Beginner Level
Intermediate Level
Advanced Level
Introduction
Object-oriented programming is a powerful programming paradigm that allows you to break down complex problems into manageable parts, by creating and manipulating objects. In this tutorial, you will learn about various object properties such as class, methods, and inheritance, and how to create and use them in your Python programs. Whether you are a beginner or already familiar with Python programming, this tutorial will give you a solid foundation in object-oriented programming in Python. So let's dive in and explore the wonderful world of Object-Oriented Programming with Python!
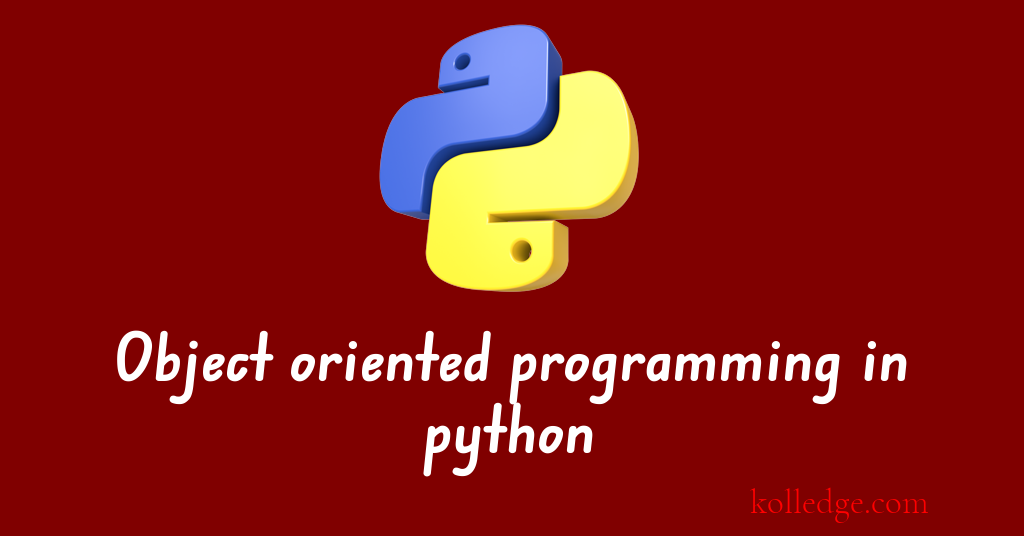
Table of Contents :
- Object oriented programming using Python
- Defining a class in Python
- Creating objects in Python
- Constructors in Python
- Destructors in Python
- Class Attributes
- Instance Variables
- Class variables
- Methods of the Class
- Instance Methods
- Class Methods
- Static methods
- Creating a class property
- Descriptors in Python
- Encapsulation in Python
- Polymorphism in Python
- Inheritance in Python
- Implementing Single inheritance in Python
- Implementing Multiple inheritance in Python
Object oriented programming using Python
- In our OOPs concepts tutorial we have read that object is an instance of class.
- i.e. to create an object, first we need to define a class.
- Then from this class we can create multiple objects.
- Class is a template for defining an object.
- Every object of a class has :
- state - defined by the data members of the class
- behavior - defined by the data functions of the class
- identity - every object of a class is different from other objects.
- Note # :
- Everything in Python is treated as an object, i.e be it variables, constants, functions, data-types, keywords, operators etc. everything in python is an object of some class or the other.
- Functions are treated as first class objects in python. This concept is used in advanced concepts like closures.
Defining a class in Python :
- In Python a class is defined by using the
class
keyword. - The basic syntax of creating a class in Python is as follows :
class Classname:
# Body of the class
# Defining attributes and methods.
Defining a real class in Python :
- Below we have a real python code of defining an
Employee
class. - The class first defines a class variable
company_address
- Then a constructor is defined to initialize the data members -
- name
- id
- desig
- Then two member functions are defined :
- calculate_salary()
- record_attendance()
class Employee:
company_address = "12th street"
# state - initializing data members
def __init__(self, name, id, desig):
# data members (instance variables)
self.name = name
self.id = id
self.desig = desig
# Behavior (instance methods)
def calculate_salary(self):
# Code to calculate salary
# Behavior (instance methods)
def record_attendance(self):
# Code to record attendance
Creating an object of the class - Instantiating the class
- An instance of the class is created when we create a new object of the class.
- The basic syntax of creating a new object of a class is
object_name = Classname(data_member1, data_member2, data_member3,...)
- In our previous example an object of the employee class can be created as :
steve = Employee('Steve', 1203, 'Project Manager')
Constructors in Python :
- The process of creating an object is divided into two parts :
- creating an object using the
__new__
method. - initializing the data members of the object using the
__init__
method.
- creating an object using the
- In Python a constructor is used to create and initialize an object of the class.
- A constructor is a special method of the class.
- We have a detailed tutorial on constructors in Python which explains all these concepts and different types of constructors in detail.
Class Attributes
- Class attributes can be of two types :
- Instance variables
- Class variables (or static variables)
Instance variables :
- instance variables are the data members attached to the instances of a class.
- instance variables are created using the constructor of the class.
- Every object has its own copy of instance variables.
- instance variables are accessed by dot notation using the object name :
object_name.instance_var_name
- In the class defined in the previous section the instance variables are :
name
id
desig
- To access the instance variables for the object created above we can use the following code :
emp_name = steve.name
emp_id = steve.id
emp_desig = steve.desig
Class Variables :
- Class variables are defined for the class itself.
- Class variables are not attached to any particular object.
- Class variables are shared by all objects of the class.
- The value of the class variables remain the same for all objects.
- Class variables are also known as static variables.
- Only one copy of class variables is created and all objects share this copy.
- class variables are accessed by dot notation using the class name :
class_name.class_var_name
- In the class defined above
company_address
is a class variable :- the value of
company_address
will be the same for all the employees. - if the address of the company changes, it will change for all the employees.
- the value of
- The class variable
company_address
can be accessed using the syntax :
adress = Employee.company_address
Methods of the Class
- In a python class we can have three types of Methods :
- Instance methods
- Class methods
- Static methods
Instance Methods :
- Instance methods define the behavior of an object.
- A method is called instance method if it uses instance variables.
- An instance method can be used to modify the state of the object i.e. the data of the object.
- Instance methods are accessed by dot notation using the object name :
obj_name.instance_method()
- In the class example shown above, the instance methods are :
- calculate_salary()
- record_attendance()
- To access these methods for object
steve
we can use the following syntax :
salary = steve.calculate_salary()
Class Methods :
- Class methods are used to access the class variables.
- These methods are not attached to any object.
- Class methods can be created in two ways :
- using @classmethod decorator
- using the classmethod() function
- Both these methods are discussed in detail in our tutorial on class methods in python.
- class methods are accessed by dot notation using the class name :
class_name.class_method()
Static methods :
- Static methods are generic utility methods.
- These are neither instance methods nor class methods.
- In other words these methods do not have access to instance and class variables.
- Static methods can be created in two ways :
- using @staticmethod decorator
- using the staticmethod() function
- Both these methods are discussed in detail in our tutorial on static methods in python.
- static methods can be accessed :
- using the class name and dot operator.
- using the object name and dot operator.
- static methods are accessed by dot notation using the class name :
class_name.static_method()
Properties
- In Python, properties are a way of controlling access to class attributes.
- Properties can be used to execute the setter and getter methods of the class.
- The setter method is used to set the value of an attribute.
- The getter method is used to retrieve the value of an attribute.
- We have a dedicated tutorial on the topic of properties in Python.
Prev. Tutorial : What is Polymorphism
Next Tutorial : Constructors