Beginner Level
Intermediate Level
Advanced Level
Introduction
Constructors are a fundamental concept in object-oriented programming that allow for the creation and initialization of objects. In Python, constructors are special methods that are automatically called when an object of a class is created. They can be used to set initial values for variables and perform other setup tasks. Understanding how to use constructors is essential for building efficient and scalable applications in Python. In this tutorial, you will learn everything about constructors in Python, how to define them and how to put them into practice in your Python programs. So let's get started!
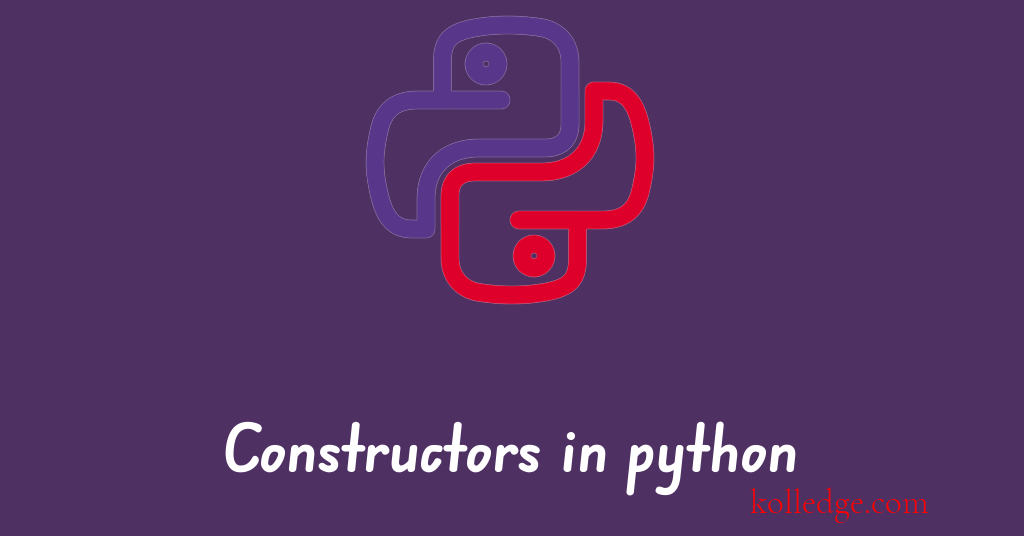
Table of Contents :
- What are Constructors in Python
- Types of constructors in Python
- Default constructors
- Non-parameterized constructors
- Parameterized constructors
- Parameterized constructors with default values
- Self keyword in Python
- Constructor overloading
- super() function
What are Constructors in Python
- In Python Constructors are special functions that are used to create and initialize an object.
- A constructor is executed automatically when we create an object.
- The constructor declares and initializes the instance variables of the class.
- In Python the object creation is divided into two step parts -
- The
__new__
method creates the object. - The
__init__
method is used to implement the constructor for initializing the object.
- The
- The basic syntax of a constructor is as follows :
def __init__(self):
# Initialization code
- In the code above :
- The
__init__
method is a reserved method and is called as soon as an object is created. - self argument refers to the current object.
- The
- The
__init__
method can have any number of arguments. - For every object the constructor is called only once, i.e. while creating the object.
- The constructors do not return anything in Python.
- Every class has a default constructor but we can define our own constructor explicitly.
- Defining the constructor is not mandatory in Python.
- If we do not define a constructor explicitly, the default constructor of the class is called while creating the objects.
- Code Sample : In the
Employee
class from our previous tutorial a constructor was defined :
def __init__(self, name, id, desig):
self.name = name
self.id = id
self.desig = desig
Types of constructors in Python :
- There are different types of constructors in Python :
- Default constructors
- Non-parameterized constructors
- Parameterized constructors
- Parameterized constructors with default values
Default constructors :
- If we do not define a constructor in our class, Python provides a default constructor for the class.
- It is an empty constructor without any body.
- The only thing this constructor is initialize the objects and does not perform any other task.
- If we write our own constructor, the default constructor is ignored.
Non-Parameterized constructor :
- As the name suggests, non-parameterized constructor is a constructor with no parameters.
- This is a user-defined constructor.
- We can use this type of constructor to initialize data members of objects with default values.
- No arguments are passed while creating objects with non-parameterized constructors.
- There is one parameter called
self
in the non-parameterized constructor as well. - The self parameter is used to refer to the current object that is being constructed.
- Code Sample :
def __init__(self):
self.cost = 100
self.rate_of_int = 0.5
Parameterized constructor :
- A constructor with parameters is called a parameterized constructor.
- We can pass arguments while creating objects with parameterized constructor.
- The data members of the object are initialized with the arguments that we pass in the constructor.
- The first parameter of the parameterized constructor is
self
. - The
self
parameter is used to refer to the current object that is being constructed. - There can be any number of arguments in a parameterized constructor.
- Code Sample :
def __init__(self, name, id, desig, email):
self.name = name
self.id = id
self.desig = desig
self.email = email
Parameterized constructors with default values :
- This is a constructor in which some parameters can have default value in the
__init__
method. - These default values will be used if we do not pass these arguments at the time of creating the object.
- Code Sample :
def __init__(self, name, id, desig, dept="Finance"):
self.name = name
self.id = id
self.desig = desig
Self keyword in Python :
- Self keyword is used to refer to the current object.
- All instance methods have self as the first parameter.
- Self refers to the object which is calling the instance method at that time.
- Python interpreter passes the object reference automatically as the first argument of all instance methods.
- It is not mandatory to name this first argument as self.
- We can name this first argument as anything we like, but it is a convention to name it as
self
and it is advised to follow this convention.
Constructor overloading
- Python does not support constructor overloading.
- Many programming languages support Constructor overloading.
- Constructor overloading is a mechanism in which a class can have more than one constructors for a class.
- The differentiating factor of the constructors is the number and type of parameters.
- In Python if we define more than one constructors the interpreter will consider only the last constructor.
super() function :
- This section will be better understood only after clearly understanding our tutorials on inheritance.
- When we are dealing with inheritance, it is often needed to call the constructor of the parent class from the child class.
- The
super()
function in Python can be used to invoke parent constructor from child constructor. - This process is also known as constructor chaining.
- When an object of child class is initialized, the constructors of all the parent classes are called and then the constructor of child class is called.
- Code Sample :
class Animal:
def __init__(self, type):
self.type = type
# class Dog inherits from Animal
class Dog(Animal):
def __init__(self, name):
self.name = name
super().__init__("Dog")
d = Dog("Bruzo")
print(f"Type of Animal = {d.type}") # instance variable of Parent class
print(f"Name of {d.type} is {d.name} ")
# Output
# Type of Animal = Dog
# Name of Dog is Bruzo
Prev. Tutorial : OOPs in Python
Next Tutorial : Destructors