Beginner Level
Intermediate Level
Advanced Level
Introduction
Python offers an incredibly powerful set of tools for working with lists and tuples. Filtering elements from these objects is a common task that you may find yourself needing to perform from time to time. Fortunately, Python provides a simple and efficient way to filter elements from lists and tuples using built-in functions like filter()
, lambda functions, and list comprehension. In this tutorial, we will explore how you can filter elements from your Python lists and tuples.
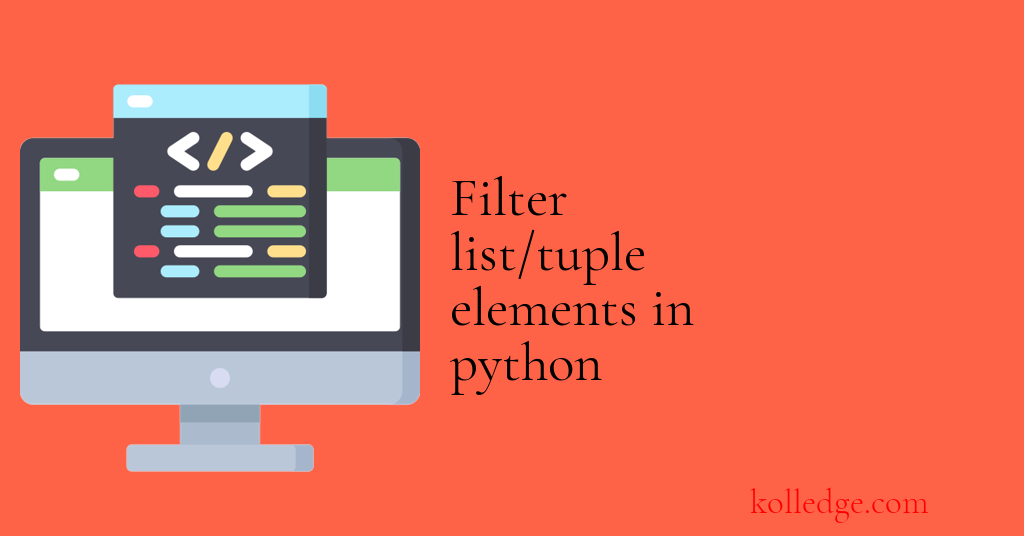
Table of Contents :
- Filter List/Tuple elements in Python
- Filtering elements using loops
- Filtering elements using filter() function
- Filtering elements using lambda expressions with filter() function
- Filtering elements using List comprehensions
Filter List/Tuple elements in Python :
- Sometimes we need to select some of the elements of the List (or tuple) based on some condition.
- This process of shortlisting elements based on some criteria is called Filtering the elements.
- We can filter the elements of a list in Python in following ways :
- Using loops
- Using filter() function
- using List comprehensions
Filtering elements using loops :
- We can iterate over all the elements using a for loop or a while loop.
- The control flow for filtering using loops :
- Check each element of the list for the condition.
- If the element satisfies the condition add the element to a new list.
- return the new list as result.
- Loops are slower than the
filter()
function discussed ahead. - Code Sample :
my_list = [10, 20, 30, 40]
print("my_list = ", my_list)
new_list = []
for item in my_list:
if 15 < item < 35:
new_list.append(item)
print("new_list = ", new_list)
# Output
# my_list = [10, 20, 30, 40]
# new_list = [20, 30]
Filtering elements using filter() function :
filter()
is a built-in function that filters list or tuple elements more smoothly.filter()
function can be used to filter elements of any iterable object - not just List or Tuple.- The syntax for filter() function is :
new_list = filter(logic_function, orig_list)
- Here :
- logic_function is the function that contains the condition on which we need to filter the elements.
- orig_list is the original list that we need to filter out.
- new_list is the list of filtered out elements.
- Control flow of filter function -
- filter() function iterates all the elements of the list (or tuple).
- It calls the logic_function() for each element.
- It creates an iterator containing the elements that satisfy the condition in the logic_function.
- filter() function then returns this new iterator as a result.
- Code Sample :
def filter_function(item):
if 15 < item < 35:
return item
my_list = [10, 20, 30, 40]
print("my_list = ", my_list)
new_list_iterator = filter(filter_function, my_list)
new_list = list(new_list_iterator)
print("new_list = ", new_list)
# Output
# my_list = [10, 20, 30, 40]
# new_list = [20, 30]
Filtering elements using lambda expressions with filter() function :
- If our filtering logic is of one line, we can use anonymous functions instead of normal function.
- Code Sample :
my_list = [10, 20, 30, 40]
print("my_list = ", my_list)
new_list_iterator = filter(lambda x: 15 < x < 35, my_list)
new_list = list(new_list_iterator)
print("new_list = ", new_list)
# Output
# my_list = [10, 20, 30, 40]
# new_list = [20, 30]
Filtering elements using List comprehensions :
- List comprehension is another method of filtering elements of a list.
- List comprehensions is a very important topic in Python.
- It is recommended that readers should go through our tutorial on List comprehensions to get a good hold of the topic.
- For the sake of completeness, below we have a simple example of filtering elements using list comprehension in python.
- Code Sample :
my_list = [10, 20, 30, 40]
print("my_list = ", my_list)
new_list = [x for x in my_list if 15 < x < 35]
print("new_list = ", new_list)
# Output
# my_list = [10, 20, 30, 40]
# new_list = [20, 30]
Prev. Tutorial : Map() function
Next Tutorial : List comprehensions