Beginner Level
Intermediate Level
Advanced Level
Introduction
Sets are a powerful data structure in Python that allow us to store an unordered collection of unique elements. The Intersection operation on sets allows us to find the common elements between two or more sets. This operation is extremely useful in data analysis and various other applications where we need to find the commonality between sets. In this tutorial, we will explore the various ways in which we can perform the Intersection operation on sets in Python. So let's get started!
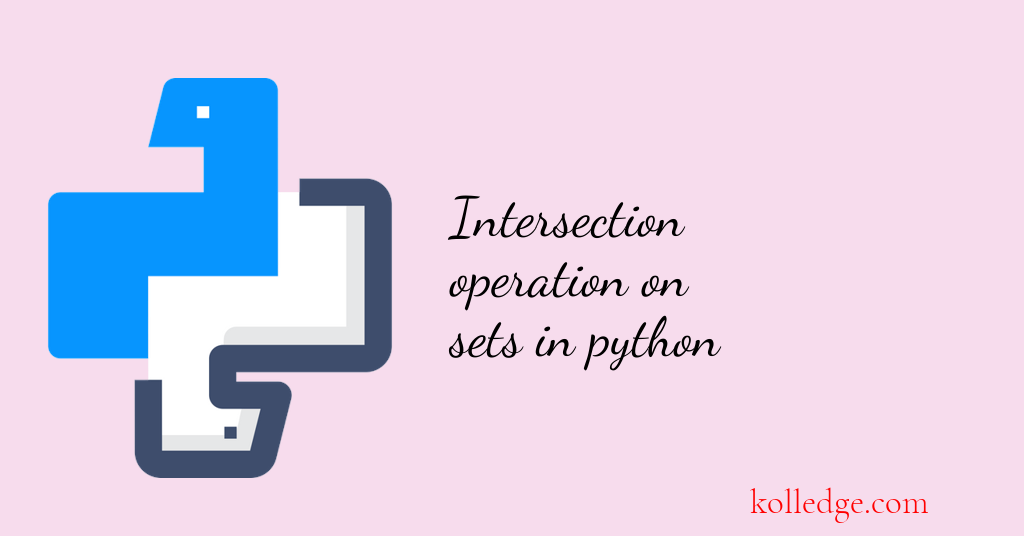
Table of Contents :
- Intersection operation
- Intersection operation using the intersection method
- Intersection operation using set intersection operator
Intersection operation :
- Intersection operation of two or more sets in Python returns a new set which contains the elements that exist in all the sets.
- We can do intersection on sets in Python using the :
- intersection() method
- set intersection operator '&'
Intersection operation using the intersection method :
- The built-in
intersection()
method can be used to intersect two or more sets in Python. - The basic syntax of using a
intersection()
method is :new_set = set_1.intersection(set_2, set_3,set_4,...)
- The
intersection()
method can also accept iterable objects as arguments. - The
intersection()
method converts the iterable object into a set before applying the intersection operation. - Code Sample :
set_1 = {1, 2, 3, 4}
set_2 = {2, 4, 5, 6, 7}
new_set = set_1.intersection(set_2)
print(f"Value of set_1 = {set_1}")
print(f"Value of set_2 = {set_2}")
print(f"Value of new_set = {new_set}")
# Output
# Value of set_1 = {1, 2, 3, 4}
# Value of set_2 = {2, 4, 5, 6, 7}
# Value of new_set = {2, 4}
Intersection operation using set intersection operator :
- The set intersection operator
&
can be used in python to intersect two sets. - The basic syntax of using a set intersection operator is :
new_set = set_1 & set_2
- The set intersection operator allows only sets as operands.
- We cannot intersect iterable objects with set intersection operator.
- Code Sample :
set_1 = {1, 2, 3, 4}
set_2 = {2, 4, 5, 6, 7}
new_set = set_1 & set_2
print(f"Value of set_1 = {set_1}")
print(f"Value of set_2 = {set_2}")
print(f"Value of new_set = {new_set}")
# Output
# Value of set_1 = {1, 2, 3, 4}
# Value of set_2 = {2, 4, 5, 6, 7}
# Value of new_set = {2, 4}
Prev. Tutorial : Union operation on sets
Next Tutorial : Difference operation on sets