Beginner Level
Intermediate Level
Advanced Level
Introduction
Asyncio is a Python package that provides an essential way to write concurrent code using coroutines. In this tutorial, we will dive deep into the Asyncio module and take in-depth look at the gather()
function. This function is an essential component of concurrent programming in Python and is used to execute multiple async functions concurrently and wait for all of them to finish. So, let's get started and learn more about the Asyncio.gather() function in Python.
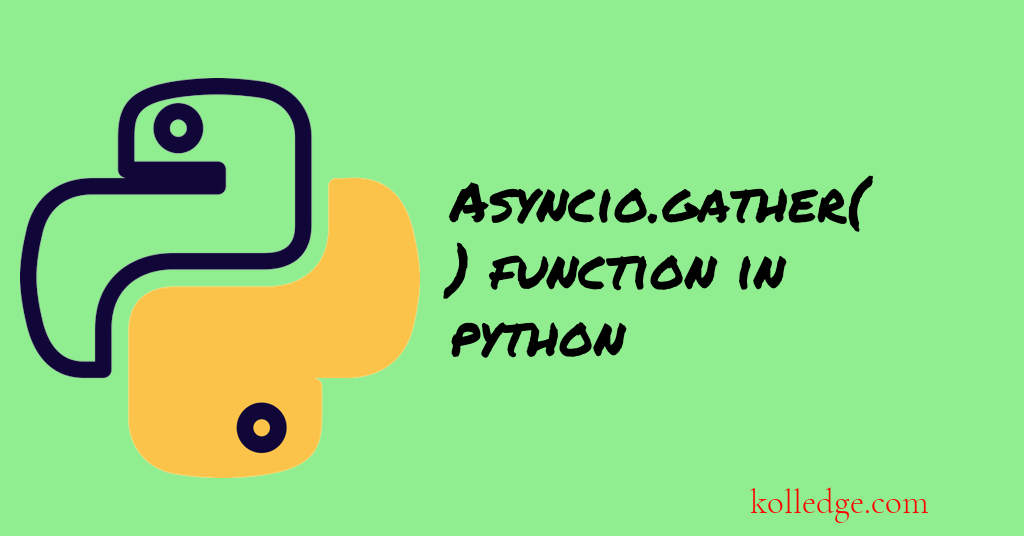
Table of Contents :
- Python asyncio.gather() Function
- How to use asyncio.gather() to Run Multiple Asynchronous Operations
- Using asyncio.gather() to Run Multiple Asynchronous Operations with Exceptions
- Returning an Exception in the Result Using asyncio.gather()
Python asyncio.gather() Function :
- The
asyncio.gather()
function is used to execute multiple co-routines concurrently and wait for their results. - The function takes one or more co-routine objects as input, and returns a future object that represents the result of all co-routines.
- We can use await with the future object to retrieve the results of all co-routines once they are complete.
How to use asyncio.gather() to Run Multiple Asynchronous Operations :
- Here's an example of using
asyncio.gather()
function to run multiple co-routines concurrently and waiting for their result: - Code Sample :
import asyncio
async def coro_1():
await asyncio.sleep(2)
return 'Result of coro 1'
async def coro_2():
await asyncio.sleep(1)
return 'Result of coro 2'
async def main():
results = await asyncio.gather(coro_1(), coro_2())
print('Results:', results)
asyncio.run(main())
Explanation :
- In the above example, we define two co-routines
coro_1()
andcoro_2()
that wait for two seconds and one second before returning respectively. - We use
asyncio.gather()
function to execute both co-routines concurrently. - When both co-routines complete, their results are gathered into a list, which is printed to the console.
Using asyncio.gather() to Run Multiple Asynchronous Operations with Exceptions :
- Here's an example of using
asyncio.gather()
function to run multiple co-routines concurrently and handling any exceptions that occur: - Code Sample :
import asyncio
async def coro_1():
await asyncio.sleep(2)
return 'Result of coro 1'
async def coro_2():
await asyncio.sleep(1)
raise ValueError('An error occurred in coro 2')
async def main():
try:
results = await asyncio.gather(coro_1(), coro_2(), return_exceptions=True)
except ValueError as exc:
print('Caught an exception:', exc)
else:
print('Results:', results)
asyncio.run(main())
Explanation :
- In the above example, we define two coroutines
coro_1()
andcoro_2()
that wait for two seconds and one second before returning respectively. - We use
asyncio.gather()
function to execute both co-routines concurrently, butcoro_2()
raises aValueError
. - We use
return_exceptions=True
to instructasyncio.gather()
function to return the exception object instead of raising it. - When an exception occurs, it is caught by the try/except block, and its message is printed to the console.
Returning an Exception in the Result Using asyncio.gather() :
- Here's an example of using
asyncio.gather()
function to return both the results and exceptions as a list: - Code Sample :
import asyncio
async def coro_1():
await asyncio.sleep(2)
return 'Result of coro 1'
async def coro_2():
await asyncio.sleep(1)
raise ValueError('An error occurred in coro 2')
async def main():
results = await asyncio.gather(coro_1(), coro_2(), return_exceptions=False)
print('Results:', results)
asyncio.run(main())
Explanation :
- In the above example, we define two co-routines
coro_1()
andcoro_2()
that wait for two seconds and one second before returning respectively. - We use
asyncio.gather()
function to execute both co-routines concurrently, butcoro_2()
raises aValueError
. - We use
return_exceptions=False
to instructasyncio.gather()
function to raise the exception instead of returning it. - When an exception occurs, it is raised and caught by
asyncio.gather()
function, and the program terminates with a stack trace.
Prev. Tutorial : Future object
Next Tutorial : Regular expressions