Beginner Level
Intermediate Level
Advanced Level
Introduction
As a Python programmer, you will frequently find yourself needing to manipulate strings to format your output in a way that is more readable and intuitive. That's where string formatting comes in. With Python's string formatting tools, you can easily customize the output of your strings to match your desired format, such as combining values with text, padding values with zeros or spaces, and formatting numbers and dates. In this tutorial, we will explore the various methods of string formatting in Python, so you can be confident in your ability to handle any string formatting task.
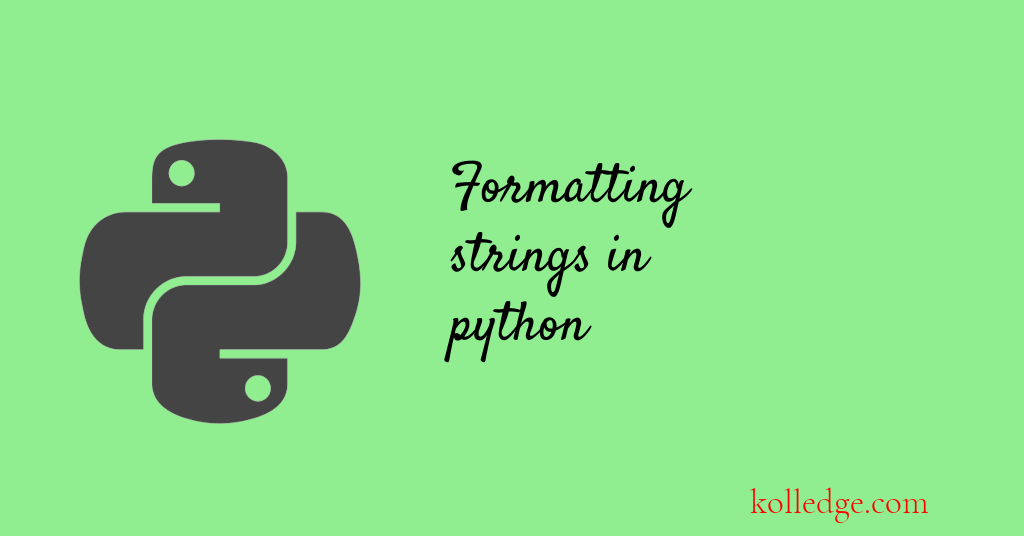
Table of Contents :
- What is String formatting in python
- Different ways of formatting strings in python
- Using the `%` operator
- Using the `str.format()` method
- Using f-strings
- Using str.format_map() method
- Using template strings
What is String formatting in python:
- String formatting is a technique used to format strings by replacing placeholders with values.
- It enables you to create dynamic strings by incorporating values of variables and literals.
Different ways of formatting strings in python:
Using the `%` operator:
- We can use the modulo operator
%
to substitute placeholders (or conversion specifiers) in a string with values specified in a tuple. - Code Sample :
name = "John"
age = 25
message = "My name is %s and I am %d years old." % (name, age)
print(message)
# Output
# My name is John and I am 25 years old.
Using the str.format() method:
- We can use the
str.format()
method to embed values in a string using placeholders. - Placeholders are defined by
{}
braces, which are replaced by values. - Code Sample :
name = "Mary"
age = 30
message = "My name is {} and I am {} years old.".format(name, age)
print(message)
# Output
# My name is Mary and I am 30 years old.
Using f-strings:
- We can use f-strings (formatted strings) to format strings with embedded expressions.
- Start the string with `f` or `F` and embed expressions in braces.
- Code Sample :
name = "Lucy"
age = 35
message = f"My name is {name} and I am {age} years old."
print(message)
# Output
# My name is Lucy and I am 35 years old.
Using str.format_map() method:
- We can use the
str.format_map()
method to apply a mapping object to the string. - The mapping object should be a dictionary that maps keys (placeholders) to values.
- Code Sample :
info = {'name': 'Peter', 'age': 40}
message = "{name} is {age} years old.".format_map(info)
print(message)
# Output
# Peter is 40 years old.
Using template strings:
- We can use template strings to substitute placeholders with values using a template string.
- The template string defines placeholders using
$
sign followed by a name. - Code Sample :
from string import Template
name = "Sarah"
age = 33
t = Template("$name is $age years old.")
message = t.substitute(name=name, age=age)
print(message)
# Output
# Sarah is 33 years old.
Prev. Tutorial : Escape sequencing a string
Next Tutorial : String Methods