Introduction
In Python programming, sets are a powerful and versatile data type that allows developers to store collections of unique elements. Sets are unordered, iterable and mutable, which means they can be modified after creation. They are useful for a range of common programming tasks, such as removing duplicate items from a list, testing set membership and performing basic set operations like union and intersection. This Python tutorial on sets data type will guide you through the basics of sets, including how to create and manipulate sets, perform operations on sets and work with set methods. By the end of this tutorial, you will have a solid foundation in the sets data type in Python programming language.
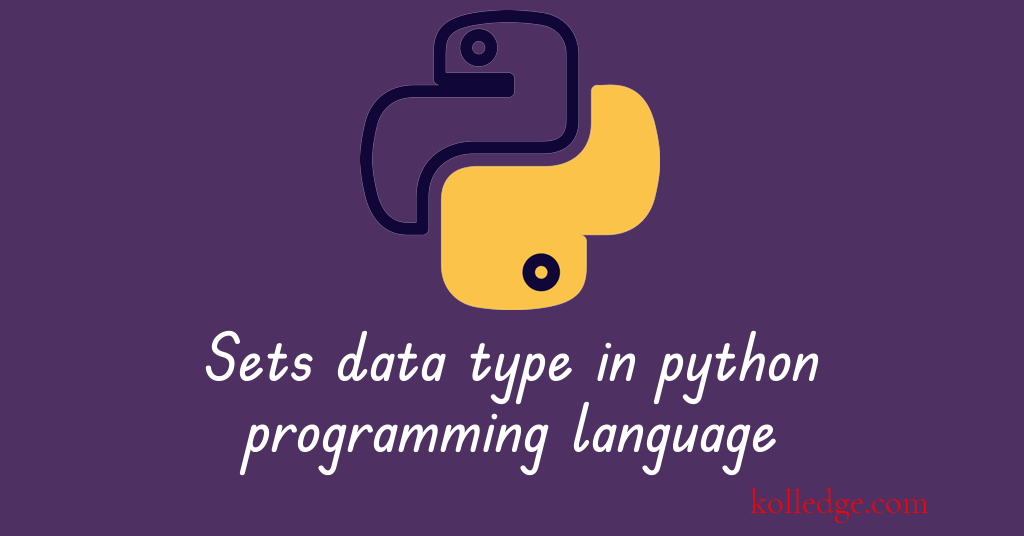
Table of Contents :
- What are Sets in Python
- Creating Sets in Python
- Different Set operations
- Frozenset data type in Python
Note # : This is just an introductory tutorial on the topic - Sets Data type in Python. We have a full section on Sets in Python in the intermediate section of this course. That section consists of a number of tutorials that cover this topic in full detail, explaining various set operations as well. After going through this tutorial if you feel like studying python sets in detail, you'd like to go through our Sets in Python section.
What are Sets in Python
- Set in Python is an unordered collection of unique elements.
- In other words Sets cannot have duplicate elements in Python.
- Set data type is used to represent a collection of unique elements as a single object.
- The Set data type in Python is implemented using the
set
class. - A simple use case of using the set data type is storing Ids of all employees.
- Set is a mutable data type.
- Set can have elements of all data types.
- Sets are unordered so indexing cannot be performed on a Set
Creating Sets in Python
- A set can be created in two ways in Python
- Enclosing elements within the curly brackets {}.
- Creating instance of set() class.
- Here are some examples of creating sets:
# Creating a set using curly braces {}
s1 = {1, 2, 3}
# Creating an empty set
s2 = set()
# Creating a set using the set() function
s3 = set([1, 2, 3])
# Creating a set from a list
s4 = set([1, 2, 3, 1, 2, 3])
# Creating a set from a tuple
s5 = set((1, 2, 3))
# Creating a set from a string
s6 = set("abc")
# Creating a set from a dictionary
s7 = set({"a": 1, "b": 2, "c": 3})
print("s1 = ", s1)
print("s2 = ", s2)
print("s3 = ", s3)
print("s4 = ", s4)
print("s5 = ", s5)
print("s6 = ", s6)
print("s7 = ", s7)
# Output
# s1 = {1, 2, 3}
# s2 = set()
# s3 = {1, 2, 3}
# s4 = {1, 2, 3}
# s5 = {1, 2, 3}
# s6 = {'a', 'b', 'c'}
# s7 = {'a', 'b', 'c'}
Different Set operations
- We can access the values in a set using a for loop
s1 = {1, 2, 3}
for value in s1:
print(value)
- We can check if a value is in a set using the in keyword
s1 = {1, 2, 3}
if 1 in s1:
print("1 is in s1")
- We can add values to a set using the add() method
s1 = {1, 2, 3}
s1.add(4)
- We can remove values from a set using the remove() method
s1 = {1, 2, 3}
s1.remove(2)
- We can find the length of a set using the len() function
s1 = {1, 2, 3}
print(len(s1))
- We can clear a set using the clear() method
s1 = {1, 2, 3}
s1.clear()
Frozenset data type in Python
- The frozenset data type creates an immutable Set.
- Once created, a frozenset cant be changed.
- The frozenset data type is implemented using a
frozenset
class in Python. - Code Sample :
fs = frozenset([1, 2, 3])
print(fs)
print(f"Data Type of fs is {type(fs)}")
# Output
# frozenset({1, 2, 3})
# Data Type of fs is <class 'frozenset'>
Prev. Tutorial : Dictionary data type
Next Tutorial : Bytes and ByteArray Data type