Beginner Level
Intermediate Level
Advanced Level
Introduction
Inheritance is one of the fundamental concepts of OOP, and it allows us to create new classes that share properties and methods of existing classes. This tutorial will teach you about inheritance in Python, including what it is, how it works, and how to use it effectively in your own code. Whether you're new to programming or an experienced developer, understanding inheritance is an essential part of becoming a proficient Python programmer.
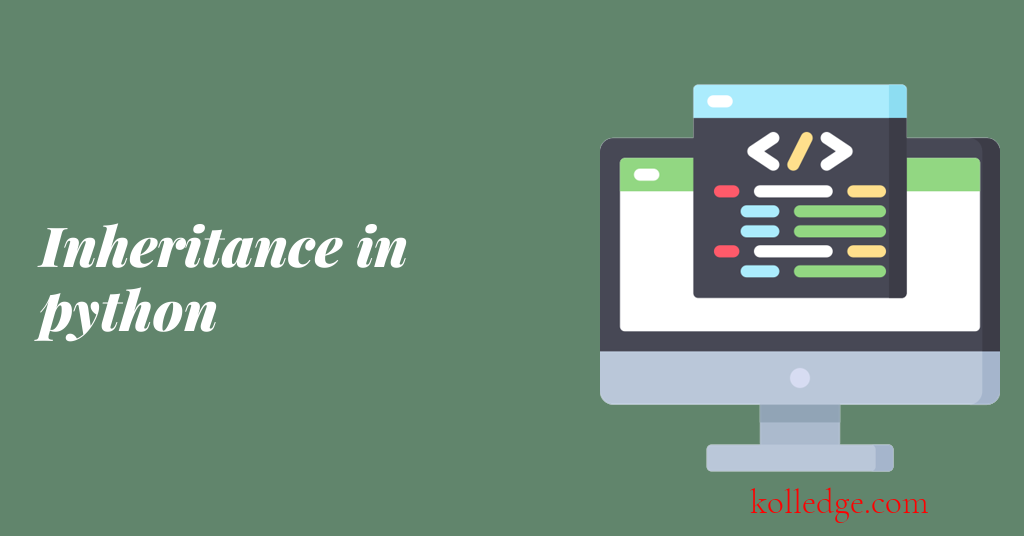
Table of Contents :
- Introduction to Inheritance in Python
- Creating a sub-class
- Overriding methods
- Accessing super-class methods
- Inheriting from multiple classes
- Types of inheritance in Python
Introduction to Inheritance in Python :
- Inheritance is a mechanism in OOPs that allows a class to inherit properties and methods from another class.
- The class that inherits is called the subclass or derived class or child class, while the class that is inherited from is called the super-class or base class or parent class.
- In Python, we achieve inheritance by passing the super-class inside parentheses after name of the inheriting class.
Creating a sub-class :
- To create a sub-class, we simply define a new class and specify the super-class from which it will inherit within parenthesis.
- Code Sample :
class Employee:
# Code for super-class
class ContractEmployees(Employee):
# Code for sub-class
Accessing Super-class Methods :
- Sub-classes can access the methods of their super-class by calling the method using the
super()
function followed by the method name. - The
super()
function returns a temporary object of the super-class which allows the sub-class to call its methods. - Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
def show_details(self):
print(f"The name of the Employee is : {self.name}")
print(f"The id of the Employee is : {self.id}")
print(f"The desig of the Employee is : {self.desig}")
class ContractEmployees(Employee):
def __init__(self, emp_name, emp_id, emp_desig, contract_term):
self.term = contract_term
# Calling constructor of parent class
super().__init__(emp_name, emp_id, emp_desig)
def emp_details(self):
# Calling show_details function of parent class
super().show_details()
print(f"The contract term of the Employee is : {self.term}")
emp = ContractEmployees("John", "2k1023", "Tech Asst.", "6 Months")
emp.emp_details()
# Output
# The name of the Employee is : John
# The id of the Employee is : 2k1023
# The desig of the Employee is : Tech Asst.
# The contract term of the Employee is : 6 Months
Overriding Methods :
- Sub-classes can override the methods of their super-class by defining a method with the same name in the sub-class.
- The sub-class method will be called instead of the super-class method when a method is called on an instance of the sub-class.
- Code Sample :
class Employee:
def __init__(self, emp_name, emp_id, emp_desig):
self.name = emp_name
self.id = emp_id
self.desig = emp_desig
def show_details(self):
print(f"The name of the Employee is : {self.name}")
print(f"The id of the Employee is : {self.id}")
print(f"The desig of the Employee is : {self.desig}")
class ContractEmployees(Employee):
def __init__(self, emp_name, emp_id, emp_desig, contract_term):
self.term = contract_term
# Calling constructor of parent class
super().__init__(emp_name, emp_id, emp_desig)
# Overriding method of the parent class
def show_details(self):
# Calling show_details function of parent class
super().show_details()
print(f"The contract term of the Employee is : {self.term}")
emp = ContractEmployees("John", "2k1023", "Tech Asst.", "6 Months")
# The method from the child class will be called
emp.show_details()
# Output
# The name of the Employee is : John
# The id of the Employee is : 2k1023
# The desig of the Employee is : Tech Asst.
# The contract term of the Employee is : 6 Months
Inheriting from Multiple classes
- In Python a class can inherit from multiple classes.
- To achieve this we specify the super-class names separated by commas within parentheses while defining the child class.
- Code Sample :
class Employee:
# Code for super-class Employee
class Contract:
# Code for super-class Contract
class ContractEmployees(Employee, Contract):
# Code for sub-class ContractEmployees
Types of inheritance in Python
In Python we have different types of inheritance :
Prev. Tutorial : Polymorphism
Next Tutorial : Single inheritance in Python