Introduction
Python is a powerful programming language that is widely used in various industries and sectors of the economy. It is an easy to learn language that is popular among both beginners and seasoned programmers alike. In this tutorial, we will be exploring the Operator functions in Python programming language. Operators are an essential part of programming language, and they allow us to perform various mathematical and logical operations on variables, data structures, and other instances. By the end of this tutorial, you will have a firm grasp on operator functions in Python and be able to apply them in your programming projects. So let's get started!
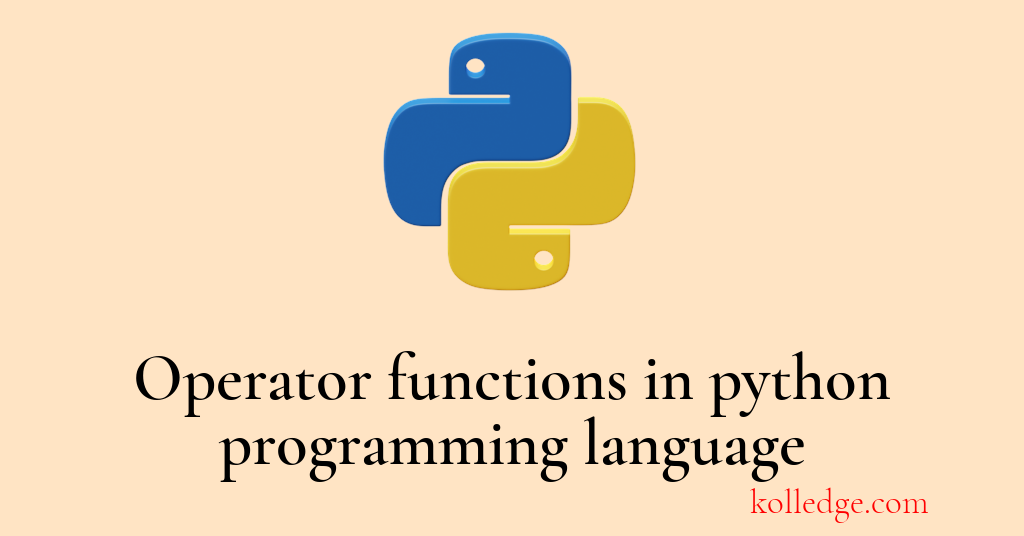
What are Dunder methods in Python?
- Dunder methods are also known as magic methods or special functions in python.
- Dunder methods can be used for operator overloading.
- Operator functions are functions that are associated with a specific operator.
- For example, the
+
operator is associated with the__add__()
function. - When you write code like
3 + 4
, the Python interpreter calls the__add__()
function on 3, passing 4 as an argument. - The
__add__()
function then returns the result of the addition, which is 7. - There are many other operator functions,
__sub__()
for subtraction__mul__()
for multiplication__truediv__()
for division.
- In addition to the standard operator functions, we can also override the behavior of the operators in our own classes.
- For example, if we have a class that represents a complex number, we can override the
+
operator to add two complex numbers together. - Here is a simple example of overriding the + operator:
class ComplexNumber:
def __init__(self, real, imaginary):
self.real = real
self.imaginary = imaginary
def __add__(self, other):
return ComplexNumber(self.real + other.real, self.imaginary + other.imaginary)
c1 = ComplexNumber(1, 2)
c2 = ComplexNumber(3, 4)
c3 = c1 + c2
print(c3.real, "+ j",c3.imaginary)
# Output
# 4 + j 6
# In this example, we have created a class that represents a complex number.
# The __init__() method initializes the real and imaginary parts of the complex number.
# The __add__() method overrides the addition operator.
# When we add two complex numbers together, the __add__() method is called and the result is a new complex number.
- We can override any of the operators in Python.
- This can be useful for creating custom data types that behave in a natural way.
- For example, we can create a class that represents a point in three-dimensional space.
- You could then override the + operator to add two points together.
Prev. Tutorial : Operator Precedence
Next Tutorial : Taking input from user