Beginner Level
Intermediate Level
Advanced Level
Introduction
Python supports the concept of functions as first-class objects - a powerful programming paradigm that allows functions to be treated as objects, making them more versatile and easier to use in your programs. In this tutorial, we will explore the concept of functions as first-class objects in Python programming language, understand how it works, and learn how to use it effectively in your code. We will also cover some advanced concepts and techniques that will help you become a better Python programmer.
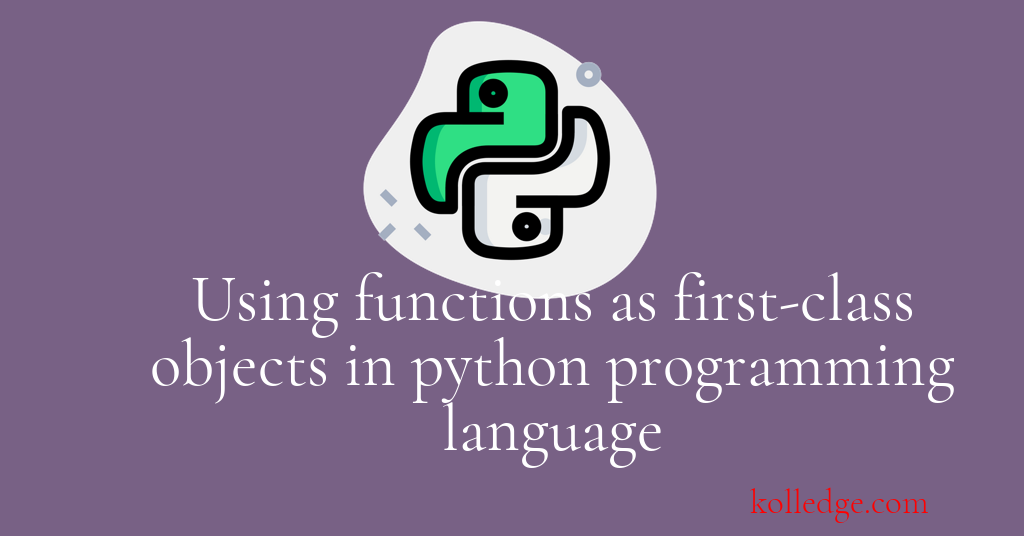
Table of Contents :
- Functions as first-class objects
- Passing functions as arguments of other functions
- Assigning to variables
- Using attributes of functions
Functions as first-class objects
- Python functions are first-class objects.
- This means that they can be created and passed around just like any other object such as string, int, list, etc.
- This has a number of implications.
Passing functions as arguments of other functions
- For one, it means that functions can be passed as arguments to other functions.
- For example, let's say we have a list of numbers and we want to filter out the even ones. We could write our own function to do this, or we could use the built-in
filter()
function, which takes a function as its first argument: - Code Sample :
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# our own function
def is_even(num):
return num % 2 == 0
# using the built-in filter function
filtered_numbers = filter(is_even, numbers)
print(list(filtered_numbers))
# [2, 4, 6, 8, 10]
Assigning to variables
- Another implication of functions being first-class objects is that they can be assigned to variables and used like any other object:
- Code Sample :
def greet(name):
print("Hello, {}!".format(name)) greet("Jill")
# Hello, Jill!
# assigning the function to a variable
greeter = greet greeter("Jack")
# Hello, Jack!
Using attributes of functions
- Finally, since functions are objects, they have attributes that can be accessed.
- For example, the
__name__
attribute contains the name of the function: - Code Sample :
def greet(name):
print("Hello, {}!".format(name))
print(greet.__name__)
# greet
Prev. Tutorial : Yield statement
Next Tutorial : Closures