Beginner Level
Intermediate Level
Advanced Level
Introduction
One of the most fundamental areas of programming is controlling the flow of time in your code. This is where the time.sleep() function comes in. In Python, time.sleep() allows you to pause the execution of your program for a certain amount of time, giving your code a chance to catch up and respond appropriately. Whether you're working on a game, simulation, or any other application where timing is crucial, understanding how to use time.sleep() is an essential skill for any Python programmer. In this tutorial, we will explore the ins and outs of using the time.sleep() function effectively.
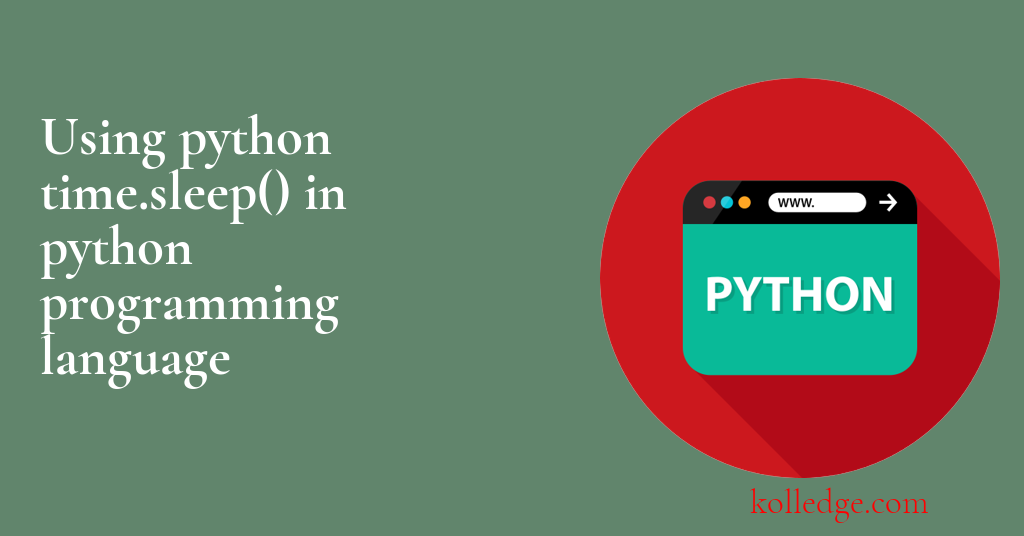
Table of Contents :
- time.sleep() function
- Some points to remember
- Code samples
- Example 1: Pausing Execution for 2 seconds
- Example 2: Using float number as argument
time.sleep() function
- The
time.sleep()
function is a Python built-in function that allows us to suspend execution of a program for a given amount of time. - Python's time.sleep() function is a handy way to slow down our code without using a lot of resources.
- This function suspends execution of the current thread for a given number of seconds.
- For example, let's say we have a loop that is supposed to run for 10 seconds. But we want it to run a little bit slower, so we add a
time.sleep()
call in the middle of the loop: - Code Sample :
import time
start_time = time.time()
for i in range(10):
time.sleep(0.5)
print(i, end=' ')
end_time = time.time()
print("Duration: {} seconds".format(end_time - start_time))
# Output
# 0 1 2 3 4 5 6 7 8 9
# Duration: 5.125827074050903 seconds
- If we want to make our code run even slower, we can increase the sleep time.
- But care should be taken of not to make it too slow, or our code will start to use a lot of resources and could even freeze.
- The syntax of the time.sleep() function is :
time.sleep(seconds)
- The time.sleep() function takes one argument :
- the number of seconds to delay the program execution
- The argument of sleep() function can be a floating-point number for sub-second resolution.
Some points to remember
- Use time.sleep() function to introduce artificial delay, for example while testing a network request or file store.
- Avoid using time.sleep() function inside loops,
- use of other methods like asyncio.sleep() function for asynchronous tasks can help with performance and avoid blocking of the event loop.
Code samples :
Example 1: Pausing Execution for 2 seconds
import time
start_time = time.time()
print("Starting...")
time.sleep(2)
print("...Stopped")
end_time = time.time()
print("Duration: {} seconds".format(end_time - start_time))
# Output:
# Starting...
# ...Stopped
# Duration: 2.011787176132202 seconds
Example 2: Using float number as argument
import time
start_time = time.time()
print("Starting...")
time.sleep(2.5)
print("...Stopped")
end_time = time.time()
print("Duration: {} seconds".format(end_time - start_time))
# Output:
# Starting...
# ...Stopped
# Duration: 2.5042598247528076 seconds
Prev. Tutorial : Using time module
Next Tutorial : Timezones