Beginner Level
Intermediate Level
Advanced Level
Introduction
One of the fundamental concepts in programming is iterating over elements in a data structure. Python provides an easy way to loop through strings using a variety of techniques, including various types of loops. By the end of this tutorial, you will have a thorough understanding of how to iterate through strings in Python and be better equipped to handle similar problems that involve iteration or looping in various contexts. So, let's dive in!
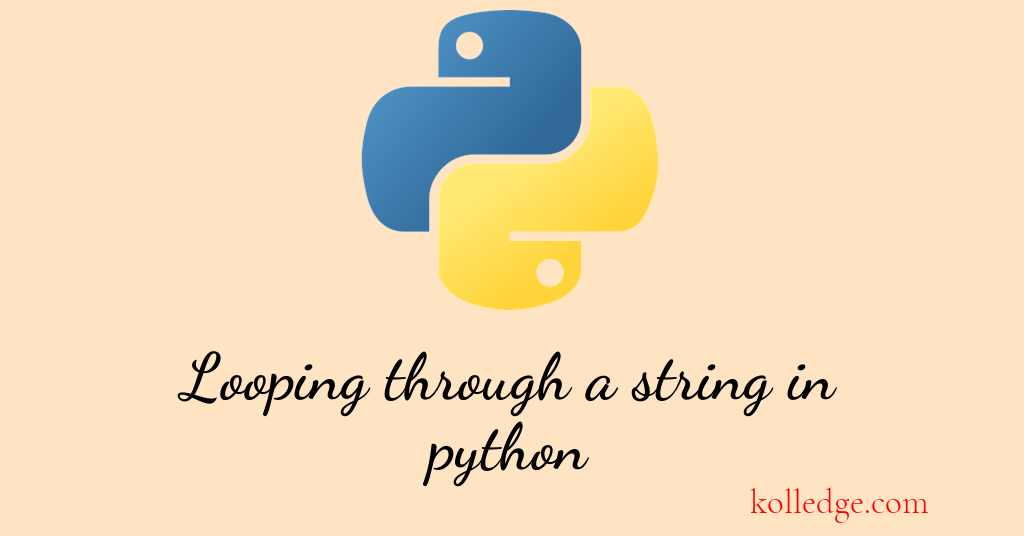
Table of Contents :
- Different ways of looping through a string in Python
- Using a for loop
- Using a while loop
- Using range() function
- Skipping characters
- Breaking out of the loop
Different ways of looping through a string in Python :
- In Python we can loop through a string in different ways :
- Using a for loop
- Using a while loop
- Using range() function
Using a for loop:
- We can use a for loop with a variable to iterate through each character of a string.
- The variable can be of any name you choose, and will be assigned to each character of the string in turn.
- Code Sample :
my_string = "Hello"
for character in my_string:
print(character)
# Output
# H
# e
# l
# l
# o
Using a while loop:
- We can use a while loop with an index variable to iterate through each character of a string.
- We can use the
len()
function to get the length of the string to know when to stop the loop. - Code Sample :
my_string = "Hello"
index = 0
while index < len(my_string):
print(my_string[index])
index += 1
# Output
# H
# e
# l
# l
# o
Using range() function:
- We can use the
range()
function with the length of the string as the argument to iterate through each character of a string using index positions. - Code Sample :
my_string = "Hello"
for index in range(len(my_string)):
print(my_string[index])
# Output
# H
# e
# l
# l
# o
Skipping characters:
- We can use the continue statement to skip a particular character if it meets a certain condition.
- Code Sample :
my_string = "Hello"
for character in my_string:
if character == 'l':
continue
print(character)
# Output
# H
# e
# o
Breaking out of the loop:
- Use the break statement to break out of the loop once a certain condition is met.
- Code Sample :
my_string = "Hello"
for character in my_string:
if character == 'l':
continue
print(character)
# Output
# H
# e
Prev. Tutorial : Accessing characters of string
Next Tutorial : Reversing a string