Beginner Level
Intermediate Level
Advanced Level
Introduction
The os
module of python has various functions to deal with different Operating Systems including path-related operations. In this tutorial, we will focus on the os.path
module, which provides functionalities to access and manipulate the file system-related paths of directories using Python code. By the end of this tutorial, you will be able to understand and utilize the os
module to extract, manipulate, and analyze the paths of directories in your Python projects. So, let's get started!
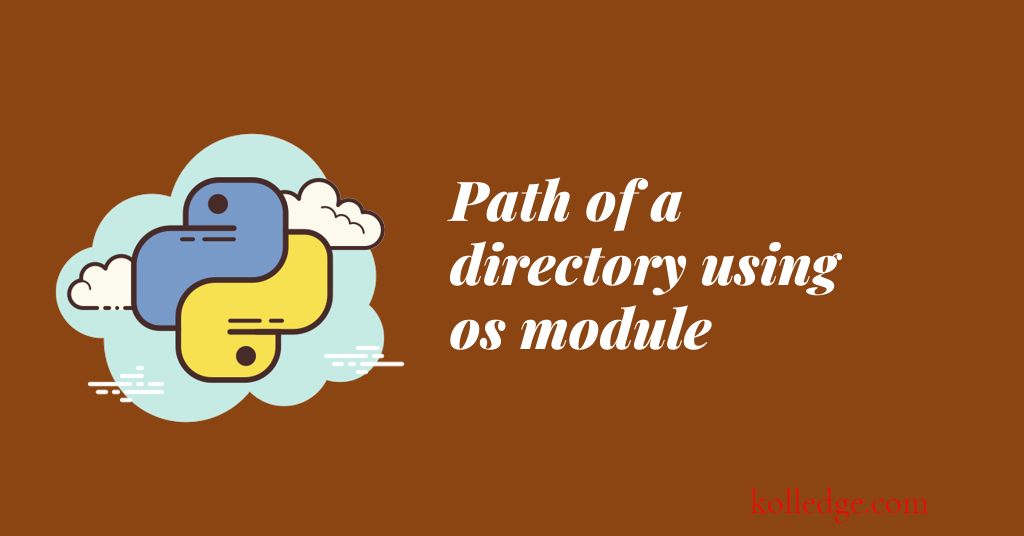
Table of Contents :
- How to get the current working directory in Python
- How to Join a Path in Python
- How to split a path in Python
- How to test if a Path is a Directory
How to get the current working directory in Python
- Import the
os
module. - Use the
os.getcwd()
function to get the current working directory. - Code sample :
import os
current_dir = os.getcwd()
print(current_dir)
How to Join a Path in Python
- Use the
os.path.join()
method to join one or more path components. - Code sample :
import os
path = os.path.join("C:", "Users", "username", "Documents")
print(path)
How to split a path in Python
- Use the
os.path.split()
method to split a path into its components. - Code sample :
import os
path = "C:/Users/username/Documents"
split_path = os.path.split(path)
print(split_path)
How to test if a Path is a Directory
- Use the
os.path.isdir()
method to test if a path is a directory. - Code sample :
import os
path = "C:/Users/username/Documents"
is_directory = os.path.isdir(path)
print(is_directory)
Prev. Tutorial : Rename Files
Next Tutorial : Directory operations