Beginner Level
Intermediate Level
Advanced Level
Introduction
Functions are one of the fundamental building blocks of programming, allowing us to encapsulate sets of instructions and execute them repeatedly. When writing functions, it's often necessary to provide input data to the function for it to carry out its task. These inputs are known as arguments, and in Python, we have several ways of passing them to our functions. Whether you're new to Python or looking to improve your knowledge of function arguments, this tutorial will cover the basics, including positional arguments, keyword arguments, default values, and variable-length arguments. Let's get started!
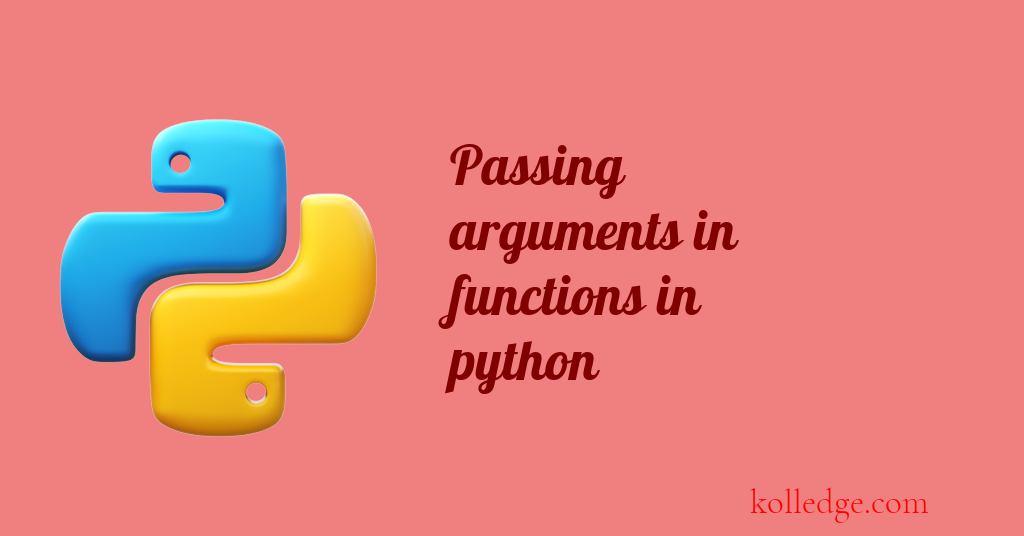
Table of Contents :
- Passing values to Python functions
- Parameters vs. Arguments
- Returning a value
- Python functions with multiple parameters
- Types of Arguments in Python
- Positional arguments
- Keyword arguments
Passing values to Python functions
- Sometimes function body needs some data that is known only to the calling portion of our code.
- We need to make this data available to the function by passing this data to the function.
- To pass data to the function we enclose this data within the parentheses of function call.
- The data that we pass are called arguments.
- The function definition should also specify that it will receive some data.
- This is done by adding parameters within parentheses to function definition.
- These parameters of function definition can be used as variables within the function body.
- These parameters are local to the function i.e. valid only within the function body.
Parameters vs. Arguments
- Often people use the terms parameters and arguments interchangeably.
- But in practice parameters and arguments of a function are different.
- A parameter is the data needed in function body.
- Parameters are specified within parentheses at the time of function definition.
- An argument is the data that we pass to the function while calling the function.
- The arguments are mentioned within the parentheses at the time of function call.
- Code Sample : In the code below
- a, b are the parameters
- x, y are the arguments
# Defining function calculate
def calculate(a, b):
res = a + b
return res
x = 2
y = 4
# calling the function calculate
s = calculate(x, y)
print("The sum of the two numbers is : ", s)
Returning a value
- A function may or may not return a value.
- Sometimes our logic requires the function to return a value after completing the desired task.
- A return statement is used to return a value.
- In the code sample shown above the function calculate returns res after calculations.
- The value returned by the function is received by the calling code.
Python functions with multiple parameters
- A function can have any number of parameters - zero, one, or more than one parameters.
- At the time of calling the function, we need to pass the arguments that match the number of parameter in function definition.
- Python interpreter will generate an error if we pass more or fewer arguments.
- This might work a bit differently in case of default parameters.
Types of Arguments in Python
- There are two types of arguments that Python functions can take:
- Positional arguments
- Keyword arguments
Positional arguments in Python
- Positional arguments are the arguments that are passed in the order that they are defined in the function.
- In case of positional arguments, the order in which we pass the arguments is very important.
- Example of Positional arguments :
- let's say we have a function that takes two arguments, an integer and a string.
- If we call the function as shown below :
- Then Python interpreter will match -
- the first argument
10
with the first parameternum
, - the second argument
“Hello”
with the second parameterstring
.
- the first argument
def example(num, string):
print(num)
print(string)
example(10, "Hello")
# Output
# 10
# Hello World
Keyword arguments in Python
- Keyword arguments are arguments that are passed using the name of the parameter.
- In case of keyword arguments, the order in which we pass the arguments is not important.
- Example of Keyword arguments :
- let's say we have a function that takes two arguments, an integer and a string.
- If we call the function as shown below :
- Then Python will match -
- the keyword argument
num
with the parameternum
, - and the keyword argument
string
with the parameterstring
.
- the keyword argument
- Even though we have passed the arguments in reverse order
- The output is correct as interpreter matches the names of the arguments.
def example(num, string):
print(num)
print(string)
example(string="Hello World", num=10)
# Output
# 10
# Hello World
Prev. Tutorial : Functions in Python
Next Tutorial : Default parameters