Beginner Level
Intermediate Level
Advanced Level
Introduction
One of the most powerful features of Python is its ability to automate repetitive tasks and streamline workflows. In this tutorial, we'll explore how to create tasks in Python, which refers to automating processes that require certain actions to be taken on a repetitive basis. By learning how to create and manage tasks, you'll be able to reduce manual workload and increase efficiency, allowing more time for important tasks and projects. This tutorial is suitable for programmers of all levels who want to learn how to automate repetitive tasks using Python.
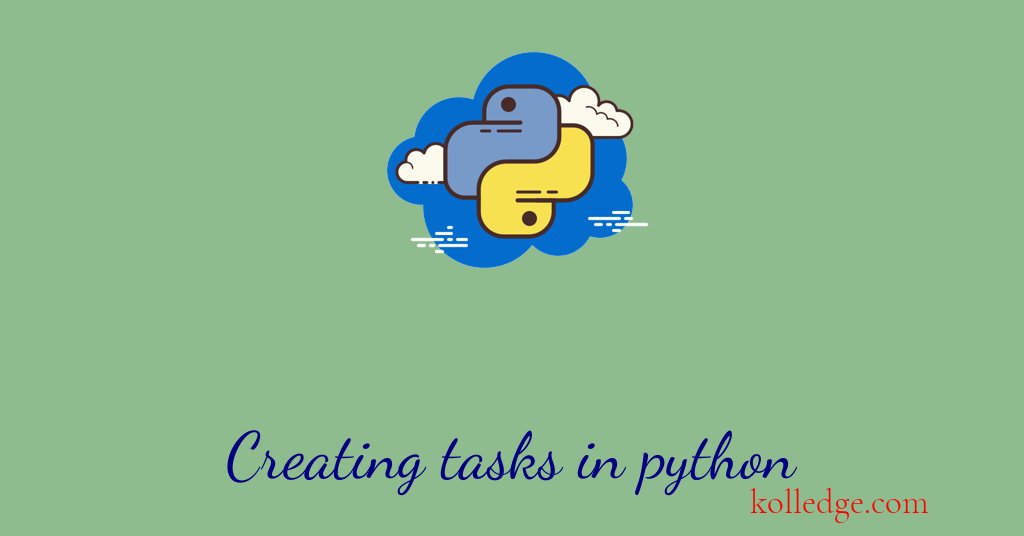
Table of Contents :
- Python Tasks
- Simulating a Long-Running Operation
- Running Other Tasks While Waiting
Python Tasks :
- A Python task is a type of co-routine object that represents a co-routine that has been scheduled to run on the event loop.
- Tasks are useful for running long-running operations and can be used to monitor the status of the operation and retrieve its result.
Simulating a Long-Running Operation :
- In order to demonstrate the use of
asyncio.create_task()
, we need a co-routine that simulates a long-running operation. - Here's an example of a co-routine that waits for three seconds before returning:
- Code Sample :
async def long_operation():
print('Long operation started')
await asyncio.sleep(3)
print('Long operation completed')
return 'Long operation result'
Running Other Tasks While Waiting :
- One of the benefits of using tasks is that we can run other tasks while waiting for a long-running operation to complete.
- Here's an example of using
asyncio.create_task()
to create a task for our long-running operation, and then running other tasks while waiting for it to complete: - Code Sample :
import asyncio
async def long_operation():
print('Long operation started')
await asyncio.sleep(3)
print('Long operation completed')
return 'Long operation result'
async def task1():
print('Task 1 started')
await asyncio.sleep(1)
print('Task 1 completed')
return 'Task 1 result'
async def task2():
print('Task 2 started')
await asyncio.sleep(2)
print('Task 2 completed')
return 'Task 2 result'
async def main():
print('Main started')
long_op_task = asyncio.create_task(long_operation())
task1_task = asyncio.create_task(task1())
task2_task = asyncio.create_task(task2())
await long_op_task
print('Long operation result:', long_op_task.result())
await task1_task
print('Task 1 result:', task1_task.result())
await task2_task
print('Task 2 result:', task2_task.result())
print('Main completed')
asyncio.run(main())
Explanation :
- In the above example, we define three co-routines,
long_operation()
,task1()
, andtask2()
. - We use
asyncio.create_task()
to create tasks for all three co-routines. - We then run these tasks concurrently using await statements.
- The result of each task is retrieved using the
result()
method of the task object. - When we run this program, it will execute the
long_operation()
co-routine in the background, while runningtask1()
andtask2()
concurrently. - Once
long_operation()
is complete, it will print its result and the results oftask1()
andtask2()
.
Prev. Tutorial : async-await
Next Tutorial : Canceling tasks