Beginner Level
Intermediate Level
Advanced Level
Introduction
With Python, it is also possible to develop multithreaded programs, which can execute multiple threads simultaneously thereby achieving concurrency and higher performance. In this tutorial, we will explore how to develop multithreaded programs in Python. We will cover with the basics of multithreading in this tutorial.
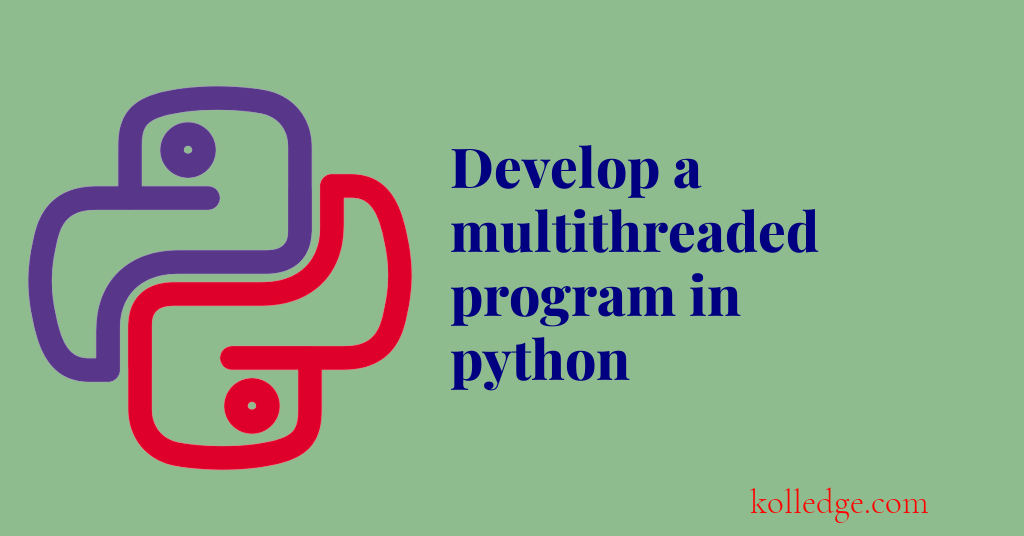
Table of Contents :
- Creating a thread class
- Using the Stock class
Creating a thread class :
- The
threading
module provides the Thread class which can be inherited to create custom threads. - To create a thread class,
- the main thread class needs to be inherited, and
- the
__init__()
andrun()
methods need to be overridden.
import threading
class CustomThread(threading.Thread):
def __init__(self, name):
threading.Thread.__init__(self)
self.name = name
def run(self):
print(f"Thread {self.name} running")
Using the Stock class :
- Threading module can be used to manage volatile variables such as stock prices.
- In a stock trading scenario, multiple threads can be created to retrieve and process stock prices.
- The following code, implements a thread class StockThread that retrieves the stock price for a given symbol:
import threading
import yfinance as yf
class StockThread(threading.Thread):
def __init__(self, symbol):
threading.Thread.__init__(self)
self.symbol = symbol
def run(self):
stock = yf.Ticker(self.symbol)
price = stock.info['regularMarketPrice']
print(f"Price of {self.symbol} is {price}")
The yfinance package is used to retrieve the stock prices of a given symbol.
Prev. Tutorial : Threading in Python
Next Tutorial : Threading Event