Introduction
In the world of programming, the ability to work with various types of data is essential. In Python, a float data type is used to represent numbers with decimal points. Understanding the float data type is an important part of working with mathematical operations and calculations in Python. In this tutorial, we will explore the float data type in Python, understand its characteristics, how to use it in programming, and various operations and functions available to work with it. By the end of this tutorial, you will have a firm grasp of the float data type in Python and be able to use it confidently in your programming projects. Let's get started!
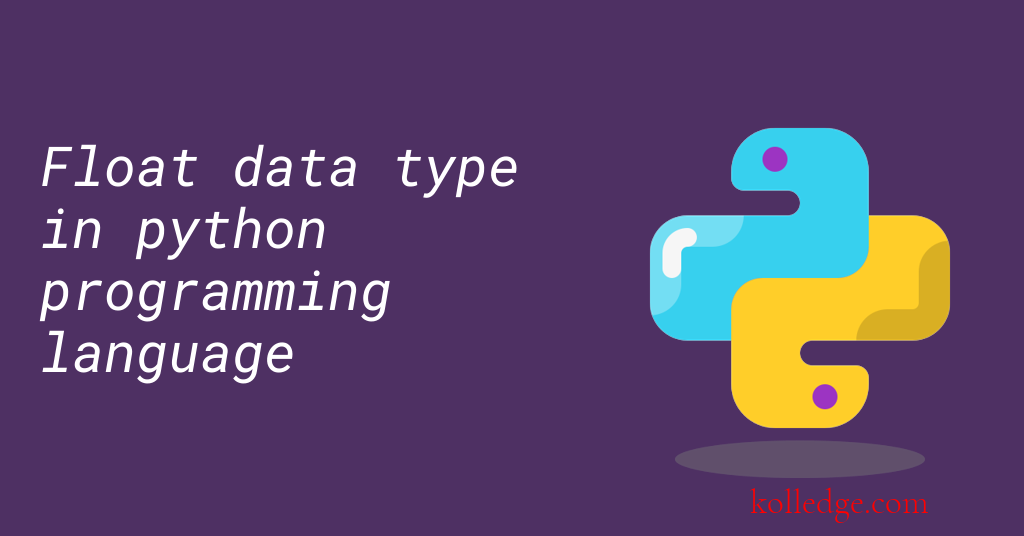
Table of Contents :
- Float data type in Python
- Creating a float variable
- Creating an instance of float class
- Float numbers in exponential form
Float data type in Python
- Float data type is used to represent decimal values (floating-point values).
- A simple use case of using the float data type is saving the price of an item in a float variable.
- The float data type is implemented using a
float
class in Python. - A float variable can be created in two ways in Python
- Assigning a float value to the variable
- Creating instance of float() class.
- Floating-point values can also be represented in scientific notation i.e. using the exponential form.
- Large floating point values can be represented in less memory by using exponential form.
Creating a float variable
- A float variable can be created simply by assigning a
float
value to a variable. - Code Sample :
pi = 3.14
print("pi is a float number.")
print(f"Value of pi = {pi}")
print(f"Type of pi is {type(pi)}")
print()
# Output
# pi is a float number.
# Value of pi = 3.14
# Type of pi is <class 'float'>
Creating an instance of float class
- A float variable can be created simply by creating instance of
float
class. - Code Sample :
pi = float(3.14)
print("pi is a float number.")
print(f"Value of pi = {pi}")
print(f"Type of pi is {type(pi)}")
print()
# Output
# pi is a float number.
# Value of pi = 3.14
# Type of pi is <class 'float'>
Float numbers in exponential form
- In Python scientific notation or exponential form of 7.62 * 10 -6 can be written as 7.62e-6. Where e is the exponent symbol.
- Code Sample :
f = 1.900000e+01
print("f is a float number.")
print(f"Value of f = {f}")
print(f"Type of f is {type(f)}")
# Output
# f is a float number.
# Value of f = 19.0
# Type of f is <class 'float'>
Prev. Tutorial : Integer Data type
Next Tutorial : Complex Data Type