Beginner Level
Intermediate Level
Advanced Level
Introduction
Loading JSON data into Python is a frequent task in many data science and software development projects. Python provides an easy-to-use library called JSON, which supports parsing, encoding, and decoding JSON data. In this tutorial, we will explore how to load JSON data into Python using the JSON library and other third-party libraries like pandas and NumPy. So, let's get started!
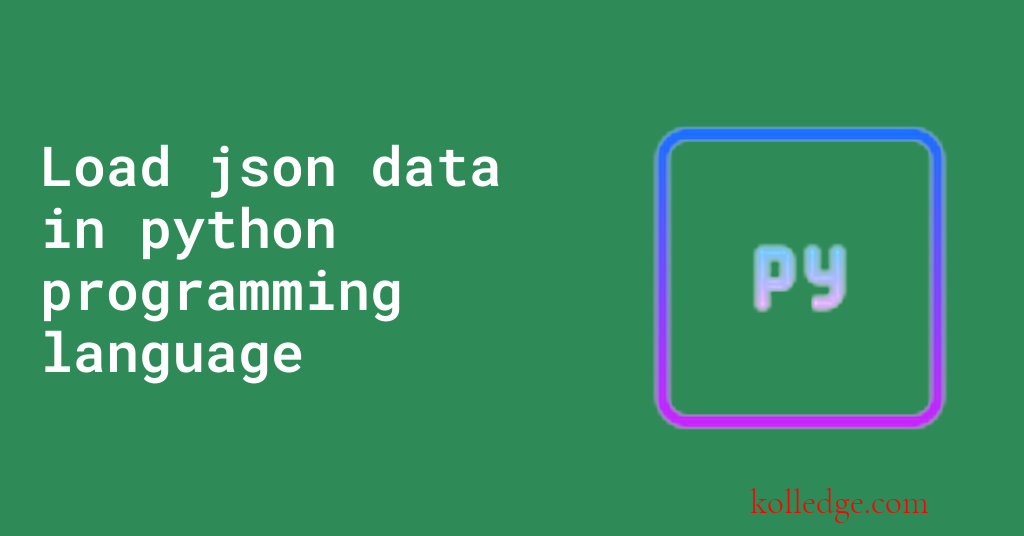
Table of Contents :
- Loading Json Data in Python
- Json.load() method
- Json.loads() method
Loading Json Data in Python :
- Python has a built-in module called json that can be used to work with JSON data.
- To read Json Data we need to load the data from json file.
- Python has two functions that can be used to load data :
- json.load() method
- json.loads() method
Json.load() method :
json.load()
function takes a file object as argument- It returns the json object.
- Steps to load json data using load method :
- open the file using open() function
- pass the file object as argument to
json.load()
method - Use
json.load()
method to load the json data.
- Code Sample : Sample code for load method
import json
# Opening JSON file
f = open('test.json',)
# returns JSON object as
# a dictionary
jdata = json.load(f)
# Iterating through the json
# list
for i in jdata['test_details']:
print(i)
# Close the file
f.close()
json.loads() method :
json.loads()
method is used to convert a valid json string into python dictionary.- It is used primarily to deserialize string, byte, or byte array which consists of JSON data into Python Dictionary.
- Arguments :
json.loads()
method takes string, bytes, or byte array instance which contains the JSON data as an argument. - It returns a Python object.
- Code Sample : Sample code for loads method
import json
# JSON string:
# Multi-line string containing json data
jstring = """{
"Emp-Id": "2398",
"Emp-Name":"Tom Grooves",
"Contact": 70079865453,
"Email-Id": "tgrooves@gmail.com",
}"""
# parse jstring :
d = json.loads(jstring)
# the result is a Python dictionary:
print(d)
Prev. Tutorial : Dump json data
Next Tutorial : PrettyPrint JSON Data