()
method to read data from a JSON file. - Code Sample :
import json
with open("example.json", "r") as f:
data = json.load(f)
print(data)
Accessing Values from JSON Data :
- Once you have loaded the JSON data into Python, you can access its values through standard Python methods.
- Use the key names to access specific values in the JSON data.
- Code Sample :
import json
with open("example.json", "r") as f:
data = json.load(f)
print(data["name"])
print(data["age"])
print(data["subjects"][0])
Working with Nested JSON Data
- JSON data can be nested with key/value pairs or arrays within key/value pairs.
- To access nested values, use multiple keys in succession with brackets to navigate to the desired value.
- Code Sample :
import json
with open("example.json", "r") as f:
data = json.load(f)
print(data["address"]["city"])
print(data["grades"][1]["marks"][2])
Handling Errors when Reading JSON Files
- Errors can occur when reading and parsing JSON files in Python, such as invalid syntax or missing keys.
- You can use error handling to gracefully handle these errors.
- Use a try-except statement with the
JSONDecodeError
exception to handle decoding errors. - Code Sample :
import json
try:
with open("example.json", "r") as f:
data = json.load(f)
except json.JSONDecodeError as e:
print(f"JSON decoding error: {e}")
Prev. Tutorial : Working with json
Next Tutorial : Writing to a json file