Beginner Level
Intermediate Level
Advanced Level
Introduction
Python programming language provides many built-in methods to manipulate strings. String methods are the set of operations that can be performed on a string object to achieve various outcomes like changing the case of letters, adding or removing white spaces, finding a sub-string and many more. Therefore, a good understanding of string methods is essential for any Python programmer who wants to work with strings. In this tutorial, we will explore the most commonly used string methods in Python and learn how to use them effectively in our programs.
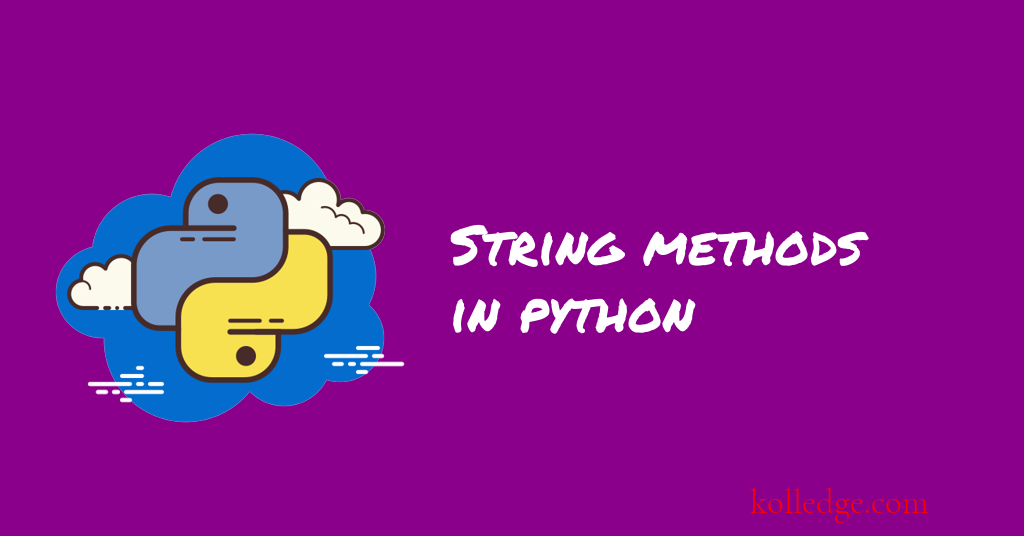
Table of Contents :
- String Methods
- capitalize()
- center(width[, fillchar])
- count(sub[, start[, end]])
- encode([encoding[,errors]])
- endswith(suffix[, start[, end]])
- find(sub[, start[, end]])
- index(sub[, start[, end]])
- isalnum()
- isalpha()
- isdigit()
- islower()
- isupper()
- join(iterable)
- lower()
- upper()
String Methods :
Some of the string methods that we'll discuss in this tutorial are :
- capitalize()
- center(width[, fillchar])
- count(sub[, start[, end]])
- encode([encoding[,errors]])
- endswith(suffix[, start[, end]])
- find(sub[, start[, end]])
- index(sub[, start[, end]])
- isalnum()
- isalpha()
- isdigit()
- islower()
- isupper()
- join(iterable)
- lower()
- upper()
capitalize()
- The
capitalize()
method capitalizes the first character of a string. - Code Sample :
text = "hello world"
new_text = text.capitalize()
print(new_text)
# Output
# Hello world
center(width[, fillchar])
- Returns a string centered in a string of specified width.
- An optional fill character can be specified.
- Code Sample :
text = "hello"
new_text = text.center(10)
print(new_text)
# Output
# hello
count(sub[, start[, end]])
- Counts the number of occurrences of a sub-string within a string.
- Optional start and end indices can be specified.
- Code Sample :
text = "hello world"
count = text.count("o")
print(count)
# Output
# 2
encode([encoding[,errors]])
- Returns a byte string representing the encoded version of a string.
- Code Sample :
text = "hello world"
encoded = text.encode()
print(encoded)
# Output
# b'hello world'
endswith(suffix[, start[, end]])
- Checks if a string ends with a specified suffix.
- Optional start and end indices can be specified.
- Code Sample :
text = "hello world"
endswith_world = text.endswith("world")
print(endswith_world)
# Output
# True
find(sub[, start[, end]])
- Searches a string for the first occurrence of a sub-string and returns the index.
- Returns -1 if the sub-string is not found.
- Optional start and end indices can be specified.
- Code Sample :
text = "hello world"
index = text.find("world")
print(index)
# Output
# 6
index(sub[, start[, end]])
- Same as the find() method, but raises a ValueError if the sub-string is not found.
- The find method returns -1 if sub-string is not found.
- Code Sample :
text = "hello world"
pos_index = text.index("foo")
print(pos_index)
# Output
# ValueError: substring not found
isalnum()
- Returns True if all characters in a string are alphanumeric (letters or digits).
- Code Sample :
text1 = "hello"
text2 = "h3ll0"
text3 = "!@#$"
isalnum1 = text1.isalnum()
isalnum2 = text2.isalnum()
isalnum3 = text3.isalnum()
print(isalnum1)
print(isalnum2)
print(isalnum3)
# Output
# True
# True
# False
isalpha()
- Returns True if all characters in a string are alphabetic (letters).
- Code Sample :
text1 = "hello"
text2 = "h3ll0"
isalpha1 = text1.isalpha()
isalpha2 = text2.isalpha()
print(isalpha1)
print(isalpha2)
# Output
# True
# False
isdigit()
- Returns True if all characters in a string are digits.
- Code Sample :
text1 = "456"
text2 = "foo"
isdigit1 = text1.isdigit()
isdigit2 = text2.isdigit()
print(isdigit1)
print(isdigit2)
# Output
# True
# False
islower()
- Returns True if all cased characters in a string are lowercase.
- Code Sample :
text1 = "hello world"
text2 = "Hello World"
islower1 = text1.islower()
islower2 = text2.islower()
print(islower1)
print(islower2)
# Output
# True
# False
isupper()
- Returns True if all cased characters in a string are uppercase.
- Code Sample :
text1 = "HELLO WORLD"
text2 = "Hello World"
isupper1 = text1.isupper()
isupper2 = text2.isupper()
print(isupper1)
print(isupper2)
# Output
# True
# False
join(iterable)
- Joins the elements of an iterable with a string as separator.
- Code Sample :
my_list = ["apple", "banana", "cherry"]
separator = ", "
text = separator.join(my_list)
print(text)
# Output
# apple, banana, cherry
lower()
- Returns a copy of a string with all cased characters converted to lowercase.
- Code Sample :
text = "HELLO WORLD"
new_text = text.lower()
print(new_text)
# Output
# hello world
upper()
- Returns a copy of a string with all cased characters converted to uppercase.
- Code Sample :
text = "hello world"
new_text = text.upper()
print(new_text)
# Output
# HELLO WORLD
Prev. Tutorial : Formatting strings
Next Tutorial : What is OOPs