Beginner Level
Intermediate Level
Advanced Level
Introduction
One essential element of working with databases is the ability to extract data based on specific criteria, which is where the SQL WHERE clause comes into play. In this tutorial, we will explore the basics of using the WHERE clause in Python, including how to filter data based on specific conditions and how to apply multiple criteria using logical operators. Whether you're a beginner or an experienced Python programmer, mastering the WHERE clause is an essential skill for anyone working with relational databases.
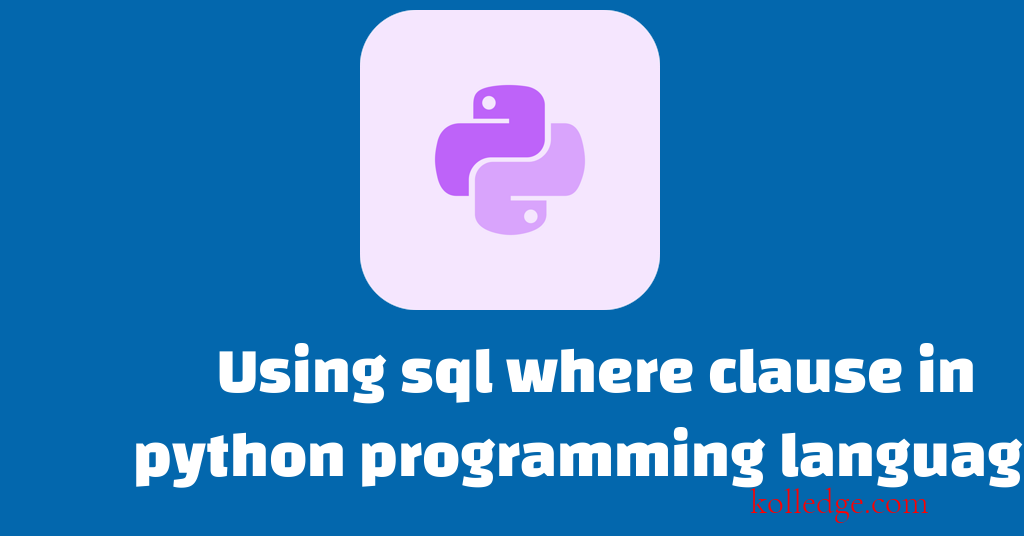
Table of Contents :
- What is a Where clause
- How to use sql where clause in MySQL with Python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: use sql where clause
What is a Where clause :
- The WHERE clause is used to filter records.
- It is used to specify a condition for which rows or columns are to be selected from a table.
- The following is a simple example of how to use the WHERE clause :
SELECT * FROM employees WHERE salary > 50000
# WHERE clause is used to select only those employees who have a salary greater than 50000.
How to use sql where clause in MySQL with Python :
- Follow the steps given below to use sql where clause in MySQL with python
- Step 1: Install the required Packages
- Step 2: Establishing a Connection to the MySQL Server
- Step 3: Create a Cursor Object
- Step 4: use sql where clause
Step 1: Install the Required Packages
- The first step is to ensure that you have installed the required packages on your machine.
- The most popular packages for connecting to MySQL in Python are
- mysql-connector and
- PyMySQL.
- You can install these packages using pip through the command line.
- Code Sample :
!pip install mysql-connector-python
!pip install PyMySQL
Step 2: Connect to the MySQL Database
- To create a new database in MySQL using Python, we must have a connection to the MySQL server.
- To connect to a MySQL server in Python we can use either
- mysql.connector or
- PyMySQL package
- We need to provide the necessary connection parameters to establish the connection.
- We need to pass the following parameters to the Connection object:
- host : the hostname of the MySQL server user
- user : username for the MySQL account
- password : password for the MySQL account database
- database : The name of the database to connect to
- To study package specific code for connecting to MySQL read our tutorial on connecting to MySQL server.
Step 3: Create a Cursor Object
- After establishing a connection to the MySQL server, your next step is to create a cursor object.
- This is done using the cursor() method provided by mysql.connector or PyMySQL.
- Example:
cursor = mydb.cursor()
Step 4: Use sql where clause
- To retrieve specific data from a MySQL table using Python, use the `WHERE` clause in your `SELECT` statement.
- Use the `%s` placeholder to avoid SQL injection attacks.
- Example:
query = "SELECT * FROM employees WHERE id = %s"
values = (1,)
cursor.execute(query, values)
result = cursor.fetchall()
for row in result:
print(row)
Prev. Tutorial : Using sql select query
Next Tutorial : Using sql orderby clause