Beginner Level
Intermediate Level
Advanced Level
Introduction
The Map() function in Python is a built-in method that allows us to apply a function to every element in an iterable such as a list, tuple or dictionary. This is accomplished by passing both the function and the iterable as parameters to the Map() function. Using Map() can significantly reduce the amount of code required to perform repetitive operations on large data structures, and it is a valuable tool to have in any Python developer’s toolkit. In this tutorial, we will explore the Map() function in depth, looking at its syntax and how to use it effectively in our Python programs.
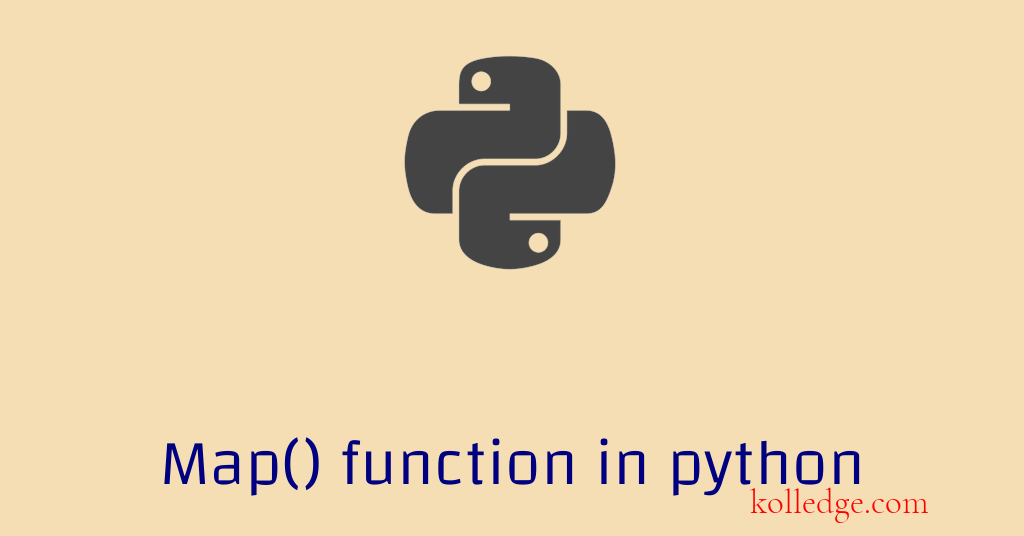
Table of Contents :
- Transforming list elements in Python
- Using loops
- Using map() function
- Using Lambda expression within the map() function
- List comprehensions
Transforming list elements in Python :
- Sometimes we need to apply some logic to each element of the list( or tuple).
- In the result we get a new list(or tuple) with transformed elements.
- This transformation can be carried over in different ways :
- Using loops
- using built-in map function
- List comprehensions
Using loops :
- We can iterate over all the elements using a for loop or a while loop.
- apply the operation to be performed.
- Add the transformed element to a new list.
- return the new list as result.
- Loops are slower than the
map()
function discussed ahead. - Code Sample :
my_list = [1, 2, 3, 4]
print("my_list = ", my_list)
new_list = []
for item in my_list:
sqr_item = item ** 2
new_list.append(sqr_item)
print("new_list = ", new_list)
# Output
# my_list = [1, 2, 3, 4]
# new_list = [1, 4, 9, 16]
Using map() function :
- The built-in
map()
function is a faster and better way of doing this task. - The transformation logic is written in a separate function which is then passed to the
map()
function as an argument. - The control flow of
map()
function is as follows :- The
map()
function iterates over all the elements of the list(or tuple). - call the function with transformation logic on each element.
- returns a new iterator object of the transformed elements.
- The
- The syntax of the map function is :
new_iterator = map(logic_function, orig_list)
- Here -
- logic_function is the function that contains the transformation logic for each element.
- orig_list is the original list on whose elements the transformation needs to be performed.
- new_iterator is the iterator object with new transformed elements.
- We can use the map() function to transform elements of any iterable, not just list or tuple.
- Code Sample :
def trans_function(item):
sqr_item = item ** 2
return sqr_item
my_list = [1, 2, 3, 4]
print("my_list = ", my_list)
new_list_iterator = map(trans_function, my_list)
new_list = list(new_list_iterator)
print("new_list = ", new_list)
# Output
# my_list = [1, 2, 3, 4]
# new_list = [1, 4, 9, 16]
Using Lambda expression within the map() function :
- Using lambda expression is actually a different way of using the map function.
- If the transformation logic we need to apply is a one line logic, then we can use lambda expression instead of a function.
- Code Sample :
my_list = [1, 2, 3, 4]
print("my_list = ", my_list)
new_list_iterator = map(lambda x: x ** 2, my_list)
new_list = list(new_list_iterator)
print("new_list = ", new_list)
# Output
# my_list = [1, 2, 3, 4]
# new_list = [1, 4, 9, 16]
List comprehensions :
- List comprehension is another method of applying transformation logic to each element of a list.
- List comprehensions is a very important topic in Python.
- We have a dedicated tutorial for List comprehensions.
- It is recommended that readers should go through our tutorial on List comprehensions to get a good hold of the topic.
- For the sake of completeness, below we have a simple example of list comprehension in python.
- Code Sample :
my_list = [1, 2, 3, 4]
print("my_list = ", my_list)
new_list = [x ** 2 for x in my_list]
print("new_list = ", new_list)
# Output
# my_list = [1, 2, 3, 4]
# new_list = [1, 4, 9, 16]
Prev. Tutorial : Iterables
Next Tutorial : Filter List/Tuple elements