Beginner Level
Intermediate Level
Advanced Level
Introduction
Unpacking a list involves splitting the elements of a list into individual variables. This is an extremely useful technique in Python, especially when we want to work with multiple elements of a list at the same time. With unpacking, we can easily assign values to variables, swap the values of variables, and iterate over multiple variables in a loop. In this tutorial, we will explore how to unpack a list in Python.
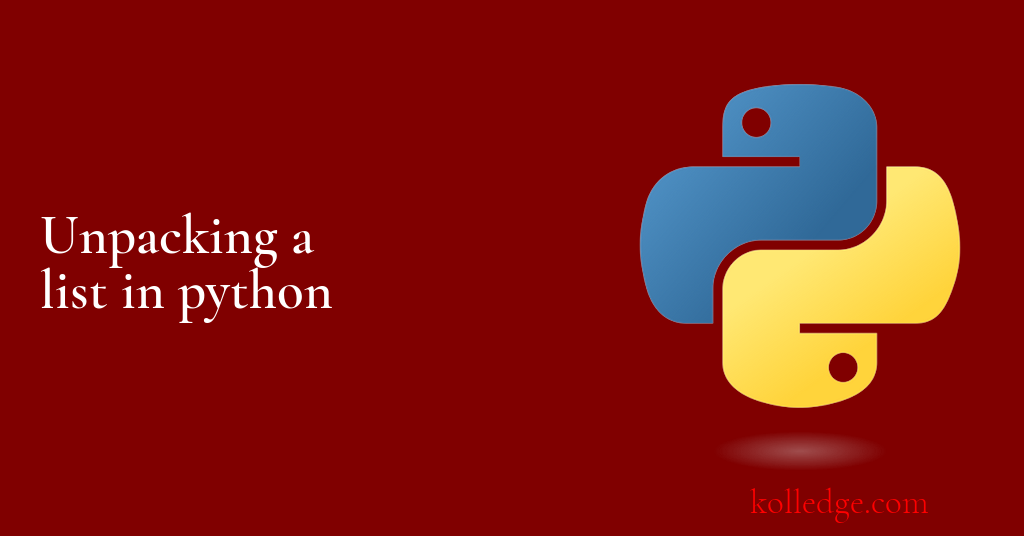
Table of Contents :
- Unpacking a list
- Unpacking - packing
Unpacking a list :
- Python provides a shortcut method of assigning all the elements of a list to individual variables at once.
- In conventional method to assign elements of the list to individual variables we will have to access all elements of list as follows :
var_1 = list_name[0]
var_2 = list_name[1]
var_3 = list_name[2]
- The list unpacking method simplifies this cumbersome process to great extent.
- The syntax for list unpacking is :
var_1, var_2, var_3 = list_name
- The unpacking process can be performed with tuples as well :
var_1, var_2, var_3 = tuple_name
- The number of variables should be equal to the number of elements in the list.
- If the number of variables is less than the number of elements of the list, python interpreter will raise an error :
ValueError: too many values to unpack
- If the number of variables is more than the number of elements of the list, python interpreter will raise an error :
ValueError: not enough values to unpack
- Code Sample :
my_list = [10, 20, 30]
print("my_list = ", my_list)
var_1, var_2, var_3 = my_list
print("var_1 = ", var_1)
print("var_2 = ", var_2)
print("var_3 = ", var_3)
# Output
# my_list = [10, 20, 30]
# var_1 = 10
# var_2 = 20
# var_3 = 30
Unpacking - packing :
- There is another syntax in python in which we can
- assign the first few elements of the list to variables and
- save the rest as a slice of the list.
- We use an asterisk for this purpose.
- The syntax for list unpacking-packing is as follows :
var_1, var_2, *var_3 = list_name
# Here :
# the first element of the list is assigned to var_1
# the second element of the list is assigned to var_2
# the rest of the elements are packed as a new list and assigned to var_3
- In this process, the python interpreter :
- first unpacks the original list
- assigns the matching number of elements to variables.
- packs the remaining elements to a new list and assign it to the variable with asterisk.
- We can have only one variable with asterisk in this syntax.
- If we try to put asterisk on more than one variable, python interpreter will raise an error :
SyntaxError: multiple starred expressions in assignment
- We can put asterisk on any of the variable.
- Code Sample :
my_list = [10, 20, 30, 40, 50, 60]
print("my_list = ", my_list)
var_1, var_2, *var_3 = my_list
print("var_1 = ", var_1)
print("var_2 = ", var_2)
print("var_3 = ", var_3)
# Output
# my_list = [10, 20, 30, 40, 50, 60]
# var_1 = 10
# var_2 = 20
# var_3 = [30, 40, 50, 60]
Prev. Tutorial : Slicing a list
Next Tutorial : Tuples