Beginner Level
Intermediate Level
Advanced Level
Introduction
As a Python programmer, you may have found yourself in a situation where you need to compare two objects to check if they refer to the same object or not. Specifically, you may need to check whether two variables point to the same object or not. This is where Identity operators come in handy. In Python, Identity operators is
and is not
are used to compare the memory address of two objects. In this tutorial, we will explain how to use identity operators in Python and why they are important in certain programming situations. So, let's get started!
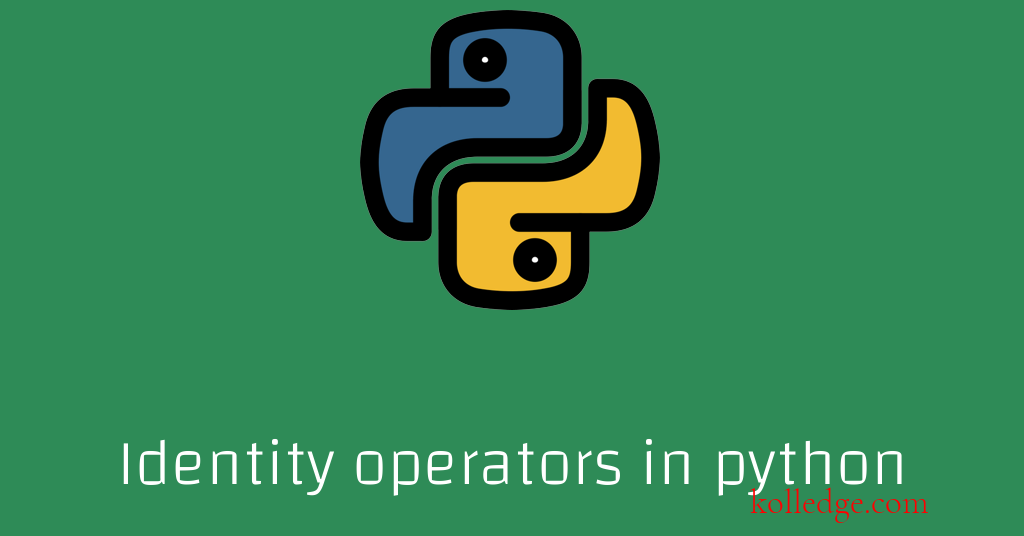
Table of Contents :
- What are Identity operators in Python
- is operator in Python
- is not operator in Python
What are Identity operators in Python
- Identity operators in Python are used to check whether two objects are actually same.
- Identity operators are also known as reference-quality operators.
- Identity operators compare not only the values of the two objects but also their memory location.
- Python has 2 identity operators
- is operator
- is not operator
is operator in Python :
- It returns True if the memory location of the first operand is equal to that of second object.
- Otherwise, it returns False.
- Code Sample :
# The value and memory location of x and y are same
x = 10
y = x
res = x is y
print(res)
# Output
# True
# The value of x and y is same
# But memory location is different
x = 10
y = float(x)
res = x is y
print(res)
# Output
# False
# The '==' operator compares only value
x = 10
y = float(x)
res = x == y
print(res)
# Output
# True
is not operator in Python :
- It returns True if the memory location of the first operand is not equal to that of second object.
- Otherwise, it returns False.
- Code Sample :
x = 10
y = x
res = x is not y
print(res)
# Output
# False
Prev. Tutorial : Membership operators
Next Tutorial : Bitwise Operators