Introduction
As we begin learning to code in Python, one of the first things we encounter is the significance of properly indented code. Unlike most programming languages, Python uses indentation to signify blocks of code, instead of using brackets or other punctuation. In this tutorial, we will explore the importance of indentation in Python and how to use it with statements. We will cover the different types of statements in Python, and how they can be used within indented code blocks. By the end of this tutorial, you will have a strong foundation in using statements and indentation in Python programming.
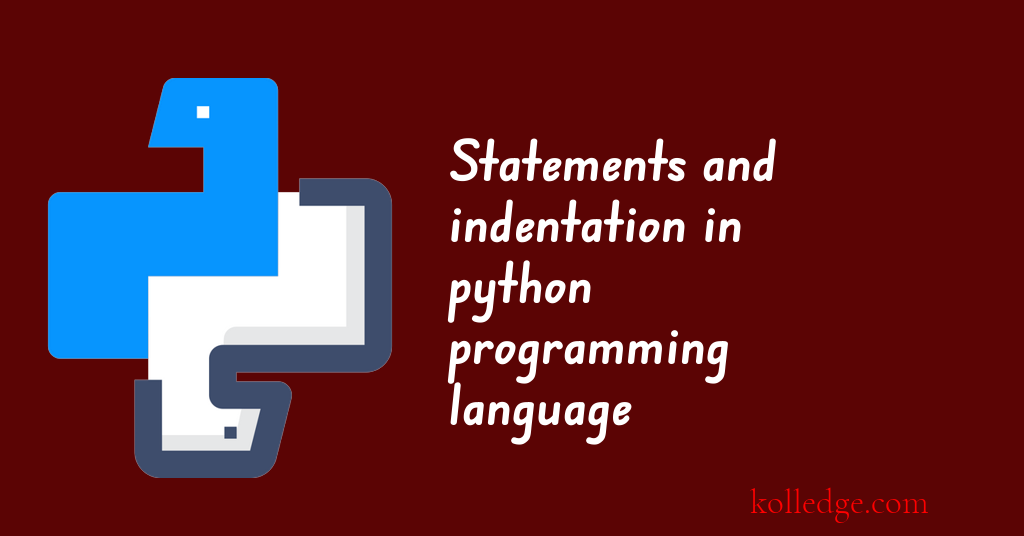
Table of Contents :
- Statements in Python
- Multi-line statements in Python
- Different types of statements in Python based on logical structure
- Empty statement
- Simple statements
- Compound statements
- Different types of statements in Python based on functionality
- Code Blocks and Indentation in Python
Statements in Python
- Statement is an instruction given to the python interpreter for execution.
- In other words a python statement is a single logical line written in Python.
- Most programming languages terminate statements using a semi colon (;).
- In python statements are terminated using newline character( \n ).
- In Python usually a single statement is written on a single line.
- But we can write multiple statements on a single line. The statements should be separated by semicolons.
Multi-line statements in Python
- In python we can write a single statement on multiple lines.
- This might be necessary for readability purposes. Long statements can be continued to next line.
- We can write statement on multiple lines using -
- explicit continuation
- implicit continuation
Explicit continuation
Explicit continuation is done :
- using backslash ( \ ) - Python statements are terminated by the token NEWLINE character but we can write a single statement over multiple lines using backslash.
Implicit continuation
Implicit continuation is done :
- using parentheses ( () ) - Parentheses can be used to create multi-line statements by just enclosing the whole statement within parenthesis. This ends the need of using backslash for continuation.
- using square brackets ( [ ] ) - Square brackets are used to create lists in Python. While creating lists we can write each list element on separate line for better readability. Thus extending a single statement to multiple lines.
- using braces ( {} ) - Braces are used to create dictionaries in python. While creating dictionaries we can write each key value pair on separate line for better readability. Thus extending a single statement to multiple lines.
Different types of statements in Python based on logical structure
- Python statements can be of three types on basis on logical structure -
- Empty statement
- Simple statements
- Compound statements
- Python Empty statement
- In Python pass statement is an empty statement.
- Python simple statements
- Declaration statements
- Calculation statements
- Expression statements
- del statement
- return statement
- import statement
- continue statement
- break statement
- Python Compound statements
- Control flow statements - if statements
- Looping statements - while statement, for statement
- Exception handling - try statement
- with statement.
- Pattern Matching - match statement
Different types of statements in Python based on functionality
- On basis of functionality Python statements can be categorized in different types like :
- Print statements
- Assignment statements
- Conditional statements
- Looping statements etc.
Code Blocks and Indentation in Python
- In a programming language a code block is a group of statements.
- All statements in a code block are usually grouped together as they serve a single logical purpose.
- A lot of programming languages use braces '{ }' to define code blocks.
- In Python, indentation is used to indicate the beginning and end of a code block.
- In Python all code blocks that are at same nested level must be indented to the same amount.
- If a block is nested within another block then it is simply indented further towards right.
- Indentation can be of four spaces or one tab.
- It is not possible to mix spaces and tabs for indentation.
Prev. Tutorial : Keywords and Identifiers
Next Tutorial : Namespaces