Introduction
One of the key advantages of Python is its ability to handle a wide range of operations related to reading, writing and manipulating files. Opening a file is an essential operation in any programming language as it forms the basis for reading, writing, and manipulating data. In this tutorial, we will explore how to open a file in Python, different modes of opening files, and crucial things to keep in mind while opening files in Python. Join us as we delve into the world of file handling in Python!
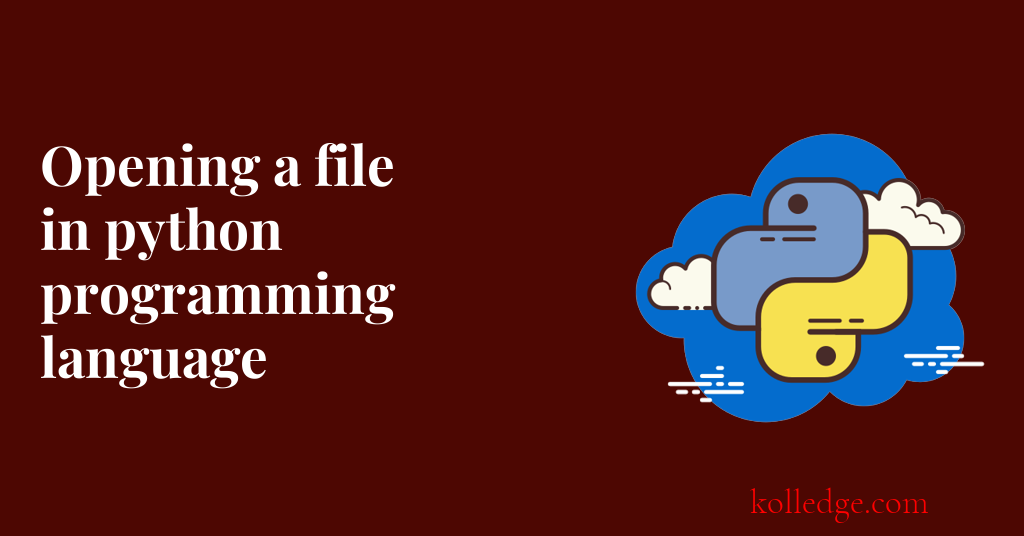
Table of Contents :
- Python open function
- Access Modes for Opening a file
- Different characters for mentioning the file opening modes
- Steps For Opening File in Python
- Different scenarios for opening a file in python
- How to open a file in read mode
- How to open a file in write mode
- How to open a file in append mode
- How to open a file with relative path
- How to open a file using with statement
- How to open a file for multiple operations
- How to open a binary file
- How to Handle the FileNotFoundError
- How to close a file
Python open function
- Python has a built-in function open() to open a file.
- This function returns a file object, also called a handle, as it is used to read or modify the file accordingly.
- We can specify the mode while opening a file.
- In mode, we specify whether we want to
- read 'r',
- write 'w' or
- append 'a' to the file.
- If we don't specify the mode, it defaults to 'r'.
- The syntax of the
open()
function is :file_object = open(file_name, access_mode, buffering)
parameters we can pass to the open() function
- file_name : Name of the file to be opened.
- access_mode : Access modes for opening a file. For example, `r`, `w`, `a`, `x` etc.
- buffering (Optional): 0 to turn off buffering. Any positive value to indicate the buffer size.
Access Modes for Opening a file
Mode | Description |
---|---|
r | Read mode, open the file for reading. |
w | Write mode, open the file for writing. If the file already exists, it will be truncated. |
a | Append mode, open the file for writing. If the file does not exist, it will be created. |
x | Exclusive creation mode. It opens a file for writing, if the file already exists, the open() method call will fail. |
t | Text mode, used by default if not mentioned. |
b | Binary mode, used to read or write binary data. |
Different characters for mentioning the file opening modes
`+`: Read and Write mode. The `r+` mode is used to open a file for both reading and writing operations.
`U`: This mode allows universal newline support. This mode is only available in Python 2.
Steps for opening file in python
- Open a file in the desired mode using the
open()
method. The function returns a file object. - Perform read or write operations on the file.
- Close the file using the
close()
method.
Different scenarios for opening a file in python
How to open a file in read mode
- We can open a file in read mode by passing the value ‘r’ for the mode argument of the open() function.
- The
read()
method can then be used to read the file. - Code Sample :
file = open("example.txt", "r")
print(file.read())
file.close()
# "example.txt" is the name of the file we want to open
# "r" indicates that we want to read the file.
# The code will print the entire contents of the file.
How to open a file in write mode
- We can open a file in write mode by passing the value ‘w’ for the mode argument of the open() function.
- The
write()
method can then be used to write to the file. - If the file already exists, opening it in write mode will delete the old contents of the file.
- Code Sample :
file = open("example.txt", "w")
file.write("Hello, World!")
file.close()
# "example.txt" is the name of the file we want to open
# "w" indicates that we want to write to the file.
# The code will create the file if it does not exist
# will overwrite the content if it does exist.
How to open a file in append mode
- We can open a file in append mode by passing the value ‘a’ for the mode argument of the open() function.
- The
write()
method can then be used to write to the file. - If the file already exists, data will be written to the end of the file, without deleting the old contents.
- Code Sample :
file = open("example.txt", "a")
file.write("\nHello, World Again!")
file.close()
# "example.txt" is the name of the file we want to open
# "a" indicates that we want to append to the file.
# The code will create the file if it does not exist
# and will append the content if it does.
How to open a file with relative path
- The
os.path.join()
method is used to join one or more path components in Python. - This method will create a path with the appropriate separator, based on the operating system.
- We can pass this file path as the first argument of the open() function to open the file.
- Code Sample :
import os
file_path = os.path.join("directory", "example.txt")
file = open(file_path, "r")
print(file.read())
file.close()
How to open a file using with statement
- The
with
statement is a better way to open files. - It automatically closes the file after the block of code is executed.
- Code Sample :
with open("example.txt", "r") as file:
print(file.read())
How to open a file for multiple operations
- The `r+` mode is used to open a file for both reading and writing operations.
- We can then use the
read()
andwrite()
methods in combination to perform multiple operations on a file. - Code Sample :
with open("example.txt", "r+") as file:
print(file.read())
file.write("\nAdded a new line!")
How to open a binary file
- The `rb` mode is used to open binary files.
- The
read()
method can be used to read binary data. - Code Sample :
with open("binaryfile.png", "rb") as file:
print(file.read())
How to Handle the FileNotFoundError
- The python interpreter raises a
FileNotFoundError
error if the file specified in the open() function does not exist. - The
os.path.exists()
method is used to check whether a file path exists or not. - We can also use try-except blocks to handle file-not-found errors.
- Code Sample :
import os
file_path = os.path.join("directory", "example.txt")
if os.path.exists(file_path):
file = open(file_path, "r")
print(file.read())
file.close()
else:
print("File does not exist.")
How to close a file
- It is always a good practice to close the file after using it.
- The
close()
method is used to close a file.
Prev. Tutorial : Creating a File
Next Tutorial : Reading a file