Beginner Level
Intermediate Level
Advanced Level
Introduction
When a class inherits from multiple classes, Python determines the order in which methods are executed. This order is known as the Method Resolution Order (MRO). Understanding MRO is essential for developing applications in Python and can help avoid unexpected behavior. In this Python tutorial, we will explore MRO and its importance in object-oriented programming with practical examples and explanations.
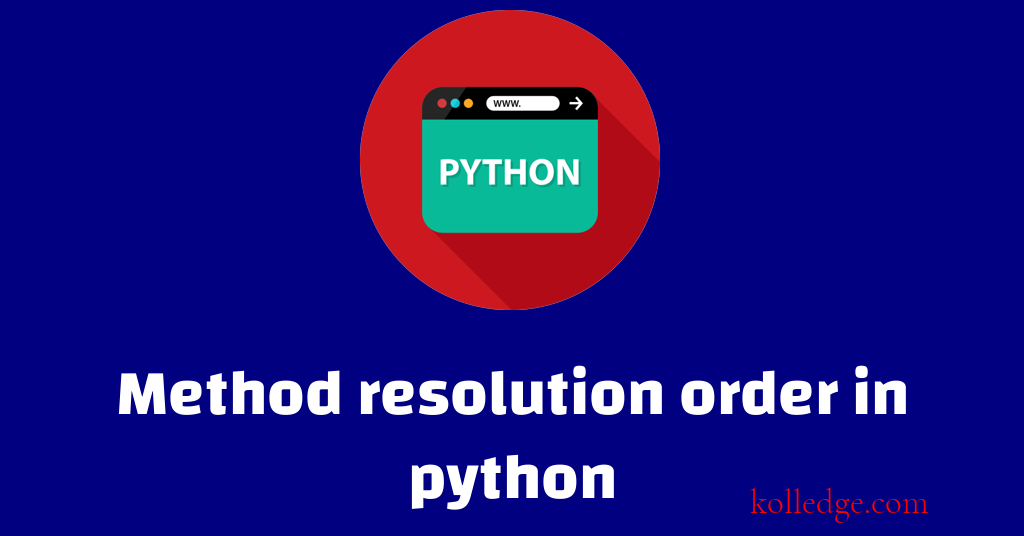
Table of Contents :
- What is Method Resolution?
- How Python Determines Method Resolution Order
- Intro to Depth-First Algorithm
What is Method Resolution?
- Method Resolution refers to the process of determining which method to invoke when a method is invoked on an object in Python.
- In Python, a class can have multiple ancestors, and each ancestor can have a method with the same name.
- When a method is called on an object, Python searches for the method in a specific order to determine which method to invoke.
How Python Determines Method Resolution Order
- When a method is called on an object, Python follows a specific order to determine which method to invoke.
- The order in which Python searches for a method is called the Method Resolution Order (MRO).
- Python's MRO algorithm is called the C3 algorithm.
- The C3 algorithm creates a linear ordering of the class hierarchy to determine the MRO.
Intro to Depth-First Algorithm
- The C3 algorithm is a depth-first algorithm that merges the MRO of the parents in a specific way to determine the MRO of a child.
- The C3 algorithm works as follows:
- The algorithm starts with the first class in the list of parents and adds it to the MRO of the child.
- If the first class has any parents, the algorithm recursively calls itself with the list of parents as the argument.
- The algorithm takes the first class from the MRO of the first parent and adds it to the MRO of the child if it is not already in the MRO.
- If the first class from the MRO of the first parent is already in the MRO, it is skipped and the algorithm continues with the next class in the MRO of the first parent.
- Once all the parents have been processed, the algorithm checks that the MRO does not violate the "local precedence ordering" of the classes. If it does, the algorithm fails, and Python raises a TypeError.
Example:
class A:
pass
class B:
pass
class C(A, B):
pass
class D(B, A):
pass
class E(C, D):
pass
print(E.mro())
# Output: [E, C, A, D, B, object]
The MRO of class `E` is `[E, C, A, D, B, object]`.
Prev. Tutorial : Method Overriding
Next Tutorial : Issubclass() function