Beginner Level
Intermediate Level
Advanced Level
Introduction
One of the most useful data structures in Python is the tuple. Tuples in Python are very similar to lists, with one key difference - they are immutable, meaning that once they are created, their size and contents cannot be changed. This makes them useful for situations where you need to store a fixed set of values that won't be modified. In this tutorial, we'll explore the basics of working with tuples in Python, including how to create them, access their values, and perform various operations on them.
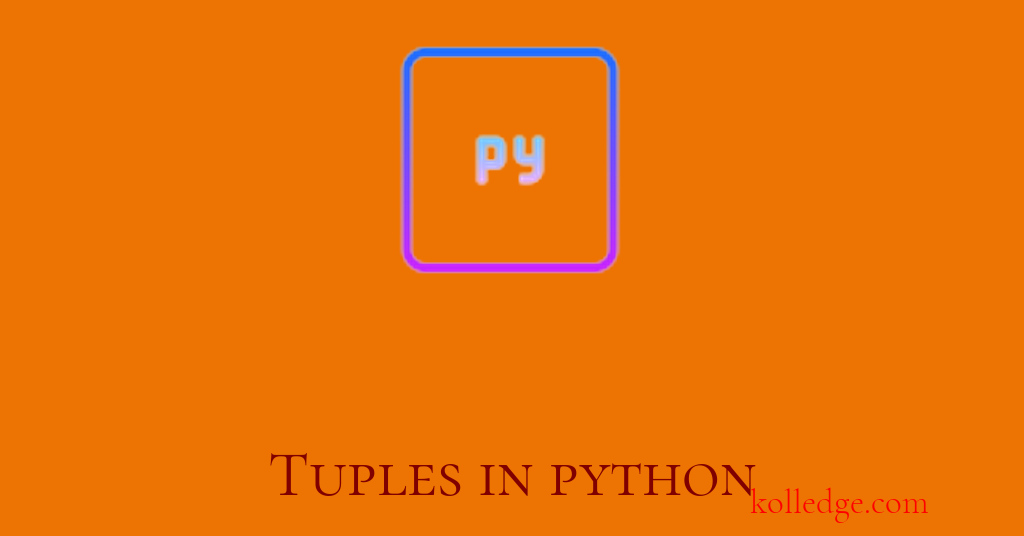
Table of Contents :
- Tuples in Python
- Accessing elements of Tuple
- Tuple with one element
Tuples in Python :
- A tuple in Python is an immutable list.
- The tuple data type is implemented using the
tuple
class. - In simple words Tuple is a list that cannot be changed once created.
- Tuple is defined in Python using parentheses - ().
- A tuple can contain one or more elements within the parentheses separated by comma.
- The syntax of creating a tuple is as follows :
new_tuple = (item1, item2, item3)
- Code Sample :
my_tuple = (10, 20, 30)
print(f"my_tuple = {my_tuple}")
print(f"Data Type of my_tuple = {type(my_tuple)}")
# Output
# my_tuple = (10, 20, 30)
# Data Type of my_tuple = <class 'tuple'>
Accessing elements of Tuple :
- Tuple is an ordered collection i.e. each element has a unique index.
- The indexes of tuples are zero based.
- The first item of a tuple in Python has an
index = 0
, the second item hasindex = 1
- Like lists the elements of a tuple in Python can be accessed using their index.
- Code Sample :
my_tuple = (10, 20, 30)
print(f"my_tuple = {my_tuple}")
element_1 = my_tuple[0]
element_2 = my_tuple[1]
element_3 = my_tuple[2]
print(f"Element 1 = {element_1}")
print(f"Element 2 = {element_2}")
print(f"Element 3 = {element_3}")
# Output
# my_tuple = (10, 20, 30)
# Element 1 = 10
# Element 2 = 20
# Element 3 = 30
Tuple with one element :
- To create a tuple of one element in Python we need to add a comma within the parentheses after the element.
- In case we do not add the trailing comma, python interpreter will not treat it as tuple.
- It will treat it of same data type as that of the element.
- Code Sample :
var_1 = (10, )
print(f"Data Type of var_1 = {type(var_1)}")
print("var_1 is a tuple with 1 element")
print()
var_2 = (10)
print(f"Data Type of var_2 = {type(var_2)}")
print("var_2 is an integer")
# Output
# Data Type of var_1 = <class 'tuple'>
# var_1 is a tuple with 1 element
# Data Type of var_2 = <class 'int'>
# var_2 is an integer
Prev. Tutorial : Unpacking a list
Next Tutorial : Iterables