Introduction
Python is a high-level, interpreted programming language with a reputation for being simple and easy-to-learn. One of the first concepts a Python programmer must grasp is the concept of literals. Literals are the simplest and most basic elements used in a programming language to represent data. They are used to represent values, such as numbers and strings, directly in the source code. In Python, the different types of literals include integer, floating-point, boolean, and string literals. Understanding the use and types of literals is crucial in developing an accurate and functional Python program. In this tutorial, we will explore the different types of literals in Python and how to use them effectively in your code.
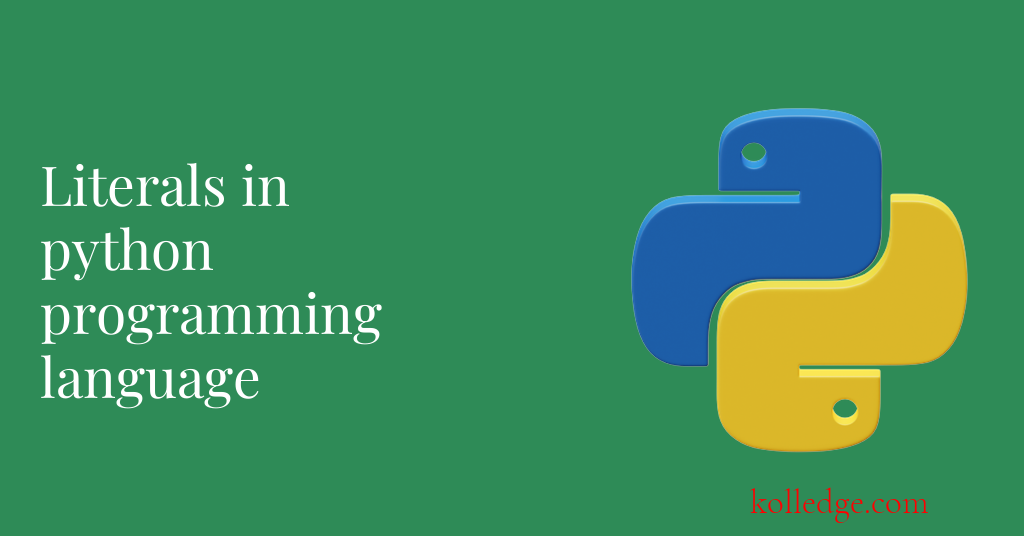
Table of Contents :
- String literals in Python
- Numeric literals in Python
- Integer Literals
- Floating point Literals
- Complex Literals
- Boolean literals in Python
- Literal Collections in Python
- List
- Tuple
- Dictionary
- Set literals
- Special literals in Python
Different types of literals in Python
- Literals in Python can be simply defined as the data values that we assign to a variable or constant.
- Different types of literals in Python are as follows:
- String literals
- Numeric literals
- Integer Literals
- Floating point Literals
- Complex Literals
- Boolean literals
- Literal Collections
- List
- Tuple
- Dictionary
- Set literals
- Special literals
String literals in Python -
- String literal is a sequence of one or more characters enclosed within quotation marks.
- We can use single quotes, double quotes or triple quotes to create string literals in Python.
- String literals can be single line or multi-line.
- We use triple quotes to create multi-line string literals.
- In python we do not have separate character literals.
- Character literals are simply string literal with length 1
- Code Sample :
str_lit = "A String literal"
print(str_lit)
print()
mult_line = '''
This is a multi-line string literal.
Created using triple quotes.
'''
print(mult_line)
# Output
# A String literal
#
#
# This is a multi-line string literal.
# Created using triple quotes.
Numeric literals in Python -
- Numeric literals are immutable.
- Python supports three types of numerical literals:
- Integer literals
- Floating-point literals
- Complex literals
Integer Literals in Python
- An integer literal is a whole number.
- Integer literals cannot have fractional part.
- Integer literals must have at least one digit.
- Integer literals can be positive, negative, or zero.
- Integer literals can be optionally preceded by a + or - sign.
- Some examples of integer literals are:
42 , -100, 0
- Before Python 3.0 there used to be another literal called long integer.
- Long integer was nothing but integers with unlimited length.
- Long literals have been dropped since Python 3.0
Floating point Literals in Python
- A floating-point literal is a number that has a decimal point.
- Floating-point literals can be positive, negative, or zero.
- They can also be optionally preceded by a + or - sign.
- Some examples of floating-point literals are:
3.14, -0.01, 0.0
Complex Literals in Python
- Complex literals are numeric literals that contain a real part and an imaginary part.
- Complex literals are written with a real part, followed by an imaginary part, separated by a + or - sign.
- The basic format of writing complex literal in python is a + bj where
- a is the real part and
- b is the imaginary part.
- The imaginary part is denoted by adding an imaginary unit j
- In Python, j is used instead of i because i is already a reserved keyword.
- Some examples of complex literals are:
1+2j, 3.5-4j, 0+0j
Boolean literals in Python -
- In Python a Boolean literal can have two possible values -
- True
- False
Literal collections in Python -
- Literal collections are used when we need to work with more than one values.
- There are four types of literal collections in Python.
- List
- Tuple
- Dictionary
- Set literals
List literals in Python
- A list literal in python is a collection of items of different data types.
- Lists are declared by enclosing list elements within square brackets '[ ]'.
- The list elements are separated by comma.
- Lists are mutable.
- Some examples of list literals can be :
[10, 20, 30] , ["John", “Peter”] , [2.1, 3.14, 10]
Tuple literals in Python
- A tuple literal in python is a collection of items of different data types.
- Tuples are declared by enclosing elements within parenthesis '( )'.
- The tuple elements are separated by comma.
- Tuples are immutable.
- Some examples of tuple literals can be :
(10, 20, 30) , ("John", “Peter”) , (2.1, 3.14, 10)
Dictionary literals in Python
- A dictionary literal in python is a collection of key-value pairs.
- Dictionaries are declared by enclosing list key-value pairs within curly brackets ‘{ }‘.
- Dictionary can have data of different types.
- The key-value pairs are separated by comma.
- Dictionaries are mutable.
- Example :
{
"Name": "John",
"id": 4927,
"Desig": “Senior Manager”
}
Set literals in Python
- Set literal in Python is a collection of unordered data.
- Set literal is declared using curly brackets '{ }'
- Elements in set are separated by comma(,).
- Set is immutable.
- Example :
{"John",4927,"Senior Manager"}
Special literal in Python
- Python has one special literal known as 'None'.
- ‘None’ is used to define a null value.
- Comparing 'None' with 'None' returns True.
- Comparing 'None' with anything else returns False.
- Example :
None
Prev. Tutorial : Constants
Next Tutorial : Rules and naming conventions