Beginner Level
Intermediate Level
Advanced Level
Introduction
Objects are the fundamental building blocks of object oriented programming, and understanding them is crucial for any programmer. An object is a collection of data and functions that work together to perform some specific task. In other words, an object can be thought of as a self-contained entity that has its own properties and behaviors. In this tutorial , we will take a closer look at objects, understand their importance in, and learn how to create and use them in programming.
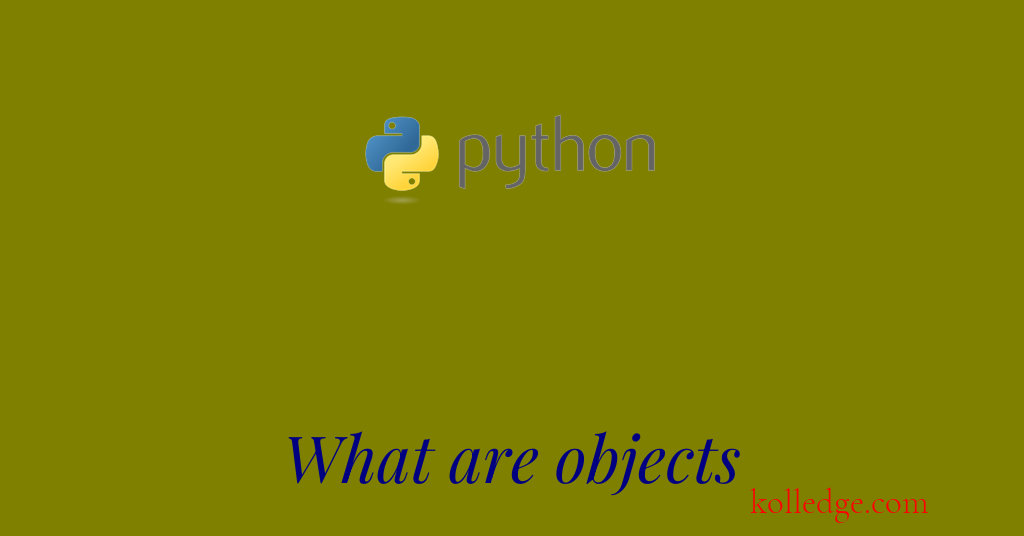
Table of Contents :
- OOPs Concepts - Objects
- Real World example of Objects
OOPs Concepts - Objects :
- An object is an instance of a class.
- It is the most basic unit of object oriented programming.
- Objects contain data and the code to process that data.
- An object has a state and behavior.
- state is defined by the data of the objects
- behavior is defined by the functions of the objects.
- Memory is allocated only when we create an object.
- In other words memory is not allocated when we define a class, but memory is allocated when we instantiate it.
- There can be many objects that belong to a single class.
- Every object of a class has a unique identity.
- Hence an object of a class has -
- state - defined by the data members of the class
- behavior - defined by the data functions of the class
- identity - every object of a class is different from other objects.
- Each object of a class contains same type of data and functions that were defined in the class.
Real World example of Objects :
In our previous example of Vehicles class that we create in the previous tutorial on Classes :
- Car can be an object.
- The value of "number of tires" for car will be 4
- The value of "top-speed" for car can be 250.
- similarly scooter can be an object of Vehicles class -
- The value of "number of tires" for scooter will be 2
- The value of "top-speed" for scooter can be 100.
Prev. Tutorial : What are classes
Next Tutorial : What is Encapsulation