Beginner Level
Intermediate Level
Advanced Level
Introduction
A set is an unordered collection of unique elements in Python, and one of its main features is the ability to perform various operations on them. One such operation is the Union operation, which combines two or more sets into a new set that contains all the elements. This is a very useful operation, especially when working with data in a data science or web development context. In this tutorial, we will discuss the various ways to perform the Union operation on sets in Python using different techniques and sample code snippets. So, let's get started!
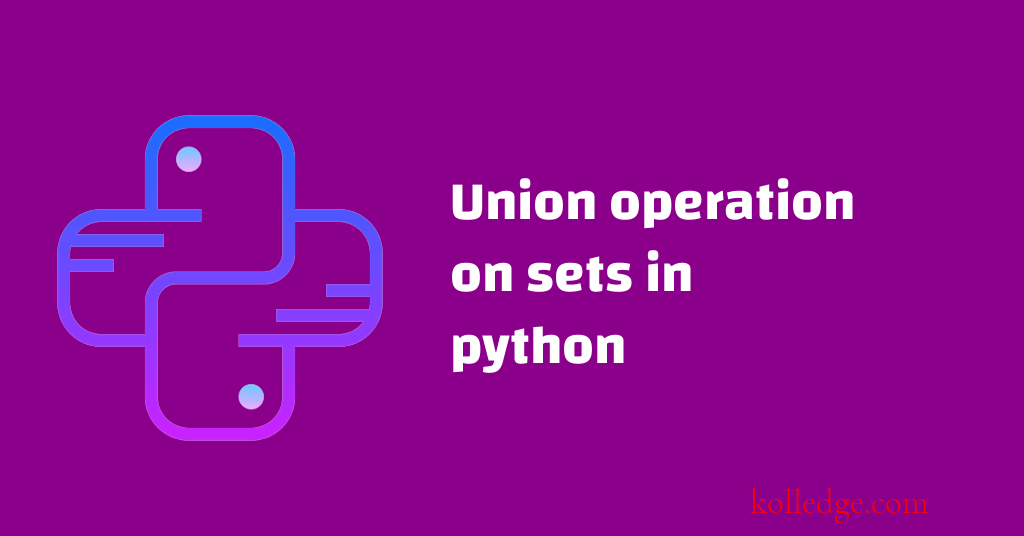
Table of Contents :
- Union operation
- Union operation using the union method
- Union operation using set union operator
Union operation :
- Union operation of two or more sets in Python returns a new set which contains all distinct elements from all the sets.
- We can union sets in Python using the :
union()
method- set union operator
|
Union operation using the union method :
- The built-in
union()
method can be used to union two or more sets in Python. - The basic syntax of using a union() method is :
new_set = set_1.union(set_2, set_3,set_4)
- The union method can also accept iterable objects as arguments.
- The union method converts the iterable object into a set before applying the union operation.
- Code Sample :
set_1 = {1, 2, 3, 4}
set_2 = {2, 4, 5, 6, 7}
union_set = set_1.union(set_2)
print(f"Value of set_1 = {set_1}")
print(f"Value of set_2 = {set_2}")
print(f"Value of union_set = {union_set}")
# Output
# Value of set_1 = {1, 2, 3, 4}
# Value of set_2 = {2, 4, 5, 6, 7}
# Value of union_set = {1, 2, 3, 4, 5, 6, 7}
Union operation using set union operator :
- The set union operator
|
can be used in python to union two sets. - The basic syntax of using a set union operator is :
new_set = set_1 | set_2
- The set union operator
|
allows only sets as operands. - We cannot union iterable objects with set union operator
|
. - Code Sample :
set_1 = {1, 2, 3, 4}
set_2 = {2, 4, 5, 6, 7}
union_set = set_1 | set_2
print(f"Value of set_1 = {set_1}")
print(f"Value of set_2 = {set_2}")
print(f"Value of union_set = {union_set}")
# Output
# Value of set_1 = {1, 2, 3, 4}
# Value of set_2 = {2, 4, 5, 6, 7}
# Value of union_set = {1, 2, 3, 4, 5, 6, 7}
Prev. Tutorial : Set operations
Next Tutorial : Intersection operation on sets