Introduction
One crucial aspect of computer programming is the understanding and manipulation of data. Data types are fundamental units for data manipulation, organisation, and storage in Python programming. Python is renowned for its ability to process different data types, including strings, numbers, and lists, which all form part of its core libraries. Understanding data types in Python is, therefore, a vital prerequisite to becoming a proficient developer. In this tutorial, we will explore the essential data types in Python through numerous examples and practical exercises, enabling you to become an expert in data type manipulation in Python.
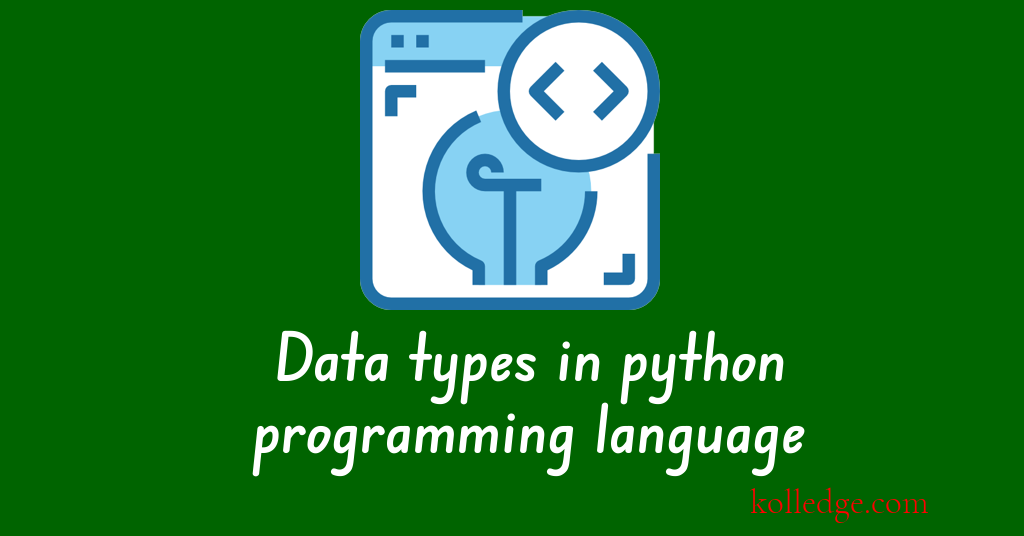
Table of Contents :
- What is Data Type in Programming
- Dynamically typed languages
- Statically typed languages
- Data Types in Python
- Changing data type of a variable in Python
- Changing Data type through reassignment
- Changing Data type through type casting
- Built-in Data Types in Python
- Numeric Data Types
- Sequence Data Types
- Text Sequence Data Types
- Binary Sequence Data Types
- Set Data Types
- Mapping Data Types
- Boolean Data Types
- None Type
- Getting the type of a value
- type() function
- isinstance() function
- Mutable vs immutable data types in Python
- Mutable data types
- Immutable data types
What is Data Type in Programming
- Data Type of a variable in any programming language specifies the type of data we can store in the variable.
- Data type tells the compiler or interpreter (interpreter in case of Python) how the variable will be used in the program. e.g.
- a variable with int data type can be used to store integers.
- a variable with string data type can be used to store strings of characters.
- a variable with list data type can be used to store list of values. etc.
- Most programming languages support data types like integer, string, character, boolean etc.
- Data type of a variable also determines how much memory should be allocated for the variable.
- Programming languages can be classified into two categories -
- Dynamically typed
- Statically typed
Dynamically typed languages
- In case of dynamically typed languages
- the data type of variables need not be declared explicitly at the time of declaring the variable.
- the data type of the variable is determined at the runtime.
Statically typed languages
- In case of statically typed languages
- the data type of the variable need to be mentioned explicitly at the time of declaration.
- the data type of the variable is determined at compile time.
Data Types in Python
- In Python Data types are implemented as classes.
- The variables that we declare in our code are instances of these classes.
- For Example :
- the Integer data type is implemented using an
int
class in Python. - all integers are objects of this
int
class.
- the Integer data type is implemented using an
- Python is a dynamically typed language. This means that -
- The data type of any variable need not be declared explicitly at the time of declaration.
- The data type of any variable is determined at runtime.
- The data type of the variable is determined by the type of the value assigned to it.
- We can change the data type of any variable simply by reassigning it with a value of some different type.
- The data type of any variable can also be changed explicitly through type casting.
Changing data type of a variable in Python
In Python we can change the data type of a variable in two ways -
- Reassignment
- Type casting
Changing Data type through reassignment :
- If we assign a value of some different data type to a variable the data type of the variable changes automatically.
- Code Sample :
var_x = 20
print("Type of var_x : ", type(var_x))
var_x = 20.5
print("Type of var_x : ", type(var_x))
var_y = 12.20
print("Type of var_y : ", type(var_y))
var_y = 50
print("Type of var_y : ", type(var_y))
# Output
# Type of var_x : <class 'int'>
# Type of var_x : <class 'float'>
# Type of var_y : <class 'float'>
# Type of var_y : <class 'int'>
Changing Data type through type casting :
- We can explicitly specifying the new data type of any variable.
- This method is called type casting.
- Code Sample :
var_x = 20
print("Type of var_x : ", type(var_x))
var_x = float(var_x)
print("Type of var_x : ", type(var_x))
var_y = 12.20
print("Type of var_y : ", type(var_y))
var_y = int(var_y)
print("Type of var_y : ", type(var_y))
# Output
# Type of var_x : <class 'int'>
# Type of var_x : <class 'float'>
# Type of var_y : <class 'float'>
# Type of var_y : <class 'int'>
Built-in Data Types in Python
Following are the built-in data types available in Python. We have detailed tutorials on the usage of each of these built-in data types in python -
Numeric Data Types in Python
- There are three numeric data types in python -
- Integer Data type :
- The Integer data type is implemented through an
int
class in Python. - We have a detailed tutorial on integer data type.
- The Integer data type is implemented through an
- Float data type :
- The float data type is implemented through a
float
class in Python. - We have a detailed tutorial on float data type.
- The float data type is implemented through a
- Complex data type :
- The complex data type is implemented through a
complex
class in Python. - We have a detailed tutorial on complex data type.
- The complex data type is implemented through a
- Integer Data type :
Sequence Data Types in Python
- There are three sequence data types in python -
- List Data type :
- The List data type is implemented through a
list
class in Python. - We have a detailed tutorial on List data type.
- The List data type is implemented through a
- Tuple Data type :
- The Tuple data type is implemented through a
tuple
class in Python. - We have a detailed tutorial on Tuple data type.
- The Tuple data type is implemented through a
- Range Data type :
- The Range data type is implemented through a
range
class in Python. - We have a detailed tutorial on Range data type.
- The Range data type is implemented through a
- List Data type :
Text Sequence Data Types in Python
- There is one text sequence data type in python -
- String Data type :
- The String data type is implemented through a
str
class in Python. - We have a detailed tutorial on Strings data type.
- The String data type is implemented through a
- String Data type :
Binary Sequence Data Types in Python
- There are three Binary sequence data types in python -
- Bytes Data type :
- The Bytes data type is implemented through a
bytes
class in Python.
- The Bytes data type is implemented through a
- Bytearray Data type :
- The Bytearray data type is implemented through a
bytearray
class in Python. - We have a detailed tutorial on Bytes and ByteArray data type.
- The Bytearray data type is implemented through a
- Memoryview Data type :
- The Memoryview data type is implemented through a
memoryview
class in Python. - We have a detailed tutorial on Memoryview data type.
- The Memoryview data type is implemented through a
- Bytes Data type :
Set Data Types in Python
- There are two Set data types in python -
- Set Data type :
- The set data type is implemented through a
set
class in Python.
- The set data type is implemented through a
- Frozenset Data type :
- The Frozenset data type is implemented through a
frozenset
class in Python. - We have a detailed tutorial on Set and frozenset data type.
- The Frozenset data type is implemented through a
- Set Data type :
Mapping Data Types in Python
- There is one mapping data type in python -
- Dictionary Data type :
- The Dictionary data type is implemented through a
dict
class in Python. - We have a detailed tutorial on Dictionary data type.
- The Dictionary data type is implemented through a
- Dictionary Data type :
Boolean Data Types in Python
- There is one Boolean data type in python -
- Bool Data type :
- The Bool data type is implemented through a
bool
class in Python. - We have a detailed tutorial on Bool data type.
- The Bool data type is implemented through a
- Bool Data type :
None Type
- In Python, the None value is a special data type that represents the absence of a value.
- The None type is implemented through a
NoneType
class in Python. - We have a detailed tutorial on None type.
Getting the type of a value
- In Python we can get information about the data type of any variable from our code.
- Pyhton provides two built-in functions to retrieve the data type information of any variable
- type()
- isinstance()
type() function :
- The type() function checks and returns the data type of the variable.
- Code Sample :
index = 20
print("Type of index : ", type(index))
# Output
# Type of index : <class 'int'>
isinstance() function :
- The isinstance() function checks whether an object is an instance of a particular class.
- Code Sample :
index = 20
t = isinstance(index, int)
print(t)
# Output
# True
Mutable vs immutable datatypes in Python
In Python each datatype can be classified as mutable or immutable.
Mutable Data types in Python :
- Mutable datatypes in python are those in which value of the object can be changed even after defining.
- built-in mutable datatypes in python are -
- Lists
- Sets
- Dictionaries
- Code Sample :
my_list = [10, 20, 30]
print(my_list)
# Adding one more element to the list
# Value of the my_list object will be changed
# Showing that lists are mutable
my_list.append(40)
print(my_list)
# Output
# [10, 20, 30]
# [10, 20, 30, 40]
Immutable Data types in Python :
- Immutable data types are those in which value of the object cannot be changed once defined.
- built-in immutable data types in python are -
- Numbers
- Strings
- Tuples
- Frozensets
- Let's try to understand immutability in python using an example of strings
- Once we have created an string literal we cannot change it's contents.
- We can assign string literals to variables.
- We can reassign new string literals to the same variable.
- But individual characters within the string literal cannot be changed.
- Code Sample :
# Assigning a string literal to a variable
message = "hello Kolledge Scholars!!"
print(message)
# Output
# hello Kolledge Scholars!!
# Assigning another string literal to the same variable
message = "howdy Kolledge Scholars!!"
print(message)
# Output
# howdy Kolledge Scholars!!
# Accessing the first character of the string literal
ch = message[0]
print(ch)
# Output
# h
# Trying to change the value of string literal
# This code gives error as strings are immutable
message[0] = "H"
print(message)
# Output
# TypeError: 'str' object does not support item assignment
Prev. Tutorial : Using String methods
Next Tutorial : Integer Data type