Beginner Level
Intermediate Level
Advanced Level
Introduction
Generator functions are an important concept in Python, offering a powerful way to efficiently generate sequences of data on-the-fly. Unlike regular functions that generate all their results at once and return them in a list, generator functions generate individual results one at a time, allowing for a much more flexible and memory-efficient approach. In this tutorial, we will explore how to create and use generator functions in Python. We will cover how to define generator functions, how to iterate over the results they generate, and how to control the flow of data using built-in Python functions.
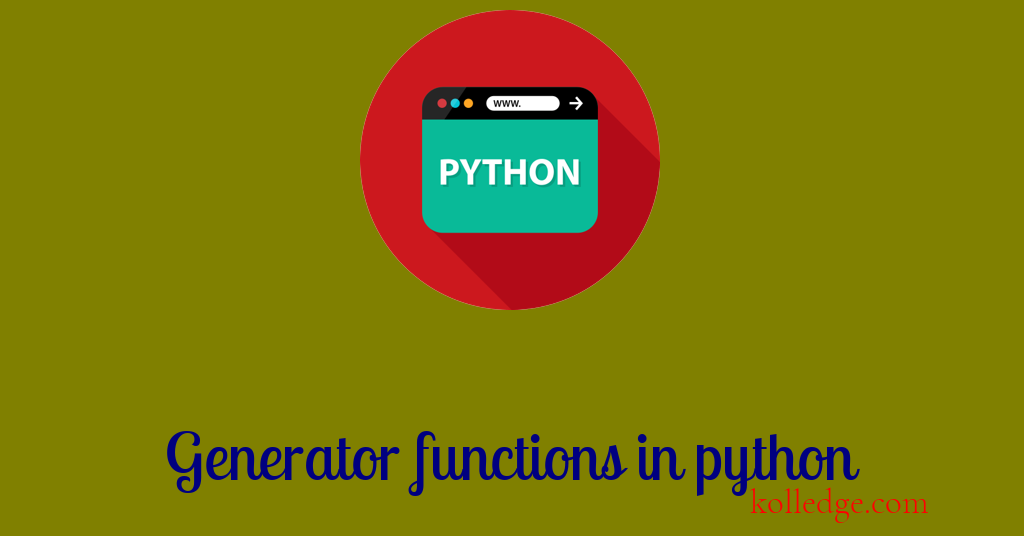
Table of Contents :
- Generators in Python
- Python generator example
- Creating iterators using Python generators
Generators in Python :
- Python generators are a way to create iterators without the need for a class definition.
- They are a convenient way to represent sequences that can be iterated over, and can also be used to generate, filter, and transform data.
- Generators work by producing a sequence of values one at a time, instead of generating all of them at once.
Python generator example :
- To create a generator, you define a function that contains one or more yield statements.
- Code Sample :
def countdown(n):
while n > 0:
yield n
n -= 1
for i in countdown(5):
print(i)
Creating iterators using generators :
- Generators can be used to create iterators that are more memory efficient than a list or other data structure.
- Using a generator for iteration allows you to process an arbitrary amount of data without having to allocate all of it to memory at once.
- Generators can also be used to filter and transform data in place.
- Code Sample :
def squares(n):
for i in range(n):
yield i 2
for i in squares(5):
print(i)
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
evens = (x for x in data if x % 2 == 0)
for i in evens:
print(i)
Prev. Tutorial : Custom Sequence Type
Next Tutorial : Generator expressions