Beginner Level
Intermediate Level
Advanced Level
Introduction
One important aspect of programming is working with dates and time. Python provides powerful tools to work with dates and time, including the datetime module. In this tutorial, we will focus on one of the most useful methods of the datetime module, which is the strftime()
method. This method allows us to format dates and times in a specific way according to our needs.
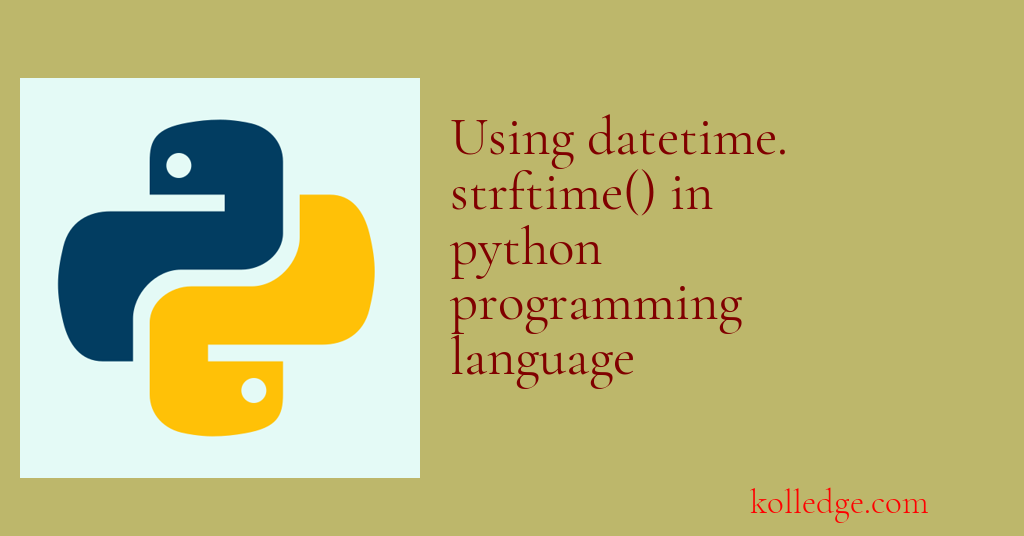
Table of Contents :
- How to convert datetime to string using strftime()
- How strftime() Works
- Format Code List
- How to create string from a Timestamp
- Locale's Appropriate Date and Time
How to convert datetime to string using strftime()
- Python's
datetime.strftime()
function formats adatetime
object into a string. - The string can be of a specific format or a generic one.
- strftime arguments :
- The first argument is the
datetime
object. - The second argument is the string format.
- The first argument is the
- Some examples:
import datetime
dt = datetime.datetime(2018, 1, 1)
d1 = dt.strftime('%A, %B %d')
print(d1)
d1 = dt.strftime('%c')
print(d1)
d1 = dt.strftime('%x')
print(d1)
d1 = dt.strftime('%X')
print(d1)
# Output
# Monday, January 01
# Mon Jan 1 00:00:00 2018
# 01/01/18
# 00:00:00
How strftime() works
strftime()
takes a format string as an argument,- The format string specifies the format of the output string.
- The format string can contain format codes that are replaced with the corresponding values from the
datetime
object. - Code Sample :
import datetime
# Create a datetime object
dt = datetime.datetime(2019, 9, 12, 10, 30, 0)
# Format the datetime object
formatted_str = dt.strftime("%Y-%m-%d %H:%M:%S")
# Print the formatted string
print(formatted_str)
# Output
# 2019-09-12 10:30:00
Format Code List in tabular form
- The format string can contain any of the format codes listed below.
Code | Meaning |
---|---|
%a | Weekday, abbreviated (Sun, Mon, Tue, etc.) |
%A | Weekday, full (Sunday, Monday, Tuesday, etc.) |
%w | Weekday as a decimal number, where 0 is Sunday and 6 is Saturday |
%d | Day of the month, zero-padded (01, 02, ..., 31) |
%b | Month name, abbreviated (Jan, Feb, Mar, etc.) |
%B | Month name, full (January, February, March, etc.) |
%m | Month as a decimal number, zero-padded (01, 02, ..., 12) |
%y | Year without century, zero-padded (00, 01, ..., 99) |
%Y | Year with century, as a decimal number |
%H | Hour in 24-hour format, zero-padded (00, 01, ..., 23) |
%I | Hour in 12-hour format, zero-padded (01, 02, ..., 12) |
%p | AM/PM |
%M | Minute, zero-padded (00, 01, ..., 59) |
%S | Second, zero-padded (00, 01, ..., 59) |
%f | Microsecond, zero-padded (000000, 000001, ..., 999999) |
%z | UTC offset in the form +HHMM or -HHMM |
%Z | time zone name %j - day of the year as a zero-padded decimal number (001-366) |
%U | week number of the year (Sunday as the first day of the week) as a zero padded decimal number (00-53) |
%W | week number of the year (Monday as the first day of the week) as a decimal number (00-53) |
%c | locale's appropriate date and time representation |
%x | locale's appropriate date representation |
%X | locale's appropriate time representation |
%% | a literal '%' character |
How to create string from a Timestamp :
- Timestamps can be converted to
datetime
objects using thedatetime.fromtimestamp()
method. - These
datetime
objects can then be converted to strings usingstrftime()
. - Code Sample :
from datetime import datetime
timestamp = 1646153014.449067
dt_object = datetime.fromtimestamp(timestamp)
datetime_string = dt_object.strftime("%d-%b-%Y %H:%M:%S")
print("Datetime string:", datetime_string)
# Output
# Datetime string: 01-Mar-2022 22:13:34
Locale's Appropriate Date and Time :
- The
strftime()
method can also format dates and times according to the current locale using the%c
code. - The
locale
module can be used to set the system's locale. - Code Sample :
import datetime
import locale
# Set the locale
locale.setlocale(locale.LC_ALL, 'en_US')
# Create a datetime object
dt = datetime.datetime(2021, 9, 22, 10, 30, 0)
# Format the datetime object
formatted_str = dt.strftime("%c")
# Print the formatted string
print("Formatted String : ",formatted_str)
# Output
# Formatted String : 9/22/2021 10:30:00 AM
Prev. Tutorial : Datetime module
Next Tutorial : Using datetime.strptime()