Introduction
One of the most commonly used functionalities of Python is file and directory management. In this tutorial, we will learn how to copy files and directories in Python using built-in functions. Copying files and directories is an essential task for any developer or system administrator who needs to transfer data from one location to another. This tutorial will provide a comprehensive guide on the syntax, methods, and examples of copying files and directories in Python. Whether you are a beginner or an experienced programmer, this tutorial will help you develop skills in managing files and directories in Python.
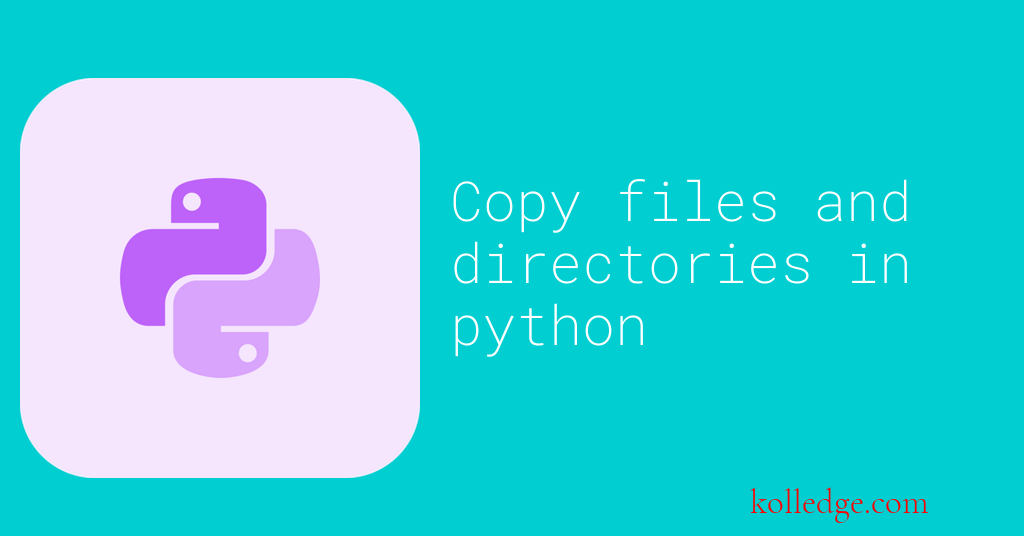
Table of Contents :
- Different methods to copy files from a directory
- The shutil.copyfile() method
- The shutil.copy() method
- The shutil.copy2() method
- The shutil.copyfileobj() method
- The os.popen() method
- The os.system() method
- The subprocess.call() method
- The subprocess.check_output() method
- How to copy all files from a directory
- How to copy entire directory in python
Different methods to copy files from a directory
The shutil.copyfile() method
- Use the
shutil
module'scopyfile()
method to copy a file from a source path to a destination path. - Code Sample :
import shutil
source_file = "/path/to/source/file"
destination_file = "/path/to/destination/file"
shutil.copyfile(source_file, destination_file)
The shutil.copy() method
- Use the
shutil
module'scopy()
method to copy a file from a source path to a destination path, preserving the metadata of the source file. - Code Sample :
import shutil
source_file = "/path/to/source/file"
destination_file = "/path/to/destination/file"
shutil.copy(source_file, destination_file)
The shutil.copy2() method
- Use the
shutil
module'scopy2()
method to copy a file from a source path to a destination path, preserving the metadata of the source file. - Use the
shutil
module'scopy()
method to copy a file from a source path to a destination path, preserving the metadata of the source file. - Use the
shutil
module'scopy2()
method to also preserve the file's metadata such as timestamps and permissions. - Code Sample :
import shutil
source_file = "/path/to/source/file"
destination_file = "/path/to/destination/file"
shutil.copy2(source_file, destination_file)
The shutil.copyfileobj() method
- Use the
shutil
module'scopyfileobj()
method to copy the contents of a file to another file, specifying the buffer size and offset. - Code Sample :
import shutil
source_file = open("/path/to/source/file", "rb")
destination_file = open("/path/to/destination/file", "wb")
shutil.copyfileobj(source_file, destination_file, length=16*1024*1024, offset=0)
source_file.close()
destination_file.close()
The os.popen() method
- Use the
os.popen()
method to copy files from a source path to a destination path. - Code Sample :
import os
source_file = "/path/to/source/file"
destination_file = "/path/to/destination/file"
os.popen("cp {0} {1}".format(source_file, destination_file))
The os.system() method
- Use the
os.system()
method to copy files from a source path to a destination path. - Code Sample :
import os
source_file = "/path/to/source/file"
destination_file = "/path/to/destination/file"
os.system("cp {0} {1}".format(source_file, destination_file))
The subprocess.call() method
- Use the
subprocess.call()
method to copy files from a source path to a destination path, specifying the shell command to be executed. - Code Sample :
import subprocess
source_file = "/path/to/source/file"
destination_file = "/path/to/destination/file"
subprocess.call(['cp', source_file, destination_file])
The subprocess.check_output() method
- Use the
subprocess.check_output()
method to copy files from a source path to a destination path, specifying the shell command to be executed. - Code Sample :
import subprocess
source_file = "/path/to/source/file"
destination_file = "/path/to/destination/file"
subprocess.check_output(['cp', source_file, destination_file])
How to copy all files from a directory
- Use the
shutil
module'scopy()
method to copy all files from a directory to another directory. - Code Sample :
import os
import shutil
source_directory = "/path/to/source_directory"
destination_directory = "/path/to/destination_directory"
for file_name in os.listdir(source_directory):
source = os.path.join(source_directory, file_name)
destination = os.path.join(destination_directory, file_name)
shutil.copy(source, destination)
How to copy entire directory in python
- Use the
shutil
module'scopytree()
method to copy an entire directory to another directory. - Code Sample :
import shutil
source_directory = "/path/to/source_directory"
destination_directory = "/path/to/destination_directory"
shutil.copytree(source_directory, destination_directory)
Prev. Tutorial : Delete Files and Directories
Next Tutorial : Move Files and Directories