Beginner Level
Intermediate Level
Advanced Level
Introduction
One of the key features of Python is its ability to use keyword arguments in function calls. Keyword arguments are useful when the order of arguments is not important, and can make code more readable by explicitly defining the meaning of each argument. In this tutorial, we will explore keyword arguments in Python and learn how to use them to make our code more efficient and easier to understand.
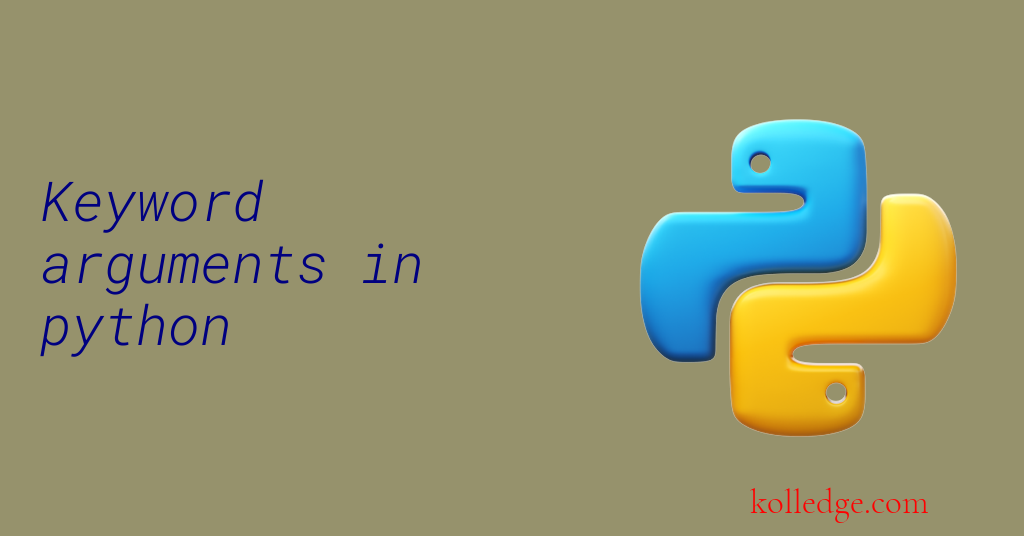
Keyword Arguments :
- In Keyword arguments names of the arguments are also passed along with the values while calling the function.
- In case of keyword arguments, the position of the arguments is not important.
- Keyword arguments can be passed in any order, the only thing that matters is the name of the arguments.
- Keyword arguments can be mixed with positional arguments.
- In other words, it is not necessary that all the arguments of a function call are keyword arguments.
- Keyword arguments come in handy when we have default parameters in function and we do not want to pass all arguments.
- The keyword arguments need to appear in the last of the function call.
- Suppose we want to use three keyword arguments, then these three arguments should be the last three arguments of the function call.
- In other words, a positional argument cannot be placed after any keyword argument in a function call.
- Code Sample :
def my_func(a, b, **kwargs):
print("Value of a = ", a)
print("Value of b = ", b)
print("Value of kwargs = ", kwargs)
print()
print("Iterating over kwargs")
# using for loop
for key in kwargs.keys():
print(key,kwargs[key])
my_func(10, 20, first=30, second=40, third=50)
# Output
# Value of a = 10
# Value of b = 20
# Value of kwargs = {'first': 30, 'second': 40, 'third': 50}
# Iterating over kwargs
# first 30
# second 40
# third 50
Prev. Tutorial : Keyword parameters
Next Tutorial : Partial Functions