Beginner Level
Intermediate Level
Advanced Level
Introduction
The Ternary Operator is a concise method of writing an if-else statement in Python. It allows you to write a simple, one-liner code that assigns a value to a variable based on a condition. Ternary operators are also known as conditional expressions, and they can greatly simplify your code by avoiding the use of long if-else statements. In this tutorial, we will cover the syntax of the ternary operator, how to use it, and some examples to help solidify what you've learned. By the end of this tutorial, you will be able to use ternary operators with ease and make your code more concise and readable.
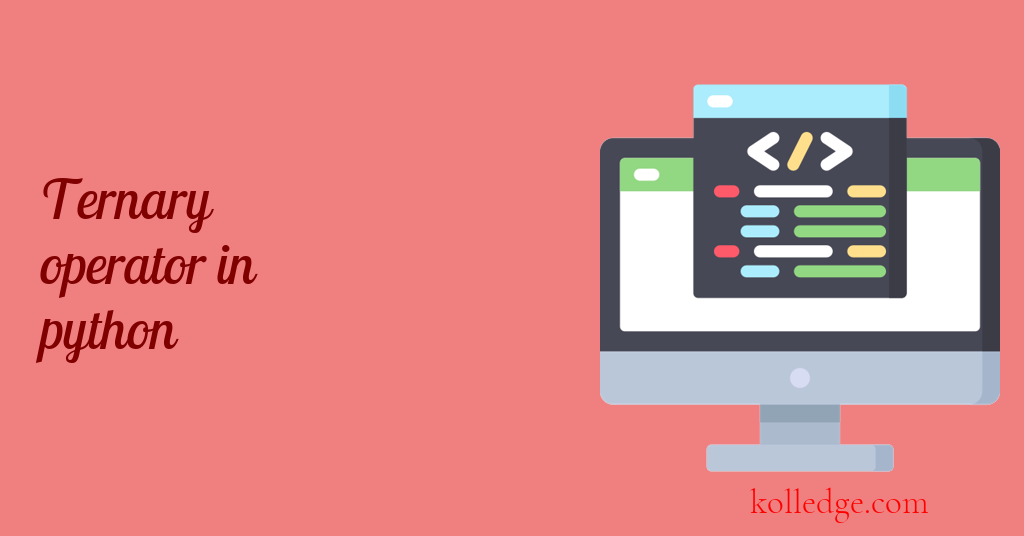
Table of Contents :
- Introduction to Python Ternary Operator
- Control flow for ternary operator
Introduction to Python Ternary Operator :
- Ternary operator is a concise way of writing simple if..else statement.
- The ternary operator works in same way as if..else statement, just the syntax is more concise.
- Suppose we have the following if...else statement :
if condition:
# code for True condition
return val_on_true
else:
# code for False condition
return val_on_false
- The above if..else statement can be rewritten in the following concise way using the ternary operator in Python :
result = val_on_true if condition else val_on_false
Control flow for ternary operator :
- the ternary operator evaluates the condition.
- If the condition is True, the value_if_true is returned.
- If the condition is False, the value_if_false is returned.
- the returned value is stored in the result variable
- Sample code for ternary operator :
qnt = 5
item_price = 200 if qnt >= 10 else 300
print(f"Price of the item = {item_price}")
# Output
# Price of the item = 300
- the left hand side of the assignment operator (=) is the variable item_price that stores the final returned value.
- the right hand side of the assignment operator (=) is the expression that evaluates the condition and returns a value accordingly.
- The value 200 is returned if the quantity is greater than or equal to 10 else value 300 is returned.
Prev. Tutorial : If…elif…else Statement
Next Tutorial : For loop