Beginner Level
Intermediate Level
Advanced Level
Introduction
Python is a powerful and concise language that has become one of the most popular tools for software development and data analysis. It provides a range of features and functions that make programming easier and more efficient. One such feature is the use of docstrings. Docstrings provide a way to document code and communicate its purpose, functionality, and usage to other programmers. In this tutorial, we will discuss the importance and benefits of using docstrings in Python, how to write effective docstrings.
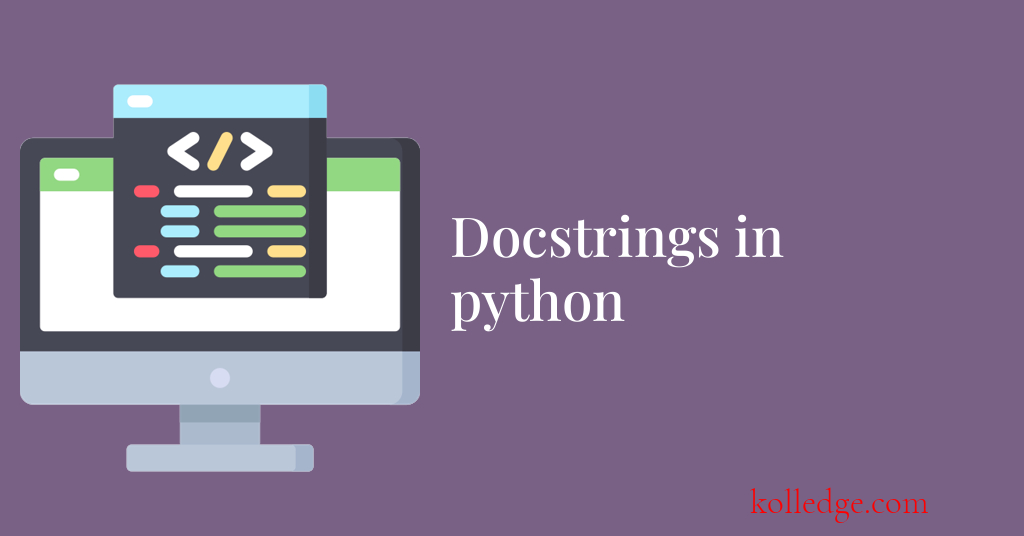
Docstrings in Python :
- Python is a very well documented programming language.
- The built-in help() function of python can be used to view the documentation of modules, functions, classes and keywords.
- docstrings are used to document a function in Python.
- If the first line of any function is a string. Python takes it as docstring.
- The help function then displays this docstring as the documentation of this function.
- Usually, multi-line strings are used as docstrings.
- In Python, docstrings are stored in the
__doc__
property of the function. - The
__doc__
property of a function can be accessed as follows -function_name.__doc__
- Docstrings can be accessed through the
__doc__
property or thehelp()
function. - Code Sample :
def my_func():
"""This is the docstring for function my_func.
docstrings are stored in the __doc__ property of the function.
docstrings of any function are used by
help function as documentation of that function"""
pass
print("printing docstring using __doc__:")
print(my_func.__doc__)
print()
print("printing docstring Using help:")
help(my_func)
# Output
# printing docstring using __doc__:
# This is the docstring for function my_func.
# We can use triple or double quotes to create docstrings.
# docstrings are stored in the __doc__ property of the function.
# docstrings of any function are used by
# help function as documentation of that function
#
# printing docstring Using help:
# Help on function my_func in module __main__:
#
# my_func()
# This is the docstring for function my_func.
# We can use triple or double quotes to create docstrings.
# docstrings are stored in the __doc__ property of the function.
# docstrings of any function are used by
# help function as documentation of that function