Introduction
Strings are a fundamental data type in Python language, and understanding how to manipulate them is a critical skill for anyone learning the language. This tutorial will guide you on different string operations in Python. You will learn how to use different string operators and string methods to perform various operations such as concatenation, slicing, length measurements, Strip operations, and much more. With an in-depth understanding of strings, you can effectively use Python to manipulate and parse data efficiently. Whether you are a beginner or an experienced programmer, this tutorial is an essential guide to perfecting your string manipulation skills in Python.
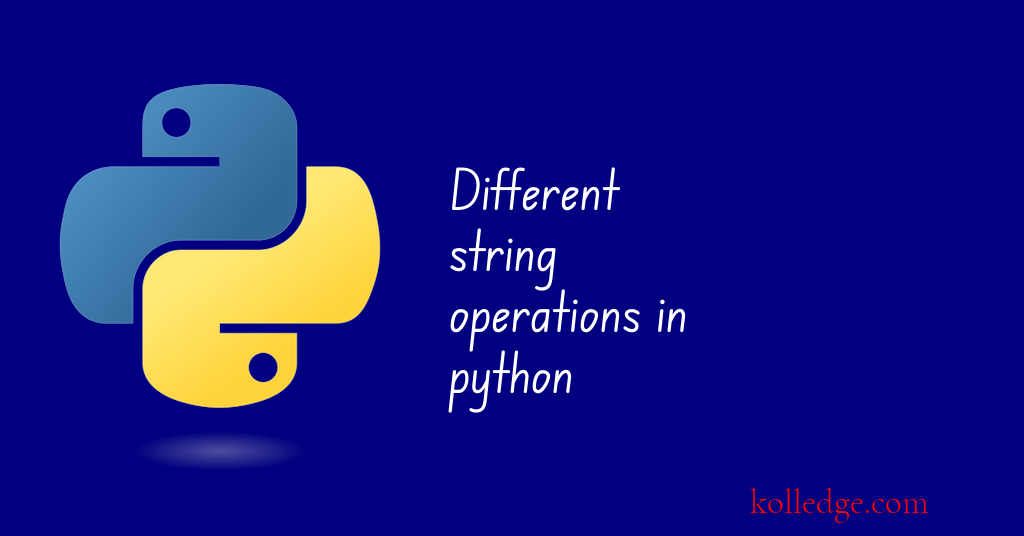
Table of Contents :
- String Operations in Python :
- String Concatenation
- String Repetition
- String Slicing
- Find String Length
- String Case Conversion
- String Strip
- String Replace
- String Split
- String Join
- String Formatting
String Operations in Python :
- Different String operations possible in Python are :
- String Concatenation
- String Repetition
- String Slicing
- Find String Length
- String Case Conversion
- String Strip
- String Replace
- String Split
- String Join
- String Formatting
String Concatenation
- The concatenation operator (+) is used to concatenate strings in python.
- Code Sample :
string1 = "Hello"
string2 = "world"
result = string1 + " " + string2
print(result)
# Output
# Hello world
String Repetition
- The repetition operator (*) is used to repeat a string a certain number of times.
- Code Sample :
string = "Hello-"
result = string * 3
print(result)
# Output
# Hello-Hello-Hello-
String Slicing
- The slicing operator (:) is used to extract a part of a string.
- Code Sample :
string = "Hello world"
result = string[0:5]
print(result)
# Output
# Hello
String Length
- The
len()
function is used to get the length of a string. - Code Sample :
string = "Hello world"
result = len(string)
print(result)
# Output
# 11
String Case Conversion
- The
upper()
method is used to convert a string to uppercase. - The
lower()
method is used to convert a string to lowercase. - Code Sample :
string = "Hello world"
result1 = string.upper()
result2 = string.lower()
print(result1)
print(result2)
# Output:
# HELLO WORLD
# hello world
String Strip
- The
strip()
method is used to remove leading and trailing white spaces from a string. - Code Sample :
string = " Hello world "
result = string.strip()
print(result)
# Output
# Hello world
String Replace
- The
replace()
method is used to replace a sub-string with another sub-string. - Code Sample :
string = "Hello world"
result = string.replace("world", "Kolledge Scholars")
print(result)
# Output
# Hello Kolledge Scholars
String Split
- The
split()
method is used to split a string into a list of sub-strings based on a delimiter. - Code Sample :
string = "Hello,world"
result = string.split(",")
print(result)
# Output
# ['Hello', 'world']
String Join
- The
join()
method is used to join a list of strings into a single string using a delimiter. - Code Sample :
list = ['Hello', 'world']
delimiter = ","
result = delimiter.join(list)
print(result)
# Output
# Hello,world
String Formatting
- String formatting is used to create a string with dynamic content.
- The
format()
method is used to insert values into placeholders in a string. - Code Sample :
name = "John"
age = 30
result = "My name is {} and I am {} years old".format(name, age)
print(result)
# Output
# My name is John and I am 30 years old
Prev. Tutorial : String operators
Next Tutorial : Accessing characters of string