Introduction
When we write code in Python, we use different words and expressions to instruct the computer to perform specific tasks. These words and expressions have special meanings within the Python language, and they are known as Keywords and Identifiers. As a beginner in Python programming, it is essential to understand these two concepts as they are fundamental building blocks of the language. This tutorial will walk you through what Keywords and Identifiers are, how to use them, and their significance in Python programming. So let's dive in and learn all about Keywords and Identifiers!
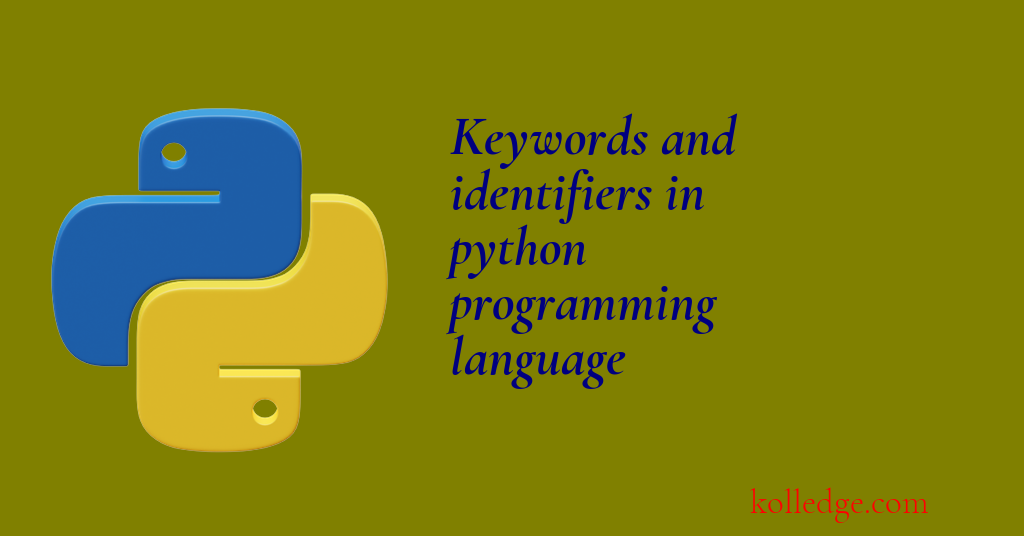
Table of Contents :
- Python Keywords
- Python Identifiers
- Rules for Naming an Identifier
- Some Points to keep in mind while naming identifiers
Python Keywords
Here are some of the main points to understand and remember about keywords in python -
- Some words have special meanings for Python compiler. These words are called keywords.
- Keywords are predefined and reserved words used in Python programming.
- These words are used to define the structure and syntax of Python language.
- Keywords cannot be used as a variable name, function name, or any other identifier.
- All the keywords except three are written in lowercase.
- The three keywords that are not in lowercase are:
- True
- False
- None
- The number of keywords in Python might change as the language evolves.
- There is a special module in Python called
keyword
for listing its keywords. - To find the current list of keywords, we can use the following code :
import keyword
print(keyword.kwlist)
- The following table shows the list of keywords in Python at the time of writing :
False | class | finally | is | return | None |
continue | for | lambda | try | True | def |
from | nonlocal | while | and | del | global |
not | with | as | elif | if | or |
yield | assert | else | import | pass | break |
except | in | raise |
Python Identifiers
- Identifiers in Python are the names given to :
- variables,
- functions,
- modules,
- classes, and
- other objects.
- For example :
language = ‘Python’
- Here, language is a variable (an identifier) which holds the value 'Python'.
- Keywords cannot be used as variable names as they are reserved names in Python. For example :
Rules for Naming an Identifier
Below we have listed some rules and naming conventions for naming identifiers in python :
- An identifier can be a combination of
- letters in lowercase (a-z),
- uppercase (A-Z),
- digits (0-9) and
- underscores (_).
- The first character of an identifier must be a letter or an underscore.
- Python is a case-sensitive language. This means that identifiers such as
myClass
andmyclass
are not the same. - Keywords cannot be used as Identifiers.
- White-spaces are not allowed in Python Identifiers.
- Identifiers cannot have special symbols like !, @, #, $ etc.
- The following are examples of some valid identifiers in Python:
- my_variable
- myVariable
- MY_VARIABLE
- my_variable_1
- myvariable
- count,
- float_value,
- max_index,
- var2,
- converted_to_float
- The following are examples of some invalid identifiers in Python :
- 1_my_variable
- my-variable
- my variable
- @count,
- float,
- max index,
- 2var,
- converted to_float
Some Points to keep in mind while naming identifiers
- Python is a case-sensitive language. This means,
count
andCount
are different identifiers. - We should try and give the identifiers some name that make sense.
- Sensible names makes our code readable and easier to understand when we look at it after long time.
- E.g. While
i = 100
is a valid identifier, writingindex = 100
makes more sense.
- We should separate multiple words using an underscore e.g. count_of_items
Prev. Tutorial : Top IDEs for Python
Next Tutorial : Statements and indentation