Introduction
One of the most common operations in programming is the concatenation of strings, which is the process of combining multiple strings into a single one. Fortunately, Python provides a variety of built-in methods and functions for concatenating strings in different ways. In this tutorial, we will guide you through the basics of string concatenation in Python, including the different methods available, best practices, and common use cases. Whether you are a beginner or an experienced Python developer, mastering string concatenation is a crucial skill that can help you write more efficient and concise code.
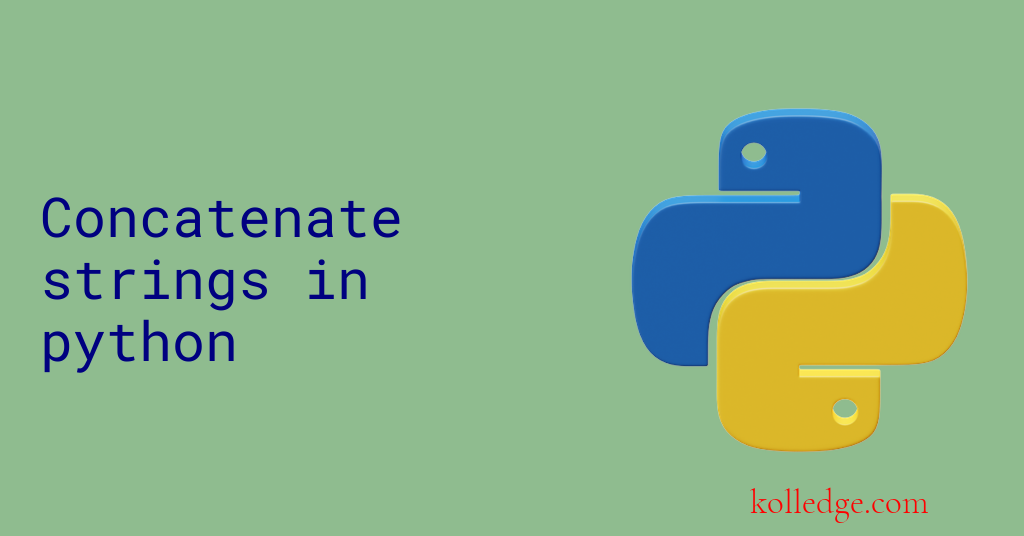
Table of Contents :
- Concatenating Strings
- Using the + Operator
- Using the join() Method
- Using f-strings
- Using the format() Method
- Using the % Formatting
- Using String Slicing
- Concatenating Strings with Non-Strings
Concatenating Strings :
In Python we can concatenate strings using the following methods :
- Using the + Operator
- Using the join() Method
- Using f-strings
- Using the format() Method
- Using the % Formatting
- Using String Slicing
Using the + Operator :
- We can use the concatenation operator to concatenate two or more strings together.
- Code Sample :
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result)
# Output
# Hello World
Using the join() Method :
- We can use the
join()
method to concatenate strings from within an iterable, such as a list or tuple. - Code Sample :
my_list = ["Hello", "World"]
separator = " "
result = separator.join(my_list)
print(result)
# Output
# Hello World
Using f-strings :
- We can use the f-string syntax to interpolate variables and literal strings together.
- Code Sample :
name = "Alice"
age = 30
result = f"My name is {name} and I am {age} years old."
print(result)
# Output
# My name is Alice and I am 30 years old.
Using the format() Method :
- We can use the format() method to format strings with placeholders.
- Code Sample :
str1 = "Hello"
str2 = "World"
result = "{} {}".format(str1, str2)
print(result)
# Output
# Hello World
Using the % Formatting :
- We can use the modulo operator
%
to format strings with placeholders. - Code Sample :
str1 = "Hello"
str2 = "World"
result = "%s %s" % (str1, str2)
print(result)
# Output
# Hello World
Using String Slicing :
- We can use string slicing to concatenate parts of strings together.
- Code Sample :
str1 = "Hello"
str2 = "Kolledge"
result = str1 + " " +str2[4:]
print(result)
# Output
# Hello edge
Concatenating Strings with Non-Strings :
- We can use
str()
method to convert data type of non-strings such as numbers to strings before concatenating. - Code Sample :
num1 = 10
num2 = 5.5
str1 = "The result is: "
result = str1 + str(num1 + num2)
print(result)
# Output
# The result is: 15.5
Prev. Tutorial : Getting substring
Next Tutorial : Modify or delete a string