Beginner Level
Intermediate Level
Advanced Level
Introduction
Context managers in python allow for the efficient and safe handling of resources in a program. Context managers help ensure that resources are properly cleaned up, even in the case of errors or exceptions, reducing the risk of memory leaks or other issues. They can be used for a variety of purposes, from file handling to database connections, making them an essential tool in any Python programmer's toolkit. In this tutorial, we'll explore the ins and outs of context managers in Python, including how they work, how to create and use them, and some best practices for implementation.
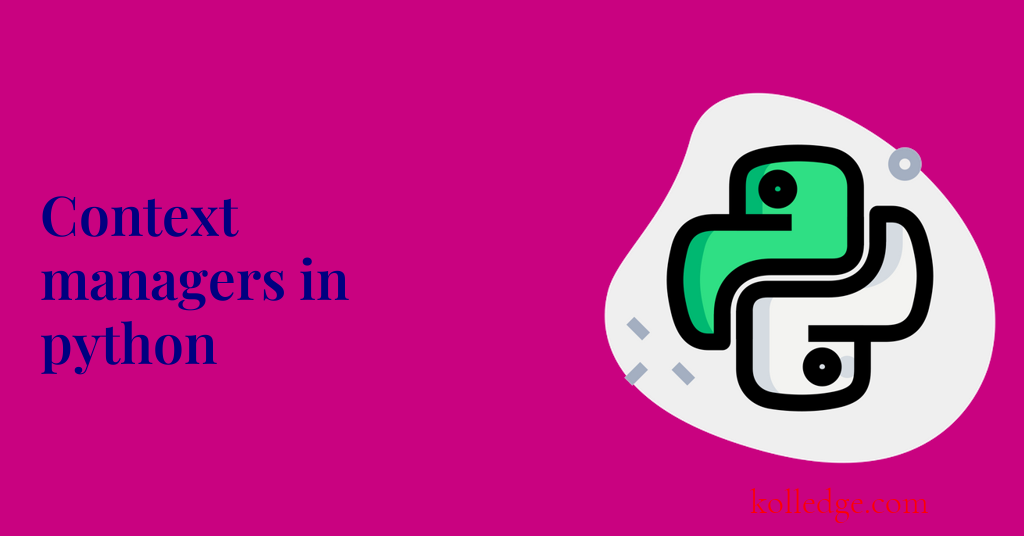
Table of Contents :
- Python Context managers
- 'with' statement in Python
- Context manager protocol
- Python context manager applications
- Implementing context manager protocol in Python
- Implementing the start and stop pattern using Python context manager
Python Context managers :
- Python context managers are a way to manage resources, such as files or network connections,
- context managers ensure resources are properly initialized and released.
- They provide a way to define setup and cleanup actions that should be executed before and after the execution of a block of code.
- Context managers are used with the
with
statement in Python.
'with' statement in Python :
- The with statement in Python is used to define a context in which a resource can be used.
- It automatically handles setup and cleanup actions before and after the block of code in the context is executed.
- The with statement is used with context manager objects.
Context manager protocol :
- The context manager protocol in Python consists of two methods:
- __enter__() and
- __exit__().
- The __enter__() method is called at the beginning of the block of code in the context, and is used to set up the resource being managed.
- The __exit__() method is called at the end of the block of code, and is used to clean up the resource being managed.
Python context manager applications :
- Context managers are commonly used for managing resources that require explicit initialization and cleanup, such as files, network connections, and threads.
- Some common examples include:
- Open – Close: Managing files that need to be opened and closed
- Lock – Release: Locking a resource before using it, and releasing it after use
- Start – Stop: Starting a service or application, and stopping it when done
- Change – Reset: Temporarily changing a configuration or state, and resetting it when done
Implementing context manager protocol in Python :
- The Python
contextlib
module provides a range of utilities for working with context managers. - A simple way to implement the context manager protocol is to use the
contextlib.contextmanager
decorator. - Code Sample :
from contextlib import contextmanager
@contextmanager
def my_context():
# Setup code
yield resource
# Cleanup code
Implementing the start and stop pattern using Python context manager :
- In Python, the start and stop pattern can be implemented using context managers.
- This can be useful for managing resources such as threads or network connections.
- Code Sample :
import threading
class MyThread(threading.Thread):
def __init__(self):
super().__init__()
def run(self):
# Start thread
pass
def stop(self):
# Stop thread
pass
with MyThread() as t:
# Thread is started
# Do something
# Thread is stopped
Prev. Tutorial : Named Tuples
Next Tutorial : Python for mysql