Beginner Level
Intermediate Level
Advanced Level
Introduction
Sometimes the patterns we need to match through regex, may depend on a context that comes after the matched pattern, or we may need to apply a condition to our pattern that only applies for certain matches. This is where Regex Lookahead comes in handy. In this tutorial, we will explore what Regex Lookahead is, how to use it in Python, and some practical examples to illustrate its usefulness.
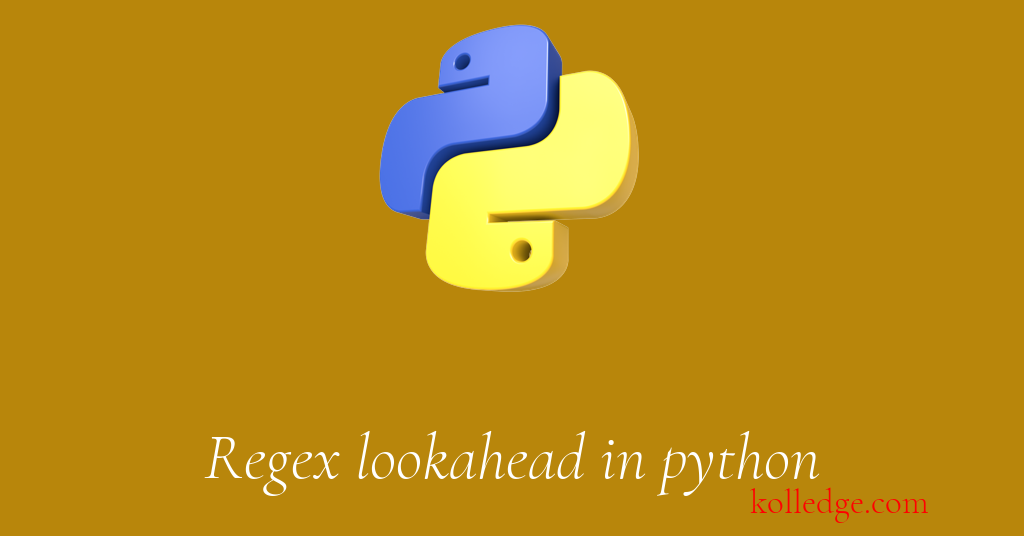
Table of Contents :
- Python Regex Lookahead
- Regex Multiple Lookaheads
- Negative Lookaheads
Python Regex Lookahead :
- Lookahead is a feature of Python's regular expression syntax that allows you to match a pattern only if it is followed by another pattern.
- Lookahead is denoted by the
(?=
sequence, which precedes the pattern to be matched. - Code Sample :
import re
# Lookahead example
text = "The quick brown fox jumps over the lazy dog."
pattern = r"\w+(?=\sfox)"
result = re.findall(pattern, text)
print(result)
# Output:
['brown']
Regex Multiple Lookaheads :
- Multiple lookaheads can be used to match a pattern only if it is followed by multiple patterns.
- The multiple patterns can be combined using the pipe
|
character. - Code Sample :
import re
# Multiple lookahead example
text = "The quick brown fox jumps over the lazy dog."
pattern = r"\w+(?=\sbrown)(?=\sfox)"
result = re.findall(pattern, text)
print(result)
# Output:
['quick']
Negative Lookaheads :
- Negative lookahead is a feature of Python's regular expression syntax that allows you to match a pattern only if it is not followed by another pattern.
- Negative lookahead is denoted by the
(?!
sequence, which precedes the pattern to be excluded. - Code Sample :
import re
# Negative lookahead example
text = "The quick brown fox jumps over the lazy dog."
pattern = r"\w+(?!\sdog)"
result = re.findall(pattern, text)
print(result)
# Output:
['The', 'quick', 'brown', 'fox', 'jumps', 'over', 'the', 'lazy']
Prev. Tutorial : Non-capturing groups
Next Tutorial : Lookbehind