Beginner Level
Intermediate Level
Advanced Level
Introduction
Two features of python that help reduce the complexity of code and improve its readability are Iterators and Iterables. Although these terms sound similar, they have different meanings and functions. An Iterator is an object in Python that allows us to loop through an iterable, while an Iterable is any object that can be looped over. In this tutorial, we will take a closer look at the differences between Iterators and Iterables in Python and how they are used in coding.
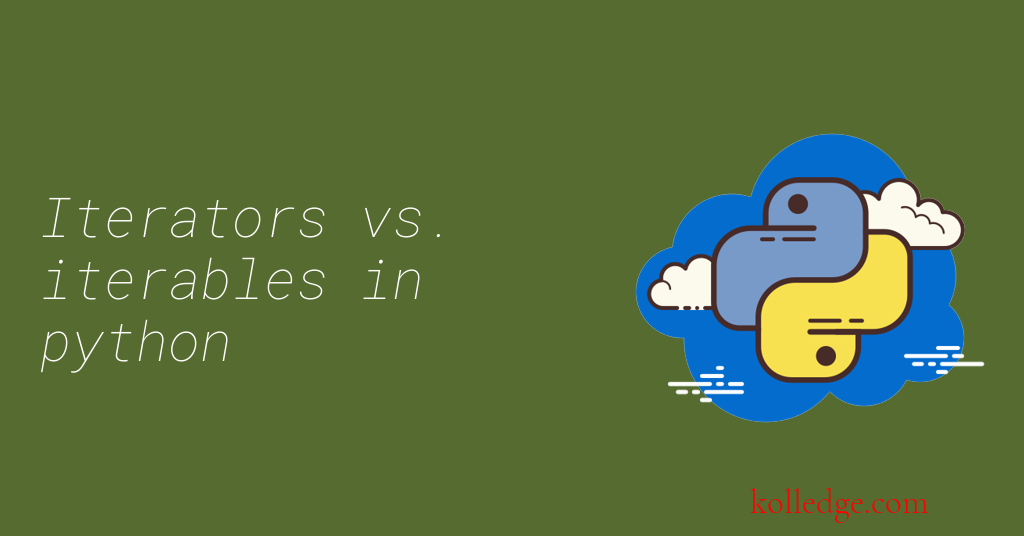
Table of Contents :
- What are Iterators in Python
- What are Iterables in Python
- Iterator vs Iterable
- List Iterator in Python
- Python Iterator and Iterable
- Split an Iterator from an Iterable
What are Iterators in Python :
- An iterator is an object that can be iterated upon, meaning that you can traverse through all the values it contains.
- In Python, an iterator is an object which implements the iterator protocol, which consists of two methods :
- __iter__() and
- __next__().
- An iterator is an object that represents a stream of data. It produces one value at a time using the
next()
- An iterator raises a
StopIteration
exception when there are no more values left to produce. - Code Sample :
iterator = iter([1, 2, 3])
print(next(iterator)) # Output: 1
print(next(iterator)) # Output: 2
print(next(iterator)) # Output: 3
print(next(iterator)) # Output: StopIteration
What are Iterables in Python :
- An iterable is an object that can produce an iterator using the
iter()
function. - Examples of iterables include lists, tuples, dictionaries, and sets.
- Code Sample :
iterable = [1, 2, 3]
iterator = iter(iterable)
print(next(iterator)) # Output: 1
print(next(iterator)) # Output: 2
print(next(iterator)) # Output: 3
print(next(iterator)) # Output: StopIteration
Iterator vs Iterable
- An iterator is an object that represents a stream of data. An iterable is an object that can return an iterator.
- Most of the built-in containers in Python like: list, tuple, string etc. are iterables.
- The built-in iter() function can be used to create an iterator from an iterable:
- Code Sample :
mylist = [1, 2, 3]
myiter = iter(mylist)
print(next(myiter)) 1
print(next(myiter)) 2
print(next(myiter)) 3
- Code Sample :
#creating an iterator from a list
mylist = [1, 2, 3]
myiter = iter(mylist)
# traversing through the iterator
for x in myiter: print(x) 1 2 3
- Code Sample :
#creating an iterator from a tuple
mytuple = ("apple", "banana", "cherry")
myit = iter(mytuple)
# traversing through the iterator
for x in myit:
print(x) apple banana cherry
- Code Sample :
#creating an iterator from a string
mystr = "banana"
myit = iter(mystr)
# traversing through the iterator
for x in myit:
print(x)
b a n a n a
List Iterator in Python :
- A list is iterable, which means it can produce an iterator.
- A list iterator is an object that iterates through a list.
- Code Sample :
my_list = [1, 2, 3]
print(iter(my_list)) # Output:
Python Iterator and Iterable :
- An object is iterable if it defines the
__iter__()
method and returns an iterator object. - An object is an iterator if it defines both the
__iter__()
and__next__()
methods. - The
__next__()
method should return the next item in the sequence or raise aStopIteration
exception if there are no more items. - Code Sample :
class MyRange:
def __init__(self, start, end):
self.current = start
self.end = end
def __iter__(self):
return self
def __next__(self):
if self.current >= self.end:
raise StopIteration
value = self.current
self.current += 1
return value
my_range = MyRange(1, 4)
for value in my_range:
print(value) # Output: 1, 2, 3
Split an Iterator from an Iterable :
- You can separate an iterator from an iterable using the
iter()
andnext()
functions. - This allows for more control over how you interact with the iterator and the iterable.
- Code Sample :
iterable = [1, 2, 3]
iterator = iter(iterable)
try:
while True:
value = next(iterator)
print(value)
except StopIteration:
pass
Prev. Tutorial : Storing float
Next Tutorial : iter() function