Introduction
Python is a popular programming language among developers, especially when it comes to automation, web development, and data science. One of the reasons for this popularity is the simplicity and flexibility of the language. Python provides a variety of string operators that are used to manipulate and analyze strings in the program. String operators are essential in performing various string operations, and mastering them is crucial for efficient programming. This tutorial will cover the essential string operators in Python and provide examples to help you learn and understand how to use them in your Python programs.
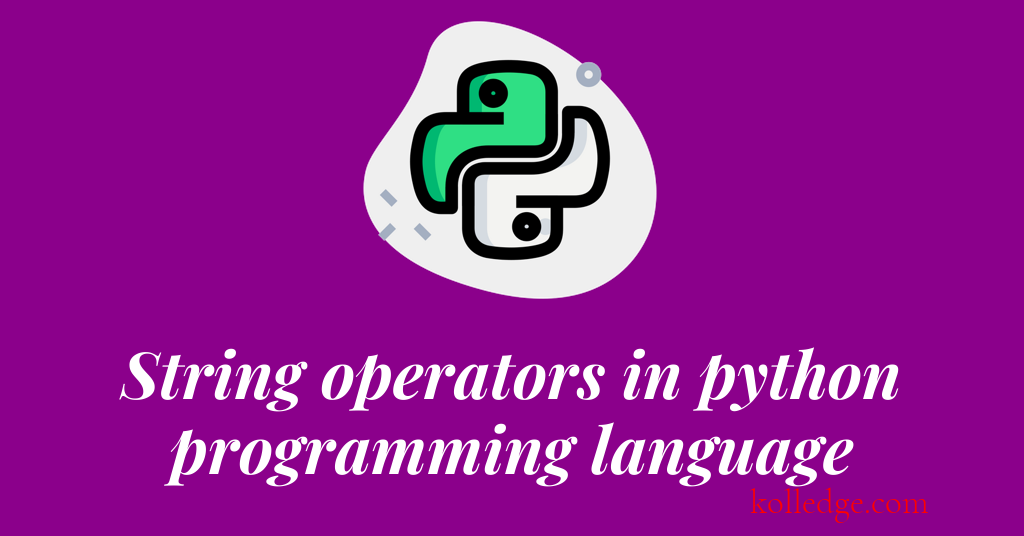
Table of Contents :
- Python string operators
- Assignment Operator for Strings in Python
- Concatenate Operator for Strings in Python
- Repetition Operator for Strings in Python
- Slicing Operator for Strings in Python
- Comparison Operator for Strings in Python
- Membership Operator for Strings in Python
- Escape Sequence Operator for Strings in Python
- Formatting Operator for Strings in Python
Python string operators :
- String operators are used to perform various operations on strings in Python.
- Python supports several string operators, which allow you to perform tasks such as string concatenation, repetition, string slicing, and comparison.
- String operators can be used to manipulate string data, which is essential for many programming tasks.
- Different string operators in Python are :
- Assignment Operator
- Concatenate Operator
- Repetition Operator
- Slicing Operator
- Comparison Operator
- Membership Operator
- Escape Sequence Operator
- Formatting Operator
Assignment Operator for Strings in Python :
- The assignment operator (=) assigns a value to a string variable.
- This operator allows you to create a new string or overwrite an existing string with a new value.
- Code Sample :
message = "Hello, World!"
Concatenate Operator for Strings in Python:
- The concatenation operator (+) combines two or more strings into a single string.
- This operator is useful when you need to combine multiple strings to create a new string value.
- Code Sample :
greeting = "Hello"
name = " John"
message = greeting + " " + name
print(message)
# Output
# Hello John
Repetition Operator for Strings in Python:
- The repetition operator (*) repeats a string a specified number of times.
- This operator allows you to create a new string by repeating an existing string multiple times.
- Code Sample :
word = "hello"
repeat_word = word * 5
print(repeat_word)
# Output
# hello_hello_hello_hello_hello_
Slicing Operator for Strings in Python:
- The slicing operator (:) extracts a portion of a string.
- This operator allows you to select a sub-string from a larger string based on its starting and ending indices.
- The syntax for using the slicing operator is
[start:end]
, wherestart
is the index of the first character to include andend
is the index of the last character to exclude.
- Code Sample :
word = "hello"
substring = word[0:3]
print(substring)
# Output
# hel
Comparison Operator for Strings in Python:
- The comparison operator (==) compares two strings to see if they are equal.
- This operator returns a Boolean value
True or False
depending on whether the strings are equal. - Other comparison operators can also be used to compare strings, such as
- not equal
!=
- less than
<
, - greater than
>
, - less than or equal to
<=
and - greater than or equal to
>=
- not equal
- Code Sample :
word1 = "hello"
word2 = "world"
word3 = "hello"
result = word1 == word2
result2 = word3 == word1
print(result)
print(result2)
# Output
# False
# True
Membership Operator for Strings in Python:
- The membership operators
in
andnot in
test whether a string contains a specified sub-string. - The
in
operator returns True if the sub-string is found in the string, - The
not in
operator returns True if the sub-string is not found in the string. - Code Sample :
word = "hello"
result1 = "ell" in word
result2 = "ell" not in word
print(result1)
print(result2)
# Output
# True
# False
result1 = "foo" in word
result2 = "foo" not in word
print(result1)
print(result2)
# Output
# False
# True
Escape Sequence Operator for Strings in Python:
- The escape sequence operator (\) allows you to include special characters in a string.
- These special characters are used to represent newline, tab, and other non-printable characters that cannot be typed directly into a string.
- For example,
\n
represents a newline character,\t
represents a tab character, and\r
represents a carriage return character.
- We have a detailed tutorial on escape sequencing in python.
- Code Sample :
message = "Hello\nWorld"
print(message)
# Output
# Hello
# World
Formatting Operator for Strings in Python:
- The formatting operator (%) allows you to create formatted strings by replacing placeholders with values.
- Placeholders aka conversion specifiers are indicated by
%s
(for strings),%d
(for integers), and other similar symbols. - A complete list of conversion specifiers can be found here .
- They are replaced with actual values when the string is formatted.
- This operator is useful when we need to create a string that includes dynamic or variable data.
- Code Sample :
name = "John"
age = 30
message = "My name is %s and I am %d years old." % (name, age)
print(message)
# Output
# My name is John and I am 30 years old.
Prev. Tutorial : Set comprehension
Next Tutorial : Different String operations